268. Missing Number LeetCode Solution
In this guide, you will get 268. Missing Number LeetCode Solution with the best time and space complexity. The solution to Missing Number problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Missing Number solution in C++
- Missing Number solution in Java
- Missing Number solution in Python
- Additional Resources
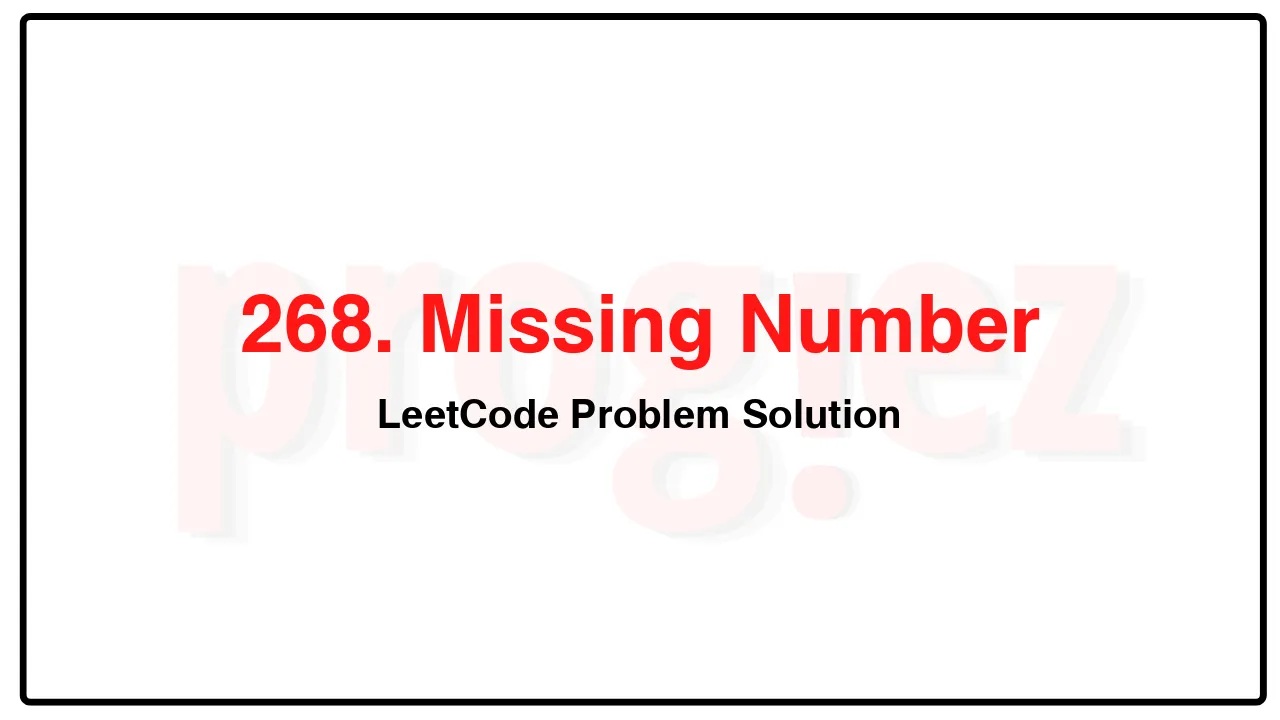
Problem Statement of Missing Number
Given an array nums containing n distinct numbers in the range [0, n], return the only number in the range that is missing from the array.
Example 1:
Input: nums = [3,0,1]
Output: 2
Explanation:
n = 3 since there are 3 numbers, so all numbers are in the range [0,3]. 2 is the missing number in the range since it does not appear in nums.
Example 2:
Input: nums = [0,1]
Output: 2
Explanation:
n = 2 since there are 2 numbers, so all numbers are in the range [0,2]. 2 is the missing number in the range since it does not appear in nums.
Example 3:
Input: nums = [9,6,4,2,3,5,7,0,1]
Output: 8
Explanation:
n = 9 since there are 9 numbers, so all numbers are in the range [0,9]. 8 is the missing number in the range since it does not appear in nums.
Constraints:
n == nums.length
1 <= n <= 104
0 <= nums[i] <= n
All the numbers of nums are unique.
Follow up: Could you implement a solution using only O(1) extra space complexity and O(n) runtime complexity?
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
268. Missing Number LeetCode Solution in C++
class Solution {
public:
int missingNumber(vector<int>& nums) {
int ans = nums.size();
for (int i = 0; i < nums.size(); ++i)
ans ^= i ^ nums[i];
return ans;
}
};
/* code provided by PROGIEZ */
268. Missing Number LeetCode Solution in Java
class Solution {
public int missingNumber(int[] nums) {
int ans = nums.length;
for (int i = 0; i < nums.length; ++i)
ans ^= i ^ nums[i];
return ans;
}
}
// code provided by PROGIEZ
268. Missing Number LeetCode Solution in Python
class Solution:
def missingNumber(self, nums: list[int]) -> int:
ans = len(nums)
for i, num in enumerate(nums):
ans ^= i ^ num
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.