2310. Sum of Numbers With Units Digit K LeetCode Solution
In this guide, you will get 2310. Sum of Numbers With Units Digit K LeetCode Solution with the best time and space complexity. The solution to Sum of Numbers With Units Digit K problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Sum of Numbers With Units Digit K solution in C++
- Sum of Numbers With Units Digit K solution in Java
- Sum of Numbers With Units Digit K solution in Python
- Additional Resources
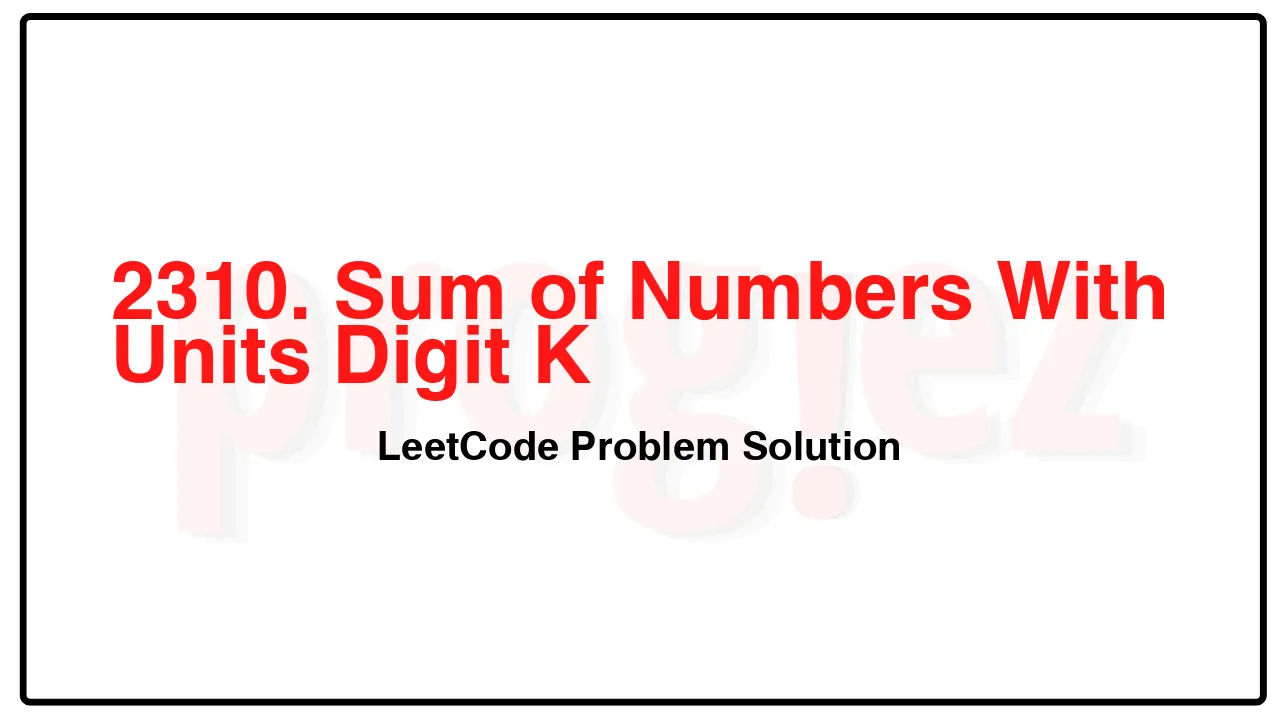
Problem Statement of Sum of Numbers With Units Digit K
Given two integers num and k, consider a set of positive integers with the following properties:
The units digit of each integer is k.
The sum of the integers is num.
Return the minimum possible size of such a set, or -1 if no such set exists.
Note:
The set can contain multiple instances of the same integer, and the sum of an empty set is considered 0.
The units digit of a number is the rightmost digit of the number.
Example 1:
Input: num = 58, k = 9
Output: 2
Explanation:
One valid set is [9,49], as the sum is 58 and each integer has a units digit of 9.
Another valid set is [19,39].
It can be shown that 2 is the minimum possible size of a valid set.
Example 2:
Input: num = 37, k = 2
Output: -1
Explanation: It is not possible to obtain a sum of 37 using only integers that have a units digit of 2.
Example 3:
Input: num = 0, k = 7
Output: 0
Explanation: The sum of an empty set is considered 0.
Constraints:
0 <= num <= 3000
0 <= k <= 9
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
2310. Sum of Numbers With Units Digit K LeetCode Solution in C++
class Solution {
public:
int minimumNumbers(int num, int k) {
if (num == 0)
return 0;
// Assume the size of the set is n, and the numbers in the set are X1, X2,
// ..., Xn. Since the units digit of each number is k, X1 + X2 + ... + Xn =
// N * k + 10 * (x1 + x2 + ... + xn) = num. Therefore, the goal is to find
// the n s.t. n * k % 10 = num % 10
for (int i = 1; i <= 10 && i * k <= num; ++i)
if (i * k % 10 == num % 10)
return i;
return -1;
}
};
/* code provided by PROGIEZ */
2310. Sum of Numbers With Units Digit K LeetCode Solution in Java
class Solution {
public int minimumNumbers(int num, int k) {
if (num == 0)
return 0;
// Assume the size of the set is n, and the numbers in the set are X1, X2,
// ..., Xn. Since the units digit of each number is k, X1 + X2 + ... + Xn =
// N * k + 10 * (x1 + x2 + ... + xn) = num. Therefore, the goal is to find
// the n s.t. n * k % 10 = num % 10
for (int i = 1; i <= 10 && i * k <= num; ++i)
if (i * k % 10 == num % 10)
return i;
return -1;
}
}
// code provided by PROGIEZ
2310. Sum of Numbers With Units Digit K LeetCode Solution in Python
class Solution:
def minimumNumbers(self, num: int, k: int) -> int:
if num == 0:
return 0
# Assume the size of the set is n, and the numbers in the set are X1, X2,
# ..., Xn. Since the units digit of each number is k, X1 + X2 + ... + Xn =
# N * k + 10 * (x1 + x2 + ... + xn) = num. Therefore, the goal is to find
# the n s.t. n * k % 10 = num % 10
for i in range(1, 11):
if i * k > num + 1:
break
if i * k % 10 == num % 10:
return i
return -1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.