343. Integer Break LeetCode Solution
In this guide, you will get 343. Integer Break LeetCode Solution with the best time and space complexity. The solution to Integer Break problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Integer Break solution in C++
- Integer Break solution in Java
- Integer Break solution in Python
- Additional Resources
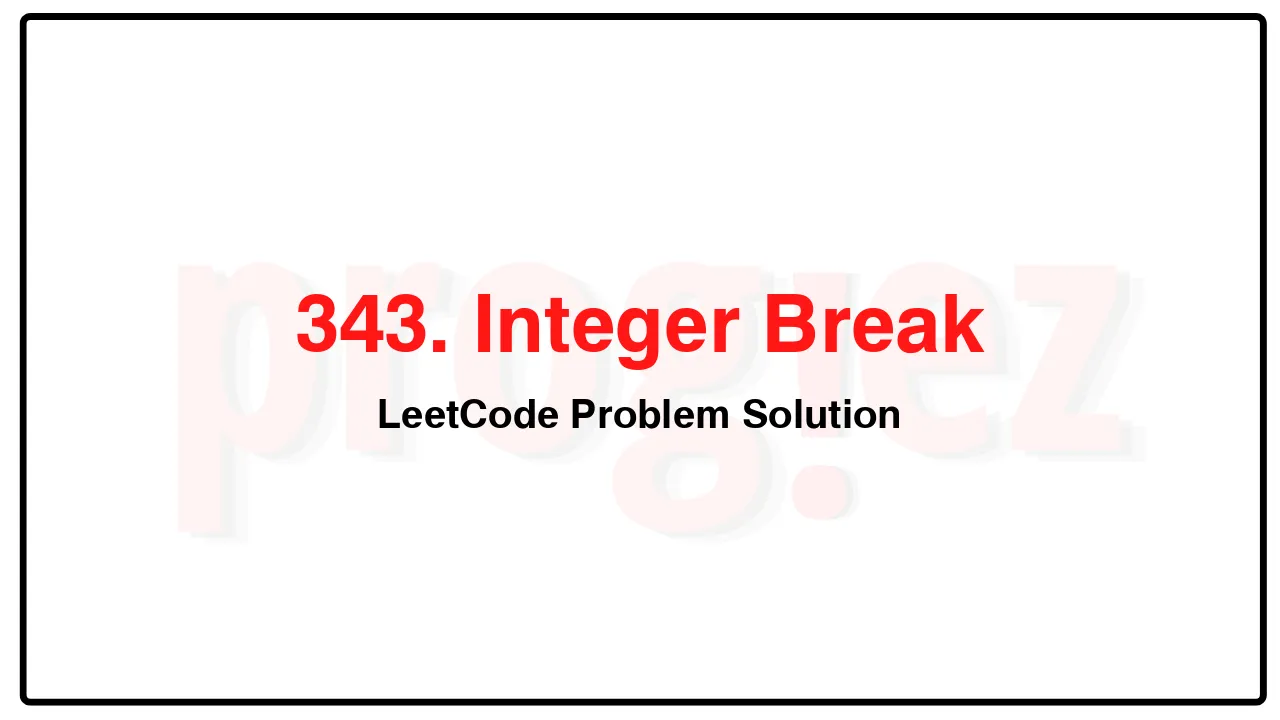
Problem Statement of Integer Break
Given an integer n, break it into the sum of k positive integers, where k >= 2, and maximize the product of those integers.
Return the maximum product you can get.
Example 1:
Input: n = 2
Output: 1
Explanation: 2 = 1 + 1, 1 × 1 = 1.
Example 2:
Input: n = 10
Output: 36
Explanation: 10 = 3 + 3 + 4, 3 × 3 × 4 = 36.
Constraints:
2 <= n <= 58
Complexity Analysis
- Time Complexity: O(n / 3)
- Space Complexity: O(1)
343. Integer Break LeetCode Solution in C++
class Solution {
public:
int integerBreak(int n) {
// If an optimal product contains a factor f >= 4, then we can replace it
// with 2 and f - 2 without losing optimality. As 2(f - 2) = 2f - 4 >= f,
// we never need a factor >= 4, meaning we only need factors 1, 2, and 3
// (and 1 is wasteful).
// Also, 3 * 3 is better than 2 * 2 * 2, so we never use 2 more than twice.
if (n == 2) // 1 * 1
return 1;
if (n == 3) // 1 * 2
return 2;
int ans = 1;
while (n > 4) {
n -= 3;
ans *= 3;
}
ans *= n;
return ans;
}
};
/* code provided by PROGIEZ */
343. Integer Break LeetCode Solution in Java
class Solution {
public int integerBreak(int n) {
// If an optimal product contains a factor f >= 4, then we can replace it
// with 2 and f - 2 without losing optimality. As 2(f - 2) = 2f - 4 >= f,
// we never need a factor >= 4, meaning we only need factors 1, 2, and 3
// (and 1 is wasteful).
// Also, 3 * 3 is better than 2 * 2 * 2, so we never use 2 more than twice.
if (n == 2)
return 1; // 1 * 1
if (n == 3)
return 2; // 1 * 2
int ans = 1;
while (n > 4) {
n -= 3;
ans *= 3;
}
ans *= n;
return ans;
}
}
// code provided by PROGIEZ
343. Integer Break LeetCode Solution in Python
class Solution:
def integerBreak(self, n: int) -> int:
# If an optimal product contains a factor f >= 4, then we can replace it
# with 2 and f - 2 without losing optimality. As 2(f - 2) = 2f - 4 >= f,
# we never need a factor >= 4, meaning we only need factors 1, 2, and 3
# (and 1 is wasteful).
# Also, 3 * 3 is better than 2 * 2 * 2, so we never use 2 more than twice.
if n == 2: # 1 * 1
return 1
if n == 3: # 1 * 2
return 2
ans = 1
while n > 4:
n -= 3
ans *= 3
ans *= n
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.