162. Find Peak ElementLeetCode Solution
In this guide, you will get 162. Find Peak ElementLeetCode Solution with the best time and space complexity. The solution to Find Peak Element problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find Peak Element solution in C++
- Find Peak Element solution in Java
- Find Peak Element solution in Python
- Additional Resources
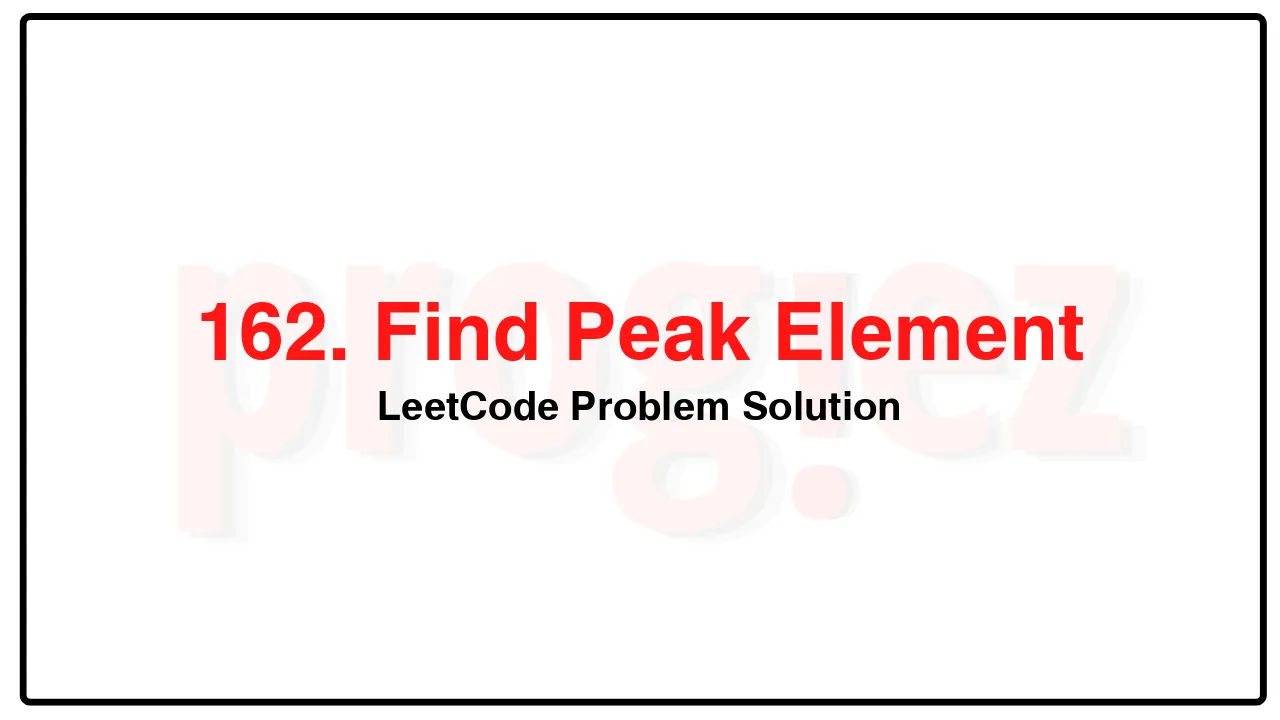
Problem Statement of Find Peak Element
A peak element is an element that is strictly greater than its neighbors.
Given a 0-indexed integer array nums, find a peak element, and return its index. If the array contains multiple peaks, return the index to any of the peaks.
You may imagine that nums[-1] = nums[n] = -∞. In other words, an element is always considered to be strictly greater than a neighbor that is outside the array.
You must write an algorithm that runs in O(log n) time.
Example 1:
Input: nums = [1,2,3,1]
Output: 2
Explanation: 3 is a peak element and your function should return the index number 2.
Example 2:
Input: nums = [1,2,1,3,5,6,4]
Output: 5
Explanation: Your function can return either index number 1 where the peak element is 2, or index number 5 where the peak element is 6.
Constraints:
1 <= nums.length <= 1000
-231 <= nums[i] <= 231 – 1
nums[i] != nums[i + 1] for all valid i.
Complexity Analysis
- Time Complexity: O(\log n)
- Space Complexity: O(1)
162. Find Peak ElementLeetCode Solution in C++
class Solution {
public:
int findPeakElement(vector<int>& nums) {
int l = 0;
int r = nums.size() - 1;
while (l < r) {
const int m = (l + r) / 2;
if (nums[m] >= nums[m + 1])
r = m;
else
l = m + 1;
}
return l;
}
};
/* code provided by PROGIEZ */
162. Find Peak ElementLeetCode Solution in Java
class Solution {
public int findPeakElement(int[] nums) {
int l = 0;
int r = nums.length - 1;
while (l < r) {
final int m = (l + r) / 2;
if (nums[m] >= nums[m + 1])
r = m;
else
l = m + 1;
}
return l;
}
}
// code provided by PROGIEZ
162. Find Peak ElementLeetCode Solution in Python
class Solution:
def findPeakElement(self, nums: list[int]) -> int:
l = 0
r = len(nums) - 1
while l < r:
m = (l + r) // 2
if nums[m] >= nums[m + 1]:
r = m
else:
l = m + 1
return l
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.