166. Fraction to Recurring Decimal LeetCode Solution
In this guide, you will get 166. Fraction to Recurring Decimal LeetCode Solution with the best time and space complexity. The solution to Fraction to Recurring Decimal problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Fraction to Recurring Decimal solution in C++
- Fraction to Recurring Decimal solution in Java
- Fraction to Recurring Decimal solution in Python
- Additional Resources
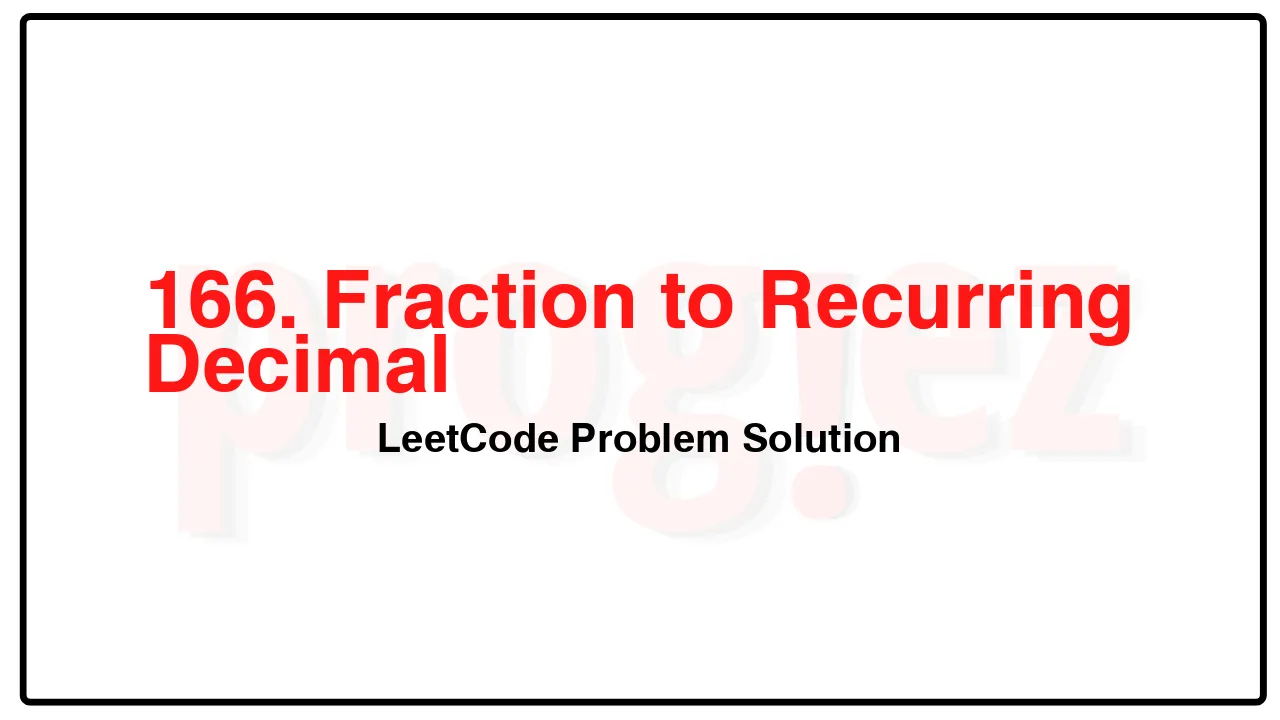
Problem Statement of Fraction to Recurring Decimal
Given two integers representing the numerator and denominator of a fraction, return the fraction in string format.
If the fractional part is repeating, enclose the repeating part in parentheses.
If multiple answers are possible, return any of them.
It is guaranteed that the length of the answer string is less than 104 for all the given inputs.
Example 1:
Input: numerator = 1, denominator = 2
Output: “0.5”
Example 2:
Input: numerator = 2, denominator = 1
Output: “2”
Example 3:
Input: numerator = 4, denominator = 333
Output: “0.(012)”
Constraints:
-231 <= numerator, denominator <= 231 – 1
denominator != 0
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
166. Fraction to Recurring Decimal LeetCode Solution in C++
class Solution {
public:
string fractionToDecimal(int numerator, int denominator) {
if (numerator == 0)
return "0";
string ans;
if (numerator < 0 ^ denominator < 0)
ans += "-";
long n = labs(numerator);
long d = labs(denominator);
ans += to_string(n / d);
if (n % d == 0)
return ans;
ans += '.';
unordered_map<int, int> seen;
for (long r = n % d; r; r %= d) {
if (const auto it = seen.find(r); it != seen.cend()) {
ans.insert(it->second, 1, '(');
ans += ')';
break;
}
seen[r] = ans.size();
r *= 10;
ans += to_string(r / d);
}
return ans;
}
};
/* code provided by PROGIEZ */
166. Fraction to Recurring Decimal LeetCode Solution in Java
class Solution {
public String fractionToDecimal(int numerator, int denominator) {
if (numerator == 0)
return "0";
StringBuilder sb = new StringBuilder();
if (numerator < 0 ^ denominator < 0)
sb.append("-");
long n = Math.abs((long) numerator);
long d = Math.abs((long) denominator);
sb.append(n / d);
if (n % d == 0)
return sb.toString();
sb.append(".");
Map<Long, Integer> seen = new HashMap<>();
for (long r = n % d; r > 0; r %= d) {
if (seen.containsKey(r)) {
sb.insert(seen.get(r), "(");
sb.append(")");
break;
}
seen.put(r, sb.length());
r *= 10;
sb.append(r / d);
}
return sb.toString();
}
}
// code provided by PROGIEZ
166. Fraction to Recurring Decimal LeetCode Solution in Python
class Solution:
def fractionToDecimal(self, numerator: int, denominator: int) -> str:
if numerator == 0:
return '0'
ans = ''
if (numerator < 0) ^ (denominator < 0):
ans += '-'
numerator = abs(numerator)
denominator = abs(denominator)
ans += str(numerator // denominator)
if numerator % denominator == 0:
return ans
ans += '.'
dict = {}
remainder = numerator % denominator
while remainder:
if remainder in dict:
ans = ans[:dict[remainder]] + '(' + ans[dict[remainder]:] + ')'
break
dict[remainder] = len(ans)
remainder *= 10
ans += str(remainder // denominator)
remainder %= denominator
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.