2899. Last Visited Integers LeetCode Solution
In this guide, you will get 2899. Last Visited Integers LeetCode Solution with the best time and space complexity. The solution to Last Visited Integers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Last Visited Integers solution in C++
- Last Visited Integers solution in Java
- Last Visited Integers solution in Python
- Additional Resources
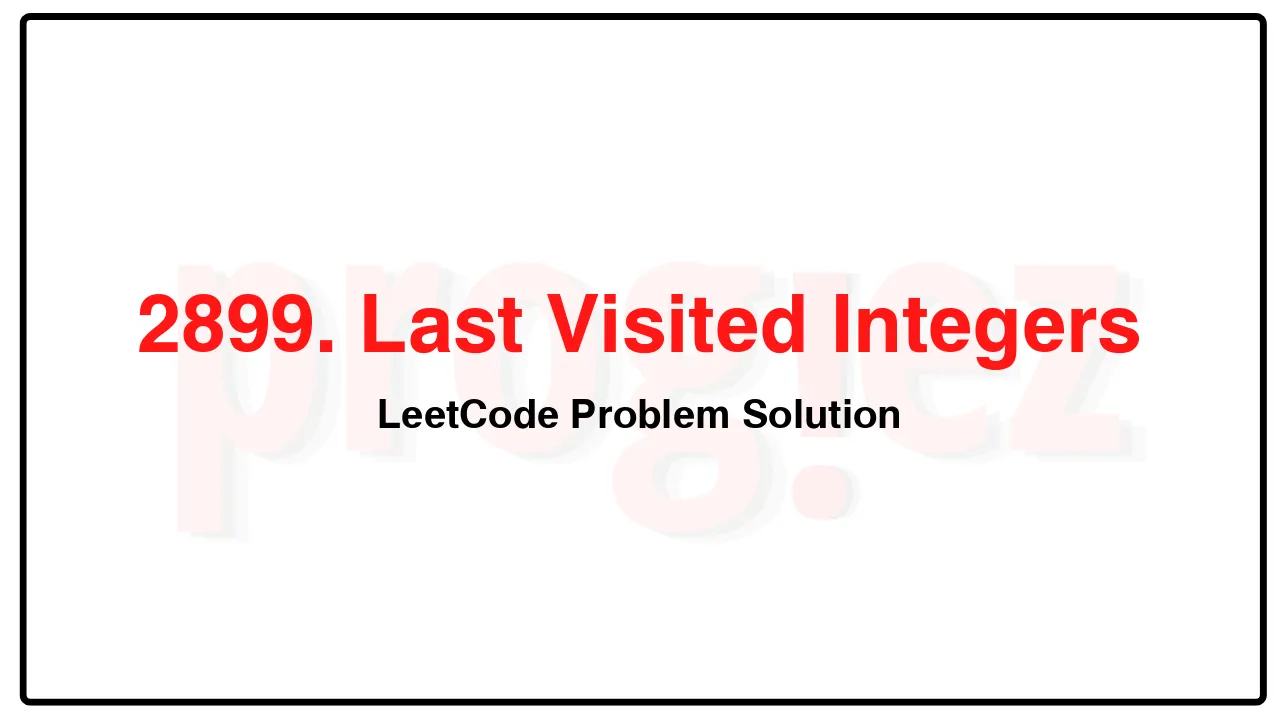
Problem Statement of Last Visited Integers
Given an integer array nums where nums[i] is either a positive integer or -1. We need to find for each -1 the respective positive integer, which we call the last visited integer.
To achieve this goal, let’s define two empty arrays: seen and ans.
Start iterating from the beginning of the array nums.
If a positive integer is encountered, prepend it to the front of seen.
If -1 is encountered, let k be the number of consecutive -1s seen so far (including the current -1),
If k is less than or equal to the length of seen, append the k-th element of seen to ans.
If k is strictly greater than the length of seen, append -1 to ans.
Return the array ans.
Example 1:
Input: nums = [1,2,-1,-1,-1]
Output: [2,1,-1]
Explanation:
Start with seen = [] and ans = [].
Process nums[0]: The first element in nums is 1. We prepend it to the front of seen. Now, seen == [1].
Process nums[1]: The next element is 2. We prepend it to the front of seen. Now, seen == [2, 1].
Process nums[2]: The next element is -1. This is the first occurrence of -1, so k == 1. We look for the first element in seen. We append 2 to ans. Now, ans == [2].
Process nums[3]: Another -1. This is the second consecutive -1, so k == 2. The second element in seen is 1, so we append 1 to ans. Now, ans == [2, 1].
Process nums[4]: Another -1, the third in a row, making k = 3. However, seen only has two elements ([2, 1]). Since k is greater than the number of elements in seen, we append -1 to ans. Finally, ans == [2, 1, -1].
Example 2:
Input: nums = [1,-1,2,-1,-1]
Output: [1,2,1]
Explanation:
Start with seen = [] and ans = [].
Process nums[0]: The first element in nums is 1. We prepend it to the front of seen. Now, seen == [1].
Process nums[1]: The next element is -1. This is the first occurrence of -1, so k == 1. We look for the first element in seen, which is 1. Append 1 to ans. Now, ans == [1].
Process nums[2]: The next element is 2. Prepend this to the front of seen. Now, seen == [2, 1].
Process nums[3]: The next element is -1. This -1 is not consecutive to the first -1 since 2 was in between. Thus, k resets to 1. The first element in seen is 2, so append 2 to ans. Now, ans == [1, 2].
Process nums[4]: Another -1. This is consecutive to the previous -1, so k == 2. The second element in seen is 1, append 1 to ans. Finally, ans == [1, 2, 1].
Constraints:
1 <= nums.length <= 100
nums[i] == -1 or 1 <= nums[i] <= 100
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
2899. Last Visited Integers LeetCode Solution in C++
class Solution {
public:
vector<int> lastVisitedIntegers(vector<string>& words) {
vector<int> ans;
vector<int> nums;
int k = 0;
for (const string& word : words)
if (word == "prev") {
++k;
ans.push_back(k > nums.size() ? -1 : nums[nums.size() - k]);
} else {
k = 0;
nums.push_back(stoi(word));
}
return ans;
}
};
/* code provided by PROGIEZ */
2899. Last Visited Integers LeetCode Solution in Java
class Solution {
public List<Integer> lastVisitedIntegers(List<String> words) {
List<Integer> ans = new ArrayList<>();
List<Integer> nums = new ArrayList<>();
int k = 0;
for (final String word : words)
if (word.equals("prev")) {
++k;
ans.add(k > nums.size() ? -1 : nums.get(nums.size() - k));
} else {
k = 0;
nums.add(Integer.valueOf(word));
}
return ans;
}
}
// code provided by PROGIEZ
2899. Last Visited Integers LeetCode Solution in Python
class Solution:
def lastVisitedIntegers(self, words: list[str]) -> list[int]:
ans = []
nums = []
k = 0
for word in words:
if word == 'prev':
k += 1
ans.append(-1 if k > len(nums) else nums[-k])
else:
k = 0
nums.append(int(word))
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.