1861. Rotating the Box LeetCode Solution
In this guide, you will get 1861. Rotating the Box LeetCode Solution with the best time and space complexity. The solution to Rotating the Box problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Rotating the Box solution in C++
- Rotating the Box solution in Java
- Rotating the Box solution in Python
- Additional Resources
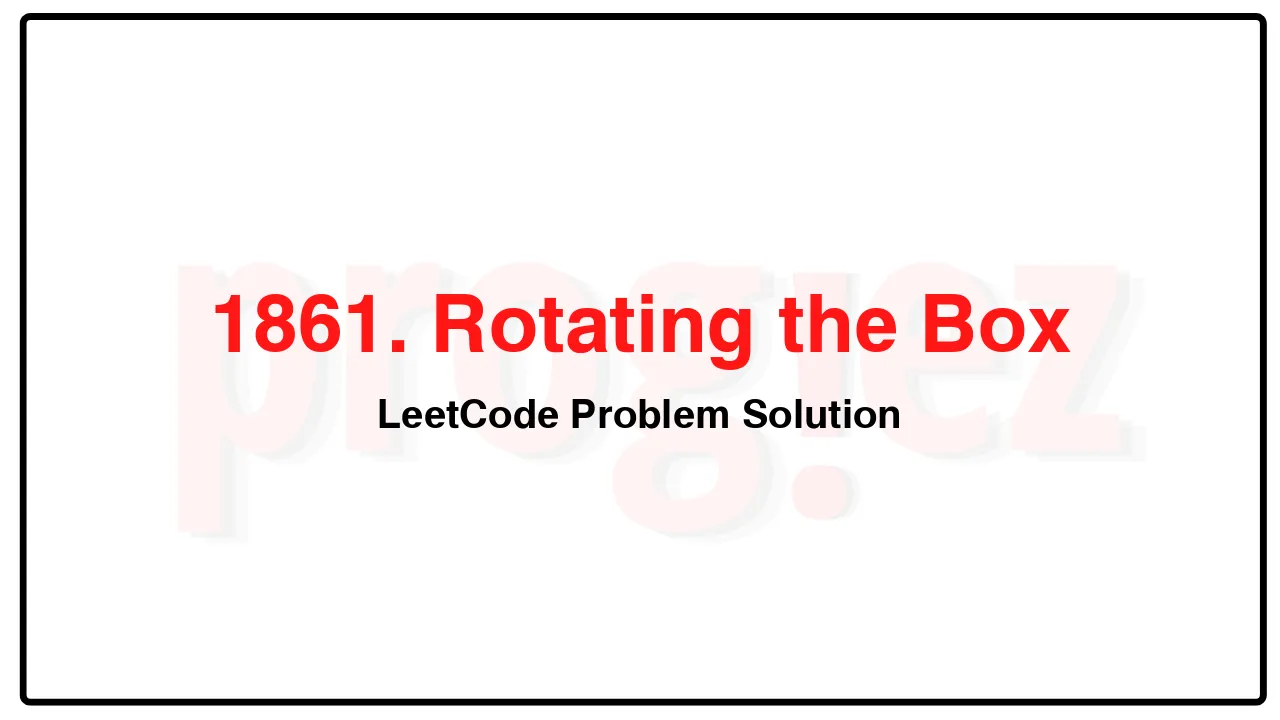
Problem Statement of Rotating the Box
You are given an m x n matrix of characters boxGrid representing a side-view of a box. Each cell of the box is one of the following:
A stone ‘#’
A stationary obstacle ‘*’
Empty ‘.’
The box is rotated 90 degrees clockwise, causing some of the stones to fall due to gravity. Each stone falls down until it lands on an obstacle, another stone, or the bottom of the box. Gravity does not affect the obstacles’ positions, and the inertia from the box’s rotation does not affect the stones’ horizontal positions.
It is guaranteed that each stone in boxGrid rests on an obstacle, another stone, or the bottom of the box.
Return an n x m matrix representing the box after the rotation described above.
Example 1:
Input: boxGrid = [[“#”,”.”,”#”]]
Output: [[“.”],
[“#”],
[“#”]]
Example 2:
Input: boxGrid = [[“#”,”.”,”*”,”.”],
[“#”,”#”,”*”,”.”]]
Output: [[“#”,”.”],
[“#”,”#”],
[“*”,”*”],
[“.”,”.”]]
Example 3:
Input: boxGrid = [[“#”,”#”,”*”,”.”,”*”,”.”],
[“#”,”#”,”#”,”*”,”.”,”.”],
[“#”,”#”,”#”,”.”,”#”,”.”]]
Output: [[“.”,”#”,”#”],
[“.”,”#”,”#”],
[“#”,”#”,”*”],
[“#”,”*”,”.”],
[“#”,”.”,”*”],
[“#”,”.”,”.”]]
Constraints:
m == boxGrid.length
n == boxGrid[i].length
1 <= m, n <= 500
boxGrid[i][j] is either '#', '*', or '.'.
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(mn)
1861. Rotating the Box LeetCode Solution in C++
class Solution {
public:
vector<vector<char>> rotateTheBox(vector<vector<char>>& box) {
const int m = box.size();
const int n = box[0].size();
vector<vector<char>> ans(n, vector<char>(m, '.'));
for (int i = 0; i < m; ++i)
for (int j = n - 1, k = n - 1; j >= 0; --j)
if (box[i][j] != '.') {
if (box[i][j] == '*')
k = j;
ans[k--][m - i - 1] = box[i][j];
}
return ans;
}
};
/* code provided by PROGIEZ */
1861. Rotating the Box LeetCode Solution in Java
class Solution {
public char[][] rotateTheBox(char[][] box) {
final int m = box.length;
final int n = box[0].length;
char[][] ans = new char[n][m];
Arrays.stream(ans).forEach(A -> Arrays.fill(A, '.'));
for (int i = 0; i < m; ++i)
for (int j = n - 1, k = n - 1; j >= 0; --j)
if (box[i][j] != '.') {
if (box[i][j] == '*')
k = j;
ans[k--][m - i - 1] = box[i][j];
}
return ans;
}
}
// code provided by PROGIEZ
1861. Rotating the Box LeetCode Solution in Python
class Solution:
def rotateTheBox(self, box: list[list[str]]) -> list[list[str]]:
m = len(box)
n = len(box[0])
rotated = [['.'] * m for _ in range(n)]
for i in range(m):
k = n - 1
for j in reversed(range(n)):
if box[i][j] != '.':
if box[i][j] == '*':
k = j
rotated[k][m - i - 1] = box[i][j]
k -= 1
return [''.join(row) for row in rotated]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.