350. Intersection of Two Arrays II LeetCode Solution
In this guide, you will get 350. Intersection of Two Arrays II LeetCode Solution with the best time and space complexity. The solution to Intersection of Two Arrays II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Intersection of Two Arrays II solution in C++
- Intersection of Two Arrays II solution in Java
- Intersection of Two Arrays II solution in Python
- Additional Resources
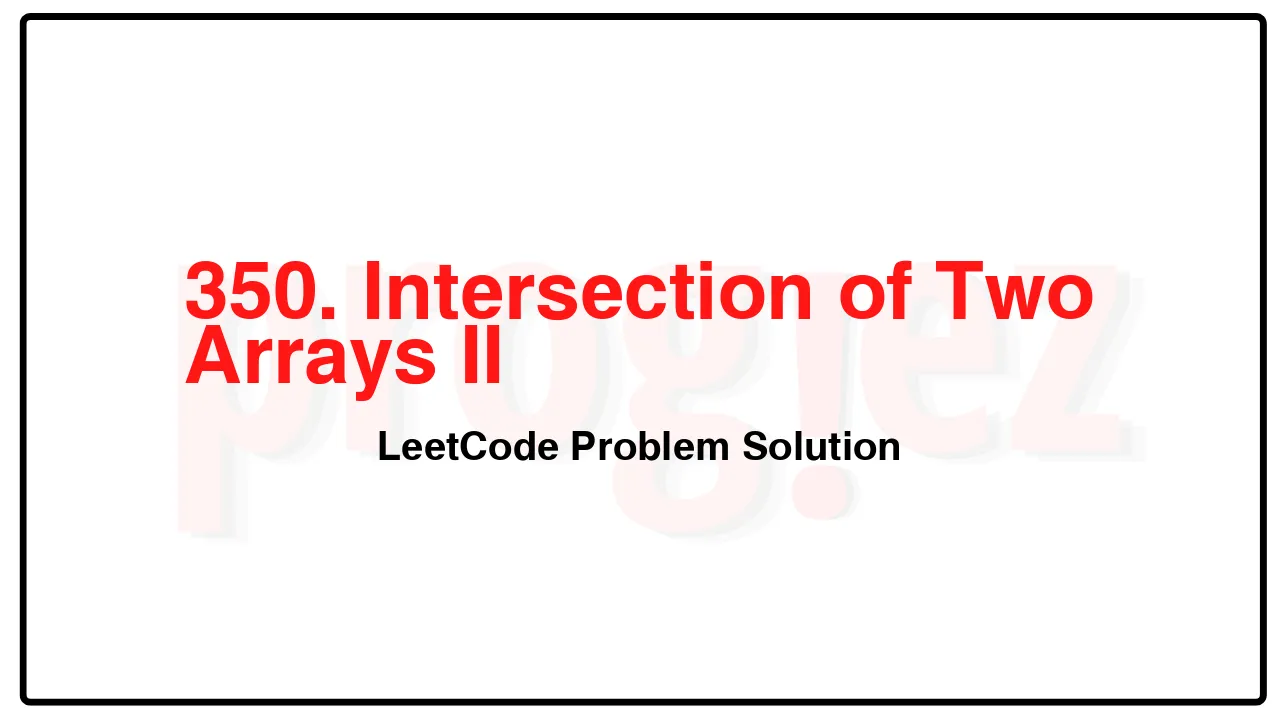
Problem Statement of Intersection of Two Arrays II
Given two integer arrays nums1 and nums2, return an array of their intersection. Each element in the result must appear as many times as it shows in both arrays and you may return the result in any order.
Example 1:
Input: nums1 = [1,2,2,1], nums2 = [2,2]
Output: [2,2]
Example 2:
Input: nums1 = [4,9,5], nums2 = [9,4,9,8,4]
Output: [4,9]
Explanation: [9,4] is also accepted.
Constraints:
1 <= nums1.length, nums2.length <= 1000
0 <= nums1[i], nums2[i] <= 1000
Follow up:
What if the given array is already sorted? How would you optimize your algorithm?
What if nums1's size is small compared to nums2's size? Which algorithm is better?
What if elements of nums2 are stored on disk, and the memory is limited such that you cannot load all elements into the memory at once?
Complexity Analysis
- Time Complexity: O(m + n)
- Space Complexity: O(\min(m, n))
350. Intersection of Two Arrays II LeetCode Solution in C++
class Solution {
public:
vector<int> intersect(vector<int>& nums1, vector<int>& nums2) {
if (nums1.size() > nums2.size())
return intersect(nums2, nums1);
vector<int> ans;
unordered_map<int, int> count;
for (const int num : nums1)
++count[num];
for (const int num : nums2)
if (const auto it = count.find(num);
it != count.cend() && it->second-- > 0)
ans.push_back(num);
return ans;
}
};
/* code provided by PROGIEZ */
350. Intersection of Two Arrays II LeetCode Solution in Java
class Solution {
public int[] intersect(int[] nums1, int[] nums2) {
if (nums1.length > nums2.length)
return intersect(nums2, nums1);
List<Integer> ans = new ArrayList<>();
Map<Integer, Integer> count = new HashMap<>();
for (final int num : nums1)
count.put(num, count.getOrDefault(num, 0) + 1);
for (final int num : nums2)
if (count.containsKey(num) && count.get(num) > 0) {
ans.add(num);
count.put(num, count.get(num) - 1);
}
return ans.stream().mapToInt(Integer::intValue).toArray();
}
}
// code provided by PROGIEZ
350. Intersection of Two Arrays II LeetCode Solution in Python
class Solution:
def intersect(self, nums1: list[int], nums2: list[int]) -> list[int]:
if len(nums1) > len(nums2):
return self.intersect(nums2, nums1)
ans = []
count = collections.Counter(nums1)
for num in nums2:
if count[num] > 0:
ans.append(num)
count[num] -= 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.