2622. Cache With Time Limit LeetCode Solution
In this guide, you will get 2622. Cache With Time Limit LeetCode Solution with the best time and space complexity. The solution to Cache With Time Limit problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Cache With Time Limit solution in C++
- Cache With Time Limit solution in Java
- Cache With Time Limit solution in Python
- Additional Resources
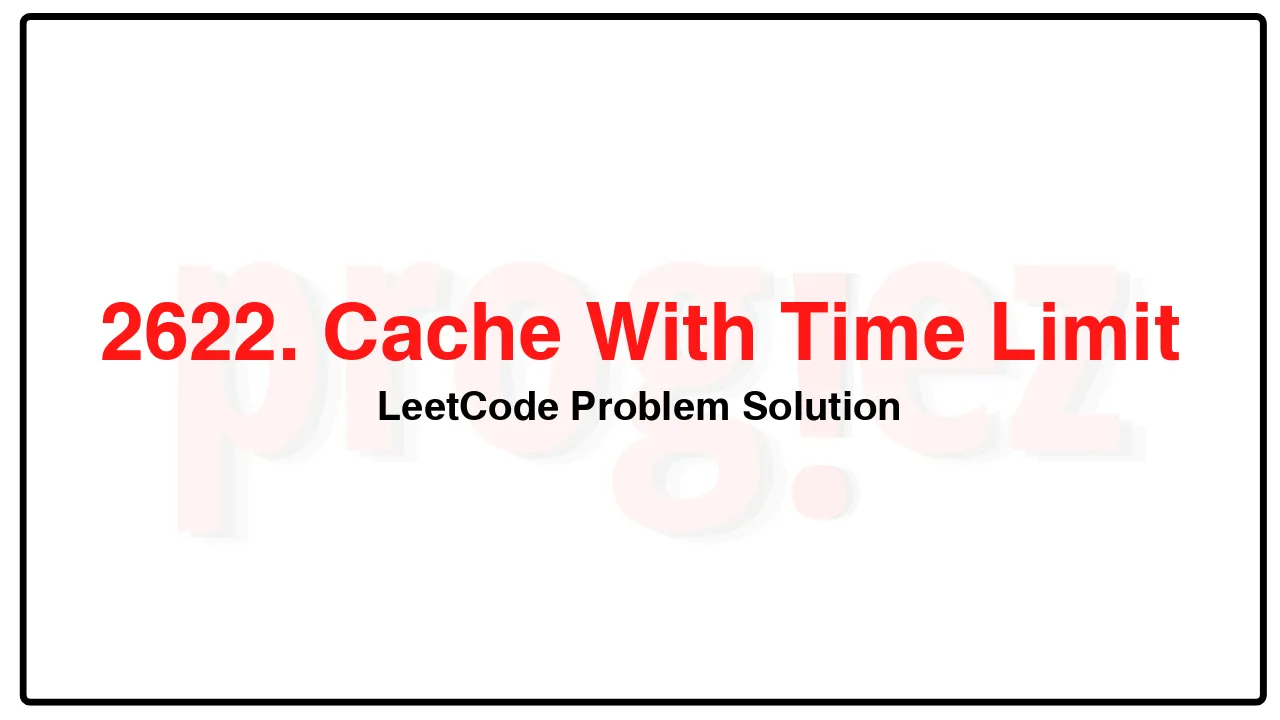
Problem Statement of Cache With Time Limit
Write a class that allows getting and setting key-value pairs, however a time until expiration is associated with each key.
The class has three public methods:
set(key, value, duration): accepts an integer key, an integer value, and a duration in milliseconds. Once the duration has elapsed, the key should be inaccessible. The method should return true if the same un-expired key already exists and false otherwise. Both the value and duration should be overwritten if the key already exists.
get(key): if an un-expired key exists, it should return the associated value. Otherwise it should return -1.
count(): returns the count of un-expired keys.
Example 1:
Input:
actions = [“TimeLimitedCache”, “set”, “get”, “count”, “get”]
values = [[], [1, 42, 100], [1], [], [1]]
timeDelays = [0, 0, 50, 50, 150]
Output: [null, false, 42, 1, -1]
Explanation:
At t=0, the cache is constructed.
At t=0, a key-value pair (1: 42) is added with a time limit of 100ms. The value doesn’t exist so false is returned.
At t=50, key=1 is requested and the value of 42 is returned.
At t=50, count() is called and there is one active key in the cache.
At t=100, key=1 expires.
At t=150, get(1) is called but -1 is returned because the cache is empty.
Example 2:
Input:
actions = [“TimeLimitedCache”, “set”, “set”, “get”, “get”, “get”, “count”]
values = [[], [1, 42, 50], [1, 50, 100], [1], [1], [1], []]
timeDelays = [0, 0, 40, 50, 120, 200, 250]
Output: [null, false, true, 50, 50, -1, 0]
Explanation:
At t=0, the cache is constructed.
At t=0, a key-value pair (1: 42) is added with a time limit of 50ms. The value doesn’t exist so false is returned.
At t=40, a key-value pair (1: 50) is added with a time limit of 100ms. A non-expired value already existed so true is returned and the old value was overwritten.
At t=50, get(1) is called which returned 50.
At t=120, get(1) is called which returned 50.
At t=140, key=1 expires.
At t=200, get(1) is called but the cache is empty so -1 is returned.
At t=250, count() returns 0 because the cache is empty.
Constraints:
0 <= key, value <= 109
0 <= duration <= 1000
1 <= actions.length <= 100
actions.length === values.length
actions.length === timeDelays.length
0 <= timeDelays[i] <= 1450
actions[i] is one of "TimeLimitedCache", "set", "get" and "count"
First action is always "TimeLimitedCache" and must be executed immediately, with a 0-millisecond delay
Complexity Analysis
- Time Complexity: Google AdSense
- Space Complexity: Google Analytics
2622. Cache With Time Limit LeetCode Solution in C++
interface Value {
value: number;
expiredAt: number;
}
class TimeLimitedCache {
private cache = new Map<number, Value>();
set(key: number, value: number, duration: number): boolean {
const now = Date.now();
const exists = this.getValue(now, key) !== undefined;
this.cache.set(key, { value, expiredAt: now + duration });
return exists;
}
get(key: number): number {
const val = this.getValue(Date.now(), key);
return val === undefined ? -1 : val.value;
}
count(): number {
const now = Date.now();
for (const key of this.cache.keys())
if (this.getValue(now, key) === undefined) {
this.cache.delete(key);
}
return this.cache.size;
}
private getValue(now: number, key: number): Value | undefined {
const val = this.cache.get(key);
return val && now <= val.expiredAt ? val : undefined;
}
}
/* code provided by PROGIEZ */
2622. Cache With Time Limit LeetCode Solution in Java
interface TTLValue {
value: number;
timer: ReturnType<typeof setTimeout>;
}
class TimeLimitedCache {
private cache = new Map<number, TTLValue>();
set(key: number, value: number, duration: number): boolean {
const exists = this.cache.has(key);
if (exists) {
clearTimeout(this.cache.get(key).timer);
}
this.cache.set(key, {
value,
timer: setTimeout(() => this.cache.delete(key), duration),
});
return exists;
}
get(key: number): number {
return this.cache.has(key) ? this.cache.get(key).value : -1;
}
count(): number {
return this.cache.size;
}
}
// code provided by PROGIEZ
2622. Cache With Time Limit LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.