707. Design Linked List LeetCode Solution
In this guide, you will get 707. Design Linked List LeetCode Solution with the best time and space complexity. The solution to Design Linked List problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Design Linked List solution in C++
- Design Linked List solution in Java
- Design Linked List solution in Python
- Additional Resources
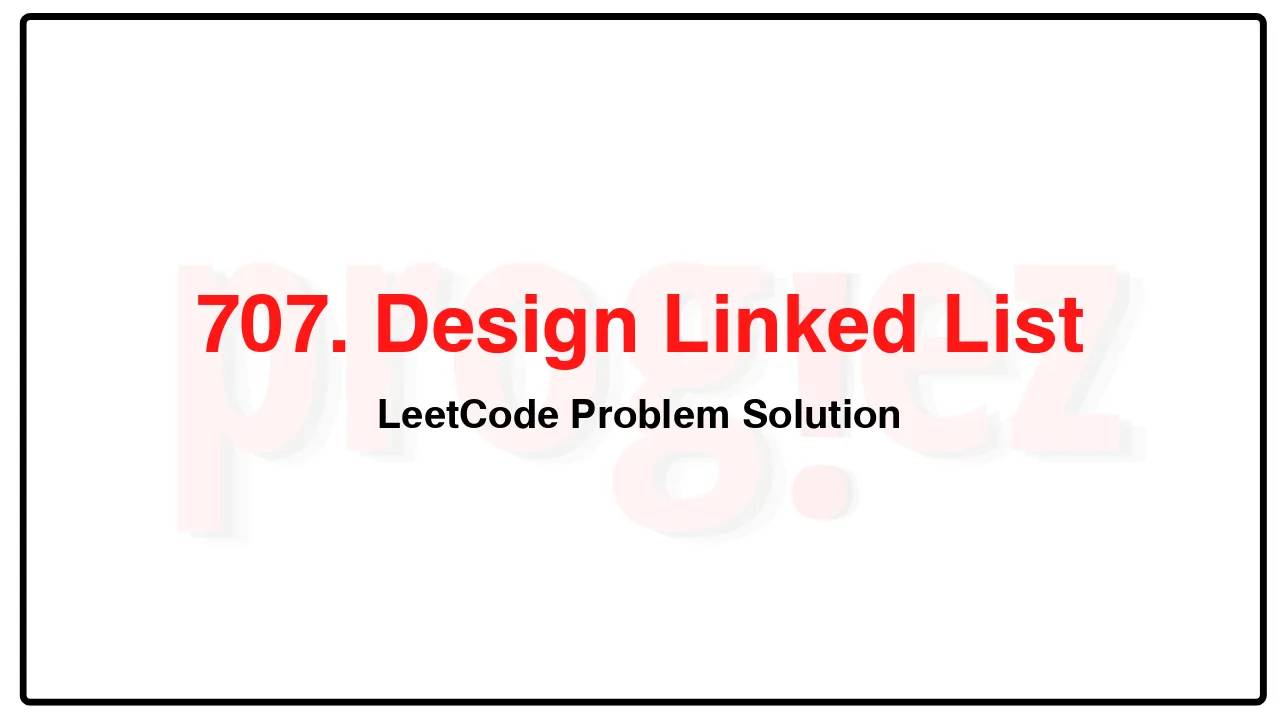
Problem Statement of Design Linked List
Design your implementation of the linked list. You can choose to use a singly or doubly linked list.
A node in a singly linked list should have two attributes: val and next. val is the value of the current node, and next is a pointer/reference to the next node.
If you want to use the doubly linked list, you will need one more attribute prev to indicate the previous node in the linked list. Assume all nodes in the linked list are 0-indexed.
Implement the MyLinkedList class:
MyLinkedList() Initializes the MyLinkedList object.
int get(int index) Get the value of the indexth node in the linked list. If the index is invalid, return -1.
void addAtHead(int val) Add a node of value val before the first element of the linked list. After the insertion, the new node will be the first node of the linked list.
void addAtTail(int val) Append a node of value val as the last element of the linked list.
void addAtIndex(int index, int val) Add a node of value val before the indexth node in the linked list. If index equals the length of the linked list, the node will be appended to the end of the linked list. If index is greater than the length, the node will not be inserted.
void deleteAtIndex(int index) Delete the indexth node in the linked list, if the index is valid.
