119. Pascal’s Triangle II LeetCode Solution
In this guide, you will get 119. Pascal’s Triangle II LeetCode Solution with the best time and space complexity. The solution to Pascal’s Triangle II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Pascal’s Triangle II solution in C++
- Pascal’s Triangle II solution in Java
- Pascal’s Triangle II solution in Python
- Additional Resources
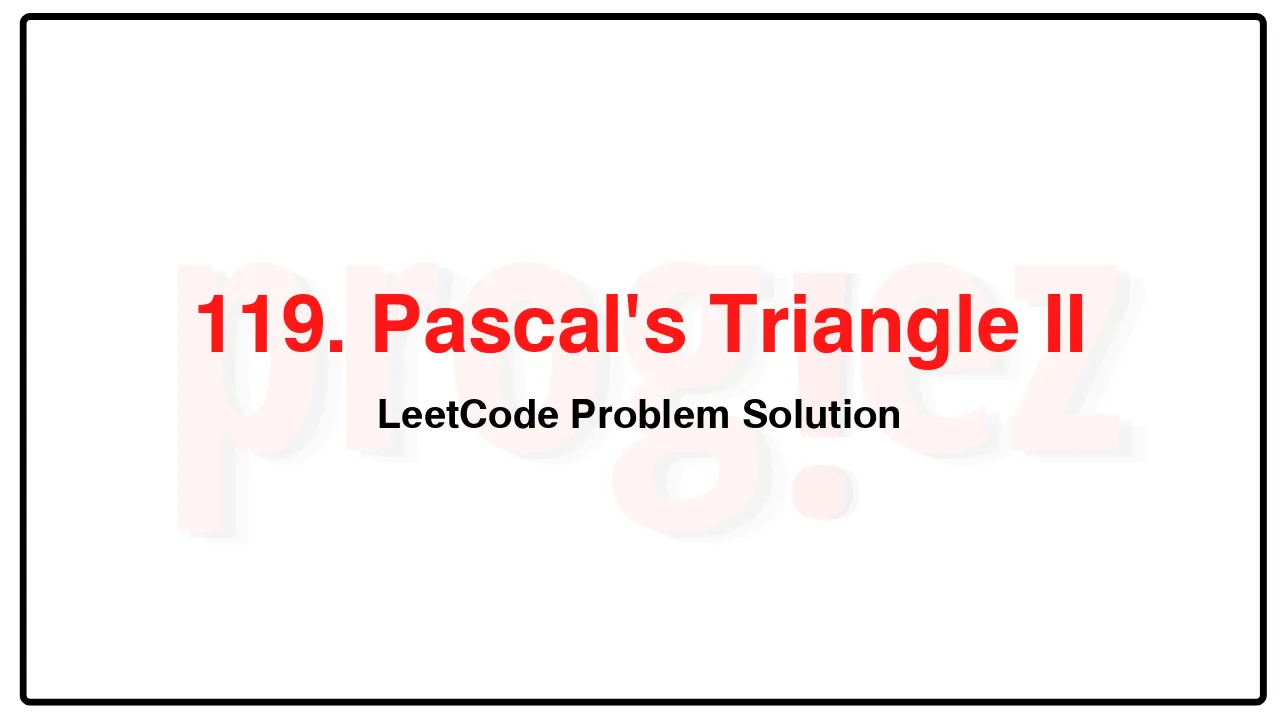
Problem Statement of Pascal’s Triangle II
Given an integer rowIndex, return the rowIndexth (0-indexed) row of the Pascal’s triangle.
In Pascal’s triangle, each number is the sum of the two numbers directly above it as shown:
Example 1:
Input: rowIndex = 3
Output: [1,3,3,1]
Example 2:
Input: rowIndex = 0
Output: [1]
Example 3:
Input: rowIndex = 1
Output: [1,1]
Constraints:
0 <= rowIndex <= 33
Follow up: Could you optimize your algorithm to use only O(rowIndex) extra space?
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(n)
119. Pascal’s Triangle II LeetCode Solution in C++
class Solution {
public:
vector<int> getRow(int rowIndex) {
vector<int> ans(rowIndex + 1, 1);
for (int i = 2; i < rowIndex + 1; ++i)
for (int j = 1; j < i; ++j)
ans[i - j] += ans[i - j - 1];
return ans;
}
};
/* code provided by PROGIEZ */
119. Pascal’s Triangle II LeetCode Solution in Java
class Solution {
public List<Integer> getRow(int rowIndex) {
Integer[] ans = new Integer[rowIndex + 1];
Arrays.fill(ans, 1);
for (int i = 2; i < rowIndex + 1; ++i)
for (int j = 1; j < i; ++j)
ans[i - j] += ans[i - j - 1];
return Arrays.asList(ans);
}
}
// code provided by PROGIEZ
119. Pascal’s Triangle II LeetCode Solution in Python
class Solution:
def getRow(self, rowIndex: int) -> list[int]:
ans = [1] * (rowIndex + 1)
for i in range(2, rowIndex + 1):
for j in range(1, i):
ans[i - j] += ans[i - j - 1]
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.