172. Factorial Trailing Zeroes LeetCode Solution
In this guide, you will get 172. Factorial Trailing Zeroes LeetCode Solution with the best time and space complexity. The solution to Factorial Trailing Zeroes problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Factorial Trailing Zeroes solution in C++
- Factorial Trailing Zeroes solution in Java
- Factorial Trailing Zeroes solution in Python
- Additional Resources
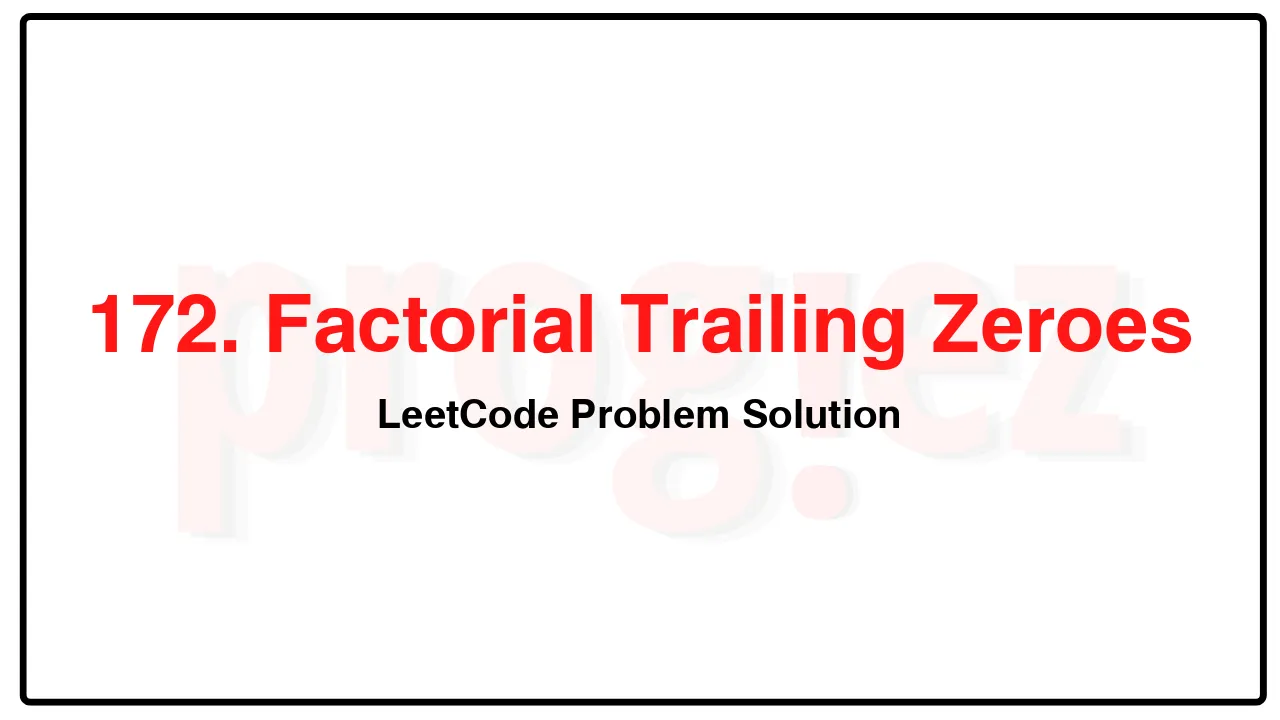
Problem Statement of Factorial Trailing Zeroes
Given an integer n, return the number of trailing zeroes in n!.
Note that n! = n * (n – 1) * (n – 2) * … * 3 * 2 * 1.
Example 1:
Input: n = 3
Output: 0
Explanation: 3! = 6, no trailing zero.
Example 2:
Input: n = 5
Output: 1
Explanation: 5! = 120, one trailing zero.
Example 3:
Input: n = 0
Output: 0
Constraints:
0 <= n <= 104
Follow up: Could you write a solution that works in logarithmic time complexity?
Complexity Analysis
- Time Complexity: O(\log_5 n)
- Space Complexity: O(1)
172. Factorial Trailing Zeroes LeetCode Solution in C++
class Solution {
public:
int trailingZeroes(int n) {
return n == 0 ? 0 : n / 5 + trailingZeroes(n / 5);
}
};
/* code provided by PROGIEZ */
172. Factorial Trailing Zeroes LeetCode Solution in Java
class Solution {
public int trailingZeroes(int n) {
return n == 0 ? 0 : n / 5 + trailingZeroes(n / 5);
}
}
// code provided by PROGIEZ
172. Factorial Trailing Zeroes LeetCode Solution in Python
class Solution:
def trailingZeroes(self, n: int) -> int:
return 0 if n == 0 else n // 5 + self.trailingZeroes(n // 5)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.