217. Contains Duplicate LeetCode Solution
In this guide, you will get 217. Contains Duplicate LeetCode Solution with the best time and space complexity. The solution to Contains Duplicate problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Contains Duplicate solution in C++
- Contains Duplicate solution in Java
- Contains Duplicate solution in Python
- Additional Resources
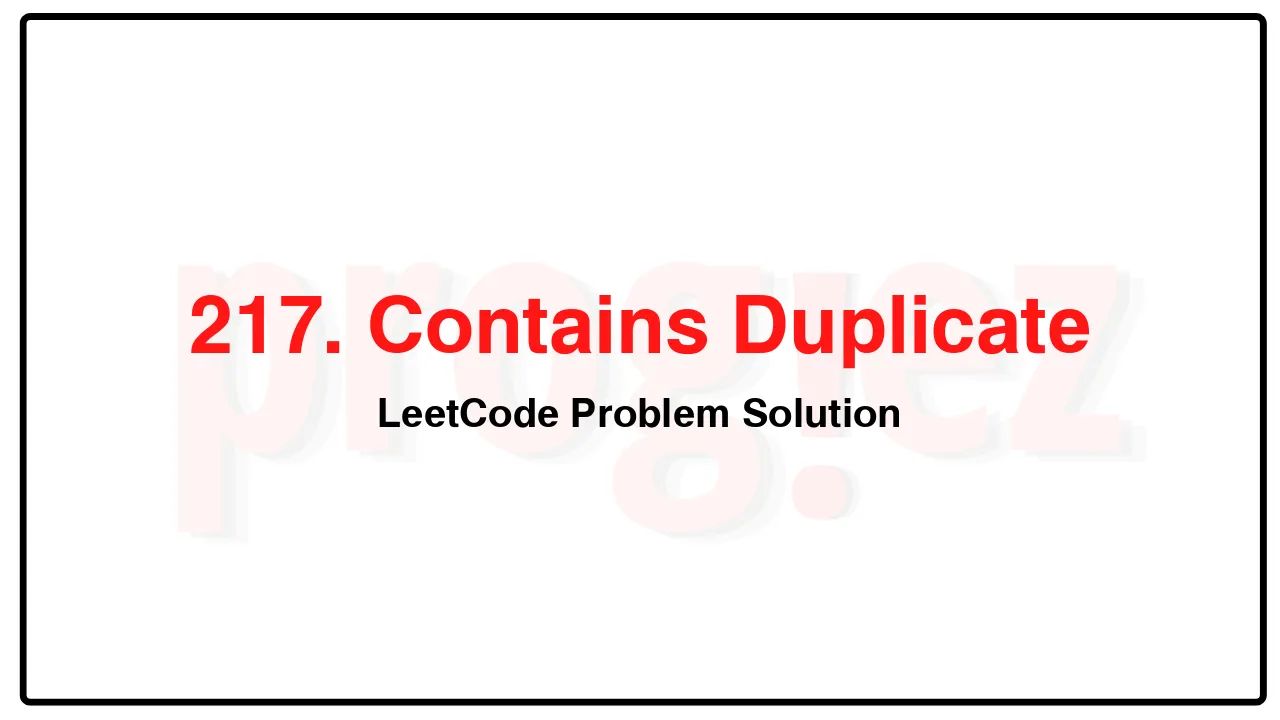
Problem Statement of Contains Duplicate
Given an integer array nums, return true if any value appears at least twice in the array, and return false if every element is distinct.
Example 1:
Input: nums = [1,2,3,1]
Output: true
Explanation:
The element 1 occurs at the indices 0 and 3.
Example 2:
Input: nums = [1,2,3,4]
Output: false
Explanation:
All elements are distinct.
Example 3:
Input: nums = [1,1,1,3,3,4,3,2,4,2]
Output: true
Constraints:
1 <= nums.length <= 105
-109 <= nums[i] <= 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
217. Contains Duplicate LeetCode Solution in C++
class Solution {
public:
bool containsDuplicate(vector<int>& nums) {
unordered_set<int> seen;
for (const int num : nums)
if (!seen.insert(num).second)
return true;
return false;
}
};
/* code provided by PROGIEZ */
217. Contains Duplicate LeetCode Solution in Java
class Solution {
public boolean containsDuplicate(int[] nums) {
Set<Integer> seen = new HashSet<>();
for (final int num : nums)
if (!seen.add(num))
return true;
return false;
}
}
// code provided by PROGIEZ
217. Contains Duplicate LeetCode Solution in Python
class Solution:
def containsDuplicate(self, nums: list[int]) -> bool:
return len(nums) != len(set(nums))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.