2843. Count Symmetric Integers LeetCode Solution
In this guide, you will get 2843. Count Symmetric Integers LeetCode Solution with the best time and space complexity. The solution to Count Symmetric Integers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Count Symmetric Integers solution in C++
- Count Symmetric Integers solution in Java
- Count Symmetric Integers solution in Python
- Additional Resources
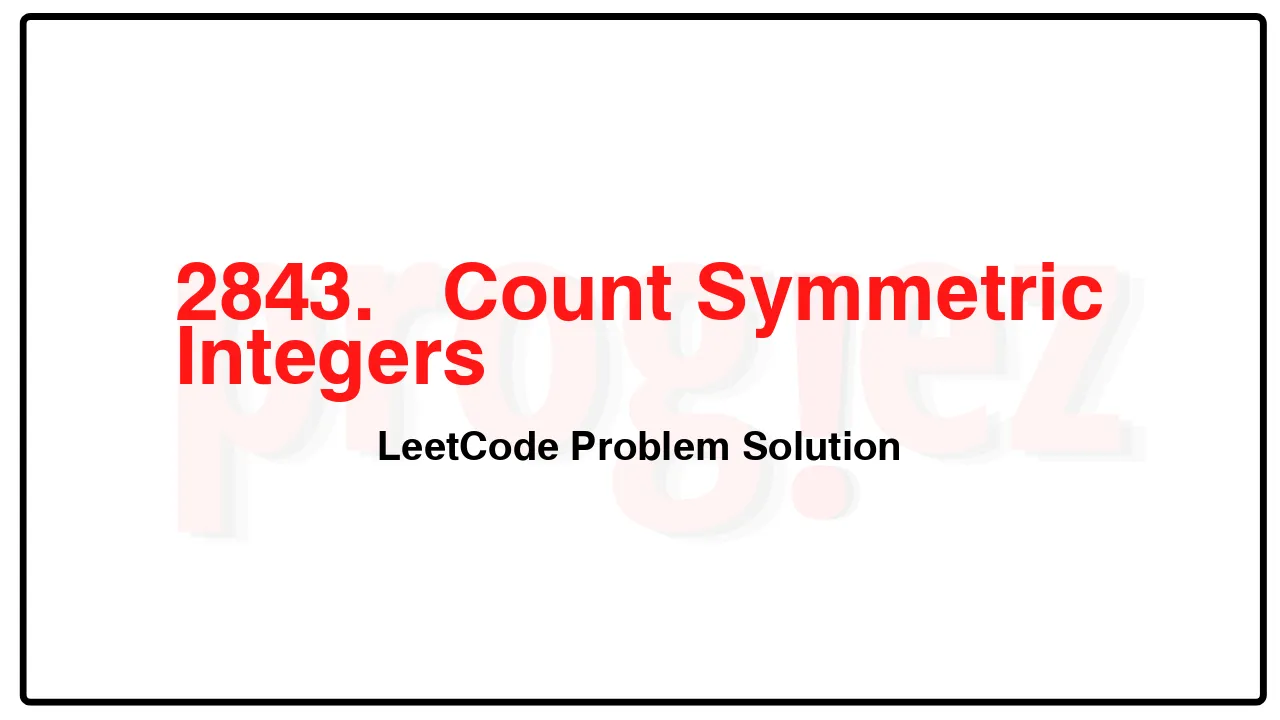
Problem Statement of Count Symmetric Integers
You are given two positive integers low and high.
An integer x consisting of 2 * n digits is symmetric if the sum of the first n digits of x is equal to the sum of the last n digits of x. Numbers with an odd number of digits are never symmetric.
Return the number of symmetric integers in the range [low, high].
Example 1:
Input: low = 1, high = 100
Output: 9
Explanation: There are 9 symmetric integers between 1 and 100: 11, 22, 33, 44, 55, 66, 77, 88, and 99.
Example 2:
Input: low = 1200, high = 1230
Output: 4
Explanation: There are 4 symmetric integers between 1200 and 1230: 1203, 1212, 1221, and 1230.
Constraints:
1 <= low <= high <= 104
Complexity Analysis
- Time Complexity: O(\texttt{high})
- Space Complexity: O(1)
2843. Count Symmetric Integers LeetCode Solution in C++
class Solution {
public:
int countSymmetricIntegers(int low, int high) {
int ans = 0;
for (int num = low; num <= high; ++num)
if (isSymmetricInteger(num))
++ans;
return ans;
}
private:
bool isSymmetricInteger(int num) {
if (num >= 10 && num <= 99)
return num / 10 == num % 10;
if (num >= 1000 && num <= 9999) {
const int left = num / 100;
const int right = num % 100;
return left / 10 + left % 10 == right / 10 + right % 10;
}
return false;
}
};
/* code provided by PROGIEZ */
2843. Count Symmetric Integers LeetCode Solution in Java
class Solution {
public int countSymmetricIntegers(int low, int high) {
int ans = 0;
for (int num = low; num <= high; ++num)
if (isSymmetricInteger(num))
++ans;
return ans;
}
private boolean isSymmetricInteger(int num) {
if (num >= 10 && num <= 99)
return num / 10 == num % 10;
if (num >= 1000 && num <= 9999) {
final int left = num / 100;
final int right = num % 100;
return left / 10 + left % 10 == right / 10 + right % 10;
}
return false;
}
}
// code provided by PROGIEZ
2843. Count Symmetric Integers LeetCode Solution in Python
class Solution:
def countSymmetricIntegers(self, low: int, high: int) -> int:
def isSymmetricInteger(num: int) -> bool:
if num >= 10 and num <= 99:
return num // 10 == num % 10
if num >= 1000 and num <= 9999:
left = num // 100
right = num % 100
return left // 10 + left % 10 == right // 10 + right % 10
return False
return sum(isSymmetricInteger(num) for num in range(low, high + 1))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.