3024. Type of Triangle LeetCode Solution
In this guide, you will get 3024. Type of Triangle LeetCode Solution with the best time and space complexity. The solution to Type of Triangle problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Type of Triangle solution in C++
- Type of Triangle solution in Java
- Type of Triangle solution in Python
- Additional Resources
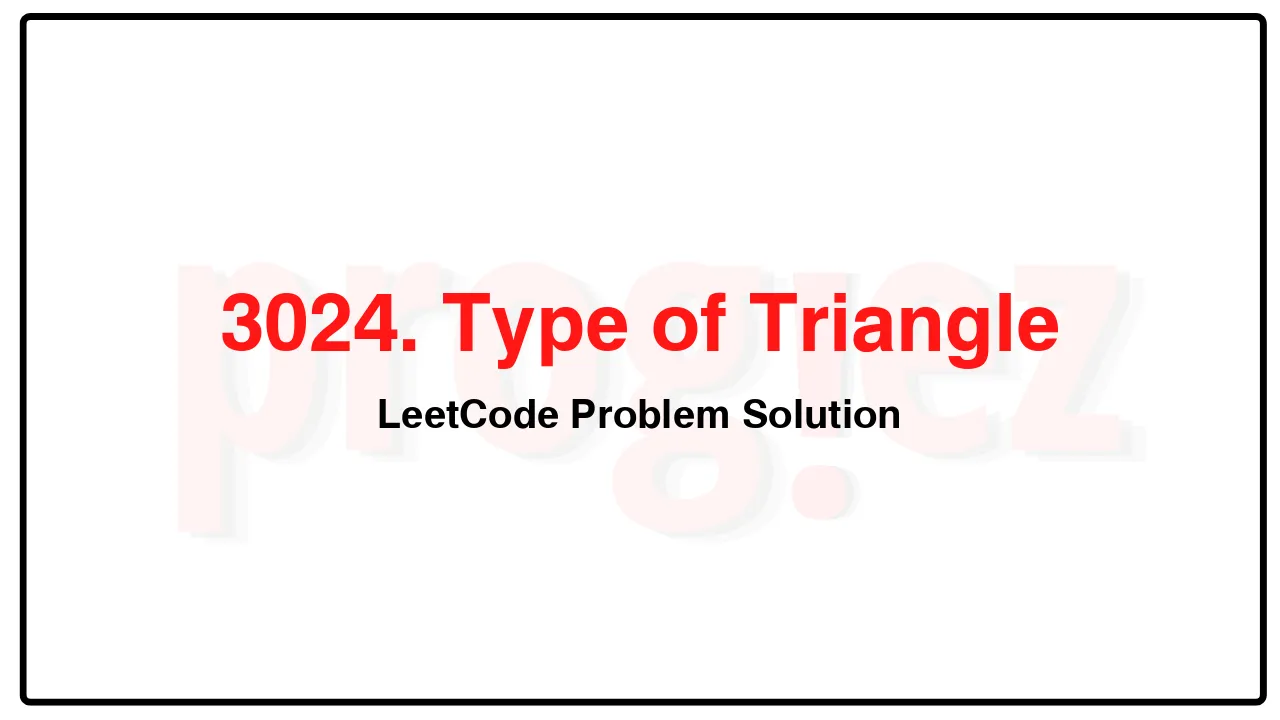
Problem Statement of Type of Triangle
You are given a 0-indexed integer array nums of size 3 which can form the sides of a triangle.
A triangle is called equilateral if it has all sides of equal length.
A triangle is called isosceles if it has exactly two sides of equal length.
A triangle is called scalene if all its sides are of different lengths.
Return a string representing the type of triangle that can be formed or “none” if it cannot form a triangle.
Example 1:
Input: nums = [3,3,3]
Output: “equilateral”
Explanation: Since all the sides are of equal length, therefore, it will form an equilateral triangle.
Example 2:
Input: nums = [3,4,5]
Output: “scalene”
Explanation:
nums[0] + nums[1] = 3 + 4 = 7, which is greater than nums[2] = 5.
nums[0] + nums[2] = 3 + 5 = 8, which is greater than nums[1] = 4.
nums[1] + nums[2] = 4 + 5 = 9, which is greater than nums[0] = 3.
Since the sum of the two sides is greater than the third side for all three cases, therefore, it can form a triangle.
As all the sides are of different lengths, it will form a scalene triangle.
Constraints:
nums.length == 3
1 <= nums[i] <= 100
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
3024. Type of Triangle LeetCode Solution in C++
class Solution {
public:
string triangleType(vector<int>& nums) {
ranges::sort(nums);
if (nums[0] + nums[1] <= nums[2])
return "none";
if (nums[0] == nums[1] && nums[1] == nums[2])
return "equilateral";
if (nums[0] == nums[1] || nums[1] == nums[2])
return "isosceles";
return "scalene";
}
};
/* code provided by PROGIEZ */
3024. Type of Triangle LeetCode Solution in Java
class Solution {
public String triangleType(int[] nums) {
Arrays.sort(nums);
if (nums[0] + nums[1] <= nums[2])
return "none";
if (nums[0] == nums[1] && nums[1] == nums[2])
return "equilateral";
if (nums[0] == nums[1] || nums[1] == nums[2])
return "isosceles";
return "scalene";
}
}
// code provided by PROGIEZ
3024. Type of Triangle LeetCode Solution in Python
class Solution:
def triangleType(self, nums: list[int]) -> str:
nums.sort()
if nums[0] + nums[1] <= nums[2]:
return 'none'
if nums[0] == nums[1] and nums[1] == nums[2]:
return 'equilateral'
if nums[0] == nums[1] or nums[1] == nums[2]:
return 'isosceles'
return 'scalene'
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.