1414. Find the Minimum Number of Fibonacci Numbers Whose Sum Is K LeetCode Solution
In this guide, you will get 1414. Find the Minimum Number of Fibonacci Numbers Whose Sum Is K LeetCode Solution with the best time and space complexity. The solution to Find the Minimum Number of Fibonacci Numbers Whose Sum Is K problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find the Minimum Number of Fibonacci Numbers Whose Sum Is K solution in C++
- Find the Minimum Number of Fibonacci Numbers Whose Sum Is K solution in Java
- Find the Minimum Number of Fibonacci Numbers Whose Sum Is K solution in Python
- Additional Resources
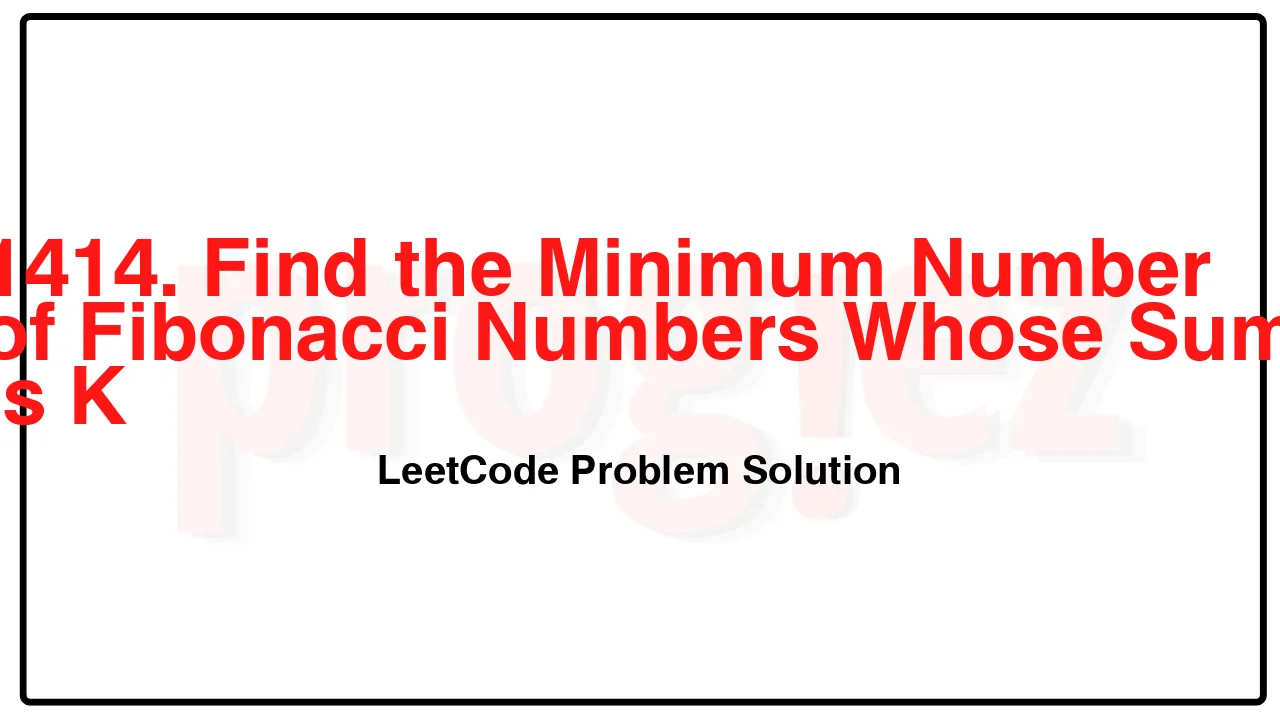
Problem Statement of Find the Minimum Number of Fibonacci Numbers Whose Sum Is K
Given an integer k, return the minimum number of Fibonacci numbers whose sum is equal to k. The same Fibonacci number can be used multiple times.
The Fibonacci numbers are defined as:
F1 = 1
F2 = 1
Fn = Fn-1 + Fn-2 for n > 2.
It is guaranteed that for the given constraints we can always find such Fibonacci numbers that sum up to k.
Example 1:
Input: k = 7
Output: 2
Explanation: The Fibonacci numbers are: 1, 1, 2, 3, 5, 8, 13, …
For k = 7 we can use 2 + 5 = 7.
Example 2:
Input: k = 10
Output: 2
Explanation: For k = 10 we can use 2 + 8 = 10.
Example 3:
Input: k = 19
Output: 3
Explanation: For k = 19 we can use 1 + 5 + 13 = 19.
Constraints:
1 <= k <= 109
Complexity Analysis
- Time Complexity: O((\log k)^2)
- Space Complexity: O(\log k)
1414. Find the Minimum Number of Fibonacci Numbers Whose Sum Is K LeetCode Solution in C++
class Solution {
public:
int findMinFibonacciNumbers(int k) {
if (k < 2) // k == 0 || k == 1
return k;
int a = 1; // F_1
int b = 1; // F_2
while (b <= k) {
// a, b = F_{i + 1}, F_{i + 2}
// -> a, b = F_{i + 2}, F_{i + 3}
const int temp = a;
a = b;
b = a + temp;
}
return 1 + findMinFibonacciNumbers(k - a);
}
};
/* code provided by PROGIEZ */
1414. Find the Minimum Number of Fibonacci Numbers Whose Sum Is K LeetCode Solution in Java
class Solution {
public int findMinFibonacciNumbers(int k) {
if (k < 2) // k == 0 || k == 1
return k;
int a = 1; // F_1
int b = 1; // F_2
while (b <= k) {
// a, b = F_{i + 1}, F_{i + 2}
// -> a, b = F_{i + 2}, F_{i + 3}
final int temp = a;
a = b;
b = a + temp;
}
return 1 + findMinFibonacciNumbers(k - a);
}
}
// code provided by PROGIEZ
1414. Find the Minimum Number of Fibonacci Numbers Whose Sum Is K LeetCode Solution in Python
class Solution:
def findMinFibonacciNumbers(self, k: int) -> int:
if k < 2: # k == 0 or k == 1
return k
a = 1 # F_1
b = 1 # F_2
while b <= k:
# a, b = F_{i + 1}, F_{i + 2}
# -> a, b = F_{i + 2}, F_{i + 3}
a, b = b, a + b
return 1 + self.findMinFibonacciNumbers(k - a)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.