Example 1:
Input
[“MyLinkedList”, “addAtHead”, “addAtTail”, “addAtIndex”, “get”, “deleteAtIndex”, “get”]
[[], [1], [3], [1, 2], [1], [1], [1]]
Output
[null, null, null, null, 2, null, 3]
Explanation
MyLinkedList myLinkedList = new MyLinkedList();
myLinkedList.addAtHead(1);
myLinkedList.addAtTail(3);
myLinkedList.addAtIndex(1, 2); // linked list becomes 1->2->3
myLinkedList.get(1); // return 2
myLinkedList.deleteAtIndex(1); // now the linked list is 1->3
myLinkedList.get(1); // return 3
Constraints:
0 <= index, val <= 1000
Please do not use the built-in LinkedList library.
At most 2000 calls will be made to get, addAtHead, addAtTail, addAtIndex and deleteAtIndex.
Complexity Analysis
- Time Complexity: O(n)`
- Space Complexity: O(n)
707. Design Linked List LeetCode Solution in C++
class MyLinkedList {
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
public:
int get(int index) {
if (index < 0 || index >= length)
return -1;
ListNode* curr = dummy.next;
for (int i = 0; i < index; ++i)
curr = curr->next;
return curr->val;
}
void addAtHead(int val) {
ListNode* head = dummy.next;
ListNode* node = new ListNode(val);
node->next = head;
dummy.next = node;
++length;
}
void addAtTail(int val) {
ListNode* curr = &dummy;
while (curr->next != nullptr)
curr = curr->next;
curr->next = new ListNode(val);
++length;
}
void addAtIndex(int index, int val) {
if (index > length)
return;
ListNode* curr = &dummy;
for (int i = 0; i < index; ++i)
curr = curr->next;
ListNode* cache = curr->next;
ListNode* node = new ListNode(val);
node->next = cache;
curr->next = node;
++length;
}
void deleteAtIndex(int index) {
if (index < 0 || index >= length)
return;
ListNode* curr = &dummy;
for (int i = 0; i < index; ++i)
curr = curr->next;
ListNode* cache = curr->next;
curr->next = cache->next;
--length;
delete cache;
}
private:
int length = 0;
ListNode dummy = ListNode(0);
};
/* code provided by PROGIEZ */
707. Design Linked List LeetCode Solution in Java
class MyLinkedList {
private class ListNode {
int val;
ListNode next;
public ListNode(int val) {
this.val = val;
this.next = null;
}
}
public int get(int index) {
if (index < 0 || index >= length)
return -1;
ListNode curr = dummy.next;
for (int i = 0; i < index; ++i)
curr = curr.next;
return curr.val;
}
public void addAtHead(int val) {
ListNode head = dummy.next;
ListNode node = new ListNode(val);
node.next = head;
dummy.next = node;
++length;
}
public void addAtTail(int val) {
ListNode curr = dummy;
while (curr.next != null)
curr = curr.next;
curr.next = new ListNode(val);
++length;
}
public void addAtIndex(int index, int val) {
if (index > length)
return;
ListNode curr = dummy;
for (int i = 0; i < index; ++i)
curr = curr.next;
ListNode cache = curr.next;
ListNode node = new ListNode(val);
node.next = cache;
curr.next = node;
++length;
}
public void deleteAtIndex(int index) {
if (index < 0 || index >= length)
return;
ListNode curr = dummy;
for (int i = 0; i < index; ++i)
curr = curr.next;
ListNode cache = curr.next;
curr.next = cache.next;
--length;
}
int length = 0;
ListNode dummy = new ListNode(0);
}
// code provided by PROGIEZ
707. Design Linked List LeetCode Solution in Python
from dataclasses import dataclass
@dataclass
class ListNode:
val: int
next: ListNode | None = None
class MyLinkedList:
def __init__(self):
self.length = 0
self.dummy = ListNode(0)
def get(self, index: int) -> int:
if index < 0 or index >= self.length:
return -1
curr = self.dummy.next
for _ in range(index):
curr = curr.next
return curr.val
def addAtHead(self, val: int) -> None:
curr = self.dummy.next
self.dummy.next = ListNode(val)
self.dummy.next.next = curr
self.length += 1
def addAtTail(self, val: int) -> None:
curr = self.dummy
while curr.next:
curr = curr.next
curr.next = ListNode(val)
self.length += 1
def addAtIndex(self, index: int, val: int) -> None:
if index > self.length:
return
curr = self.dummy
for _ in range(index):
curr = curr.next
temp = curr.next
curr.next = ListNode(val)
curr.next.next = temp
self.length += 1
def deleteAtIndex(self, index: int) -> None:
if index < 0 or index >= self.length:
return
curr = self.dummy
for _ in range(index):
curr = curr.next
temp = curr.next
curr.next = temp.next
self.length -= 1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.