165. Compare Version Numbers LeetCode Solution
In this guide, you will get 165. Compare Version Numbers LeetCode Solution with the best time and space complexity. The solution to Compare Version Numbers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Compare Version Numbers solution in C++
- Compare Version Numbers solution in Java
- Compare Version Numbers solution in Python
- Additional Resources
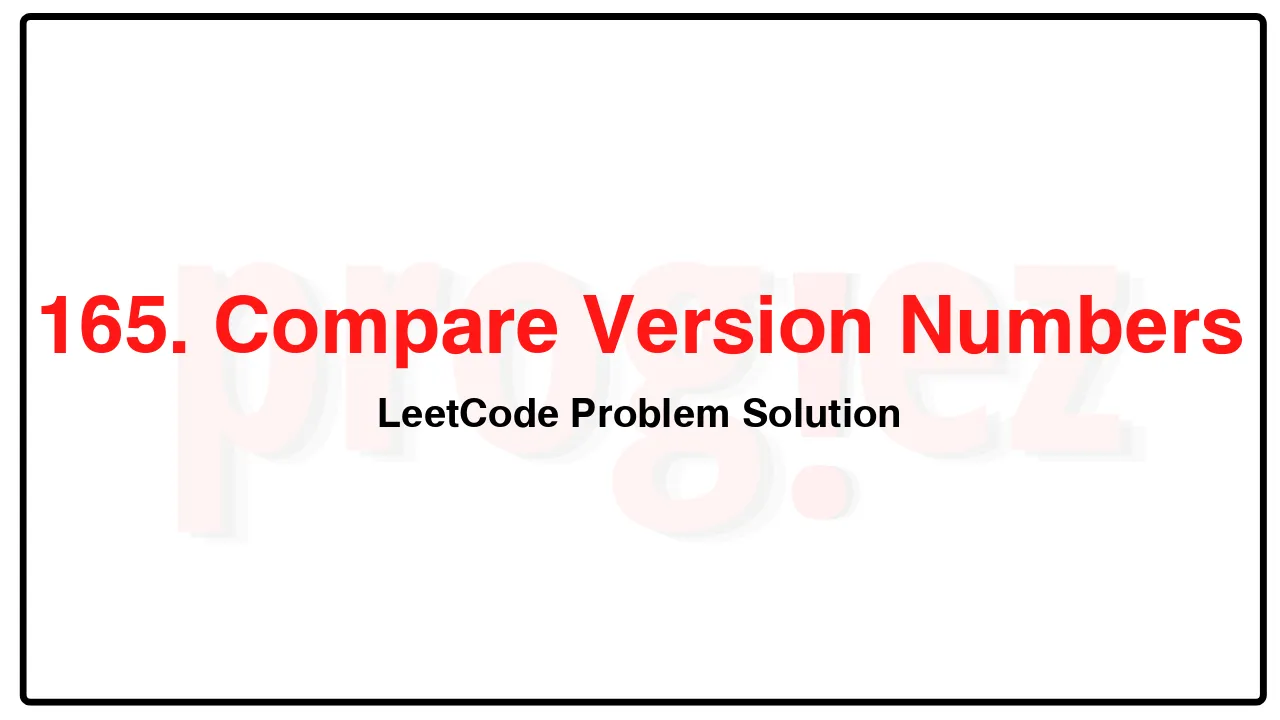
Problem Statement of Compare Version Numbers
Given two version strings, version1 and version2, compare them. A version string consists of revisions separated by dots ‘.’. The value of the revision is its integer conversion ignoring leading zeros.
To compare version strings, compare their revision values in left-to-right order. If one of the version strings has fewer revisions, treat the missing revision values as 0.
Return the following:
If version1 version2, return 1.
Otherwise, return 0.
Example 1:
Input: version1 = “1.2”, version2 = “1.10”
Output: -1
Explanation:
version1’s second revision is “2” and version2’s second revision is “10”: 2 < 10, so version1 < version2.
Example 2:
Input: version1 = "1.01", version2 = "1.001"
Output: 0
Explanation:
Ignoring leading zeroes, both "01" and "001" represent the same integer "1".
Example 3:
Input: version1 = "1.0", version2 = "1.0.0.0"
Output: 0
Explanation:
version1 has less revisions, which means every missing revision are treated as "0".
Constraints:
1 <= version1.length, version2.length <= 500
version1 and version2 only contain digits and '.'.
version1 and version2 are valid version numbers.
All the given revisions in version1 and version2 can be stored in a 32-bit integer.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
165. Compare Version Numbers LeetCode Solution in C++
class Solution {
public:
int compareVersion(string version1, string version2) {
istringstream iss1(version1);
istringstream iss2(version2);
int v1;
int v2;
char dotChar;
while (bool(iss1 >> v1) + bool(iss2 >> v2)) {
if (v1 < v2)
return -1;
if (v1 > v2)
return 1;
iss1 >> dotChar;
iss2 >> dotChar;
v1 = 0;
v2 = 0;
}
return 0;
};
};
/* code provided by PROGIEZ */
165. Compare Version Numbers LeetCode Solution in Java
class Solution {
public int compareVersion(String version1, String version2) {
final String[] levels1 = version1.split("\\.");
final String[] levels2 = version2.split("\\.");
final int length = Math.max(levels1.length, levels2.length);
for (int i = 0; i < length; ++i) {
final Integer v1 = i < levels1.length ? Integer.parseInt(levels1[i]) : 0;
final Integer v2 = i < levels2.length ? Integer.parseInt(levels2[i]) : 0;
final int compare = v1.compareTo(v2);
if (compare != 0)
return compare;
}
return 0;
}
}
// code provided by PROGIEZ
165. Compare Version Numbers LeetCode Solution in Python
class Solution:
def compareVersion(self, version1: str, version2: str) -> int:
levels1 = version1.split('.')
levels2 = version2.split('.')
length = max(len(levels1), len(levels2))
for i in range(length):
v1 = int(levels1[i]) if i < len(levels1) else 0
v2 = int(levels2[i]) if i < len(levels2) else 0
if v1 < v2:
return -1
if v1 > v2:
return 1
return 0
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.