1752. Check if Array Is Sorted and Rotated LeetCode Solution
In this guide, you will get 1752. Check if Array Is Sorted and Rotated LeetCode Solution with the best time and space complexity. The solution to Check if Array Is Sorted and Rotated problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Check if Array Is Sorted and Rotated solution in C++
- Check if Array Is Sorted and Rotated solution in Java
- Check if Array Is Sorted and Rotated solution in Python
- Additional Resources
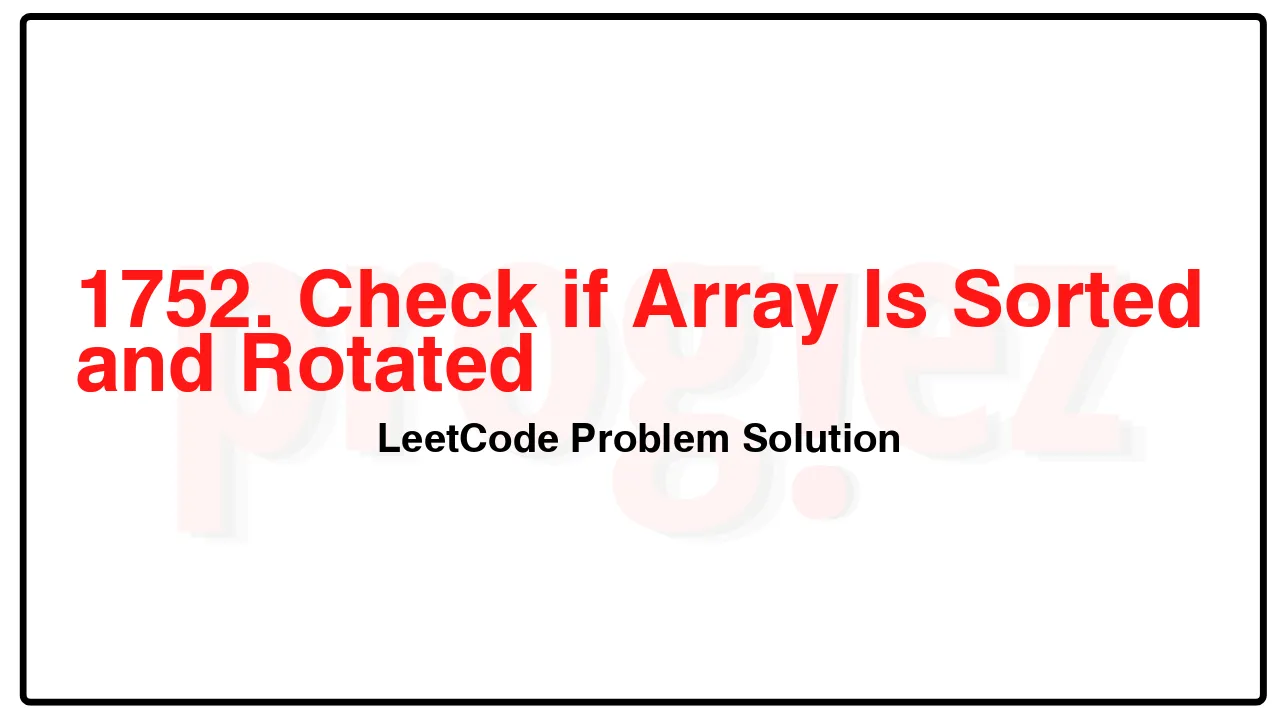
Problem Statement of Check if Array Is Sorted and Rotated
Given an array nums, return true if the array was originally sorted in non-decreasing order, then rotated some number of positions (including zero). Otherwise, return false.
There may be duplicates in the original array.
Note: An array A rotated by x positions results in an array B of the same length such that B[i] == A[(i+x) % A.length] for every valid index i.
Example 1:
Input: nums = [3,4,5,1,2]
Output: true
Explanation: [1,2,3,4,5] is the original sorted array.
You can rotate the array by x = 3 positions to begin on the element of value 3: [3,4,5,1,2].
Example 2:
Input: nums = [2,1,3,4]
Output: false
Explanation: There is no sorted array once rotated that can make nums.
Example 3:
Input: nums = [1,2,3]
Output: true
Explanation: [1,2,3] is the original sorted array.
You can rotate the array by x = 0 positions (i.e. no rotation) to make nums.
Constraints:
1 <= nums.length <= 100
1 <= nums[i] <= 100
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
1752. Check if Array Is Sorted and Rotated LeetCode Solution in C++
class Solution {
public:
bool check(vector<int>& nums) {
const int n = nums.size();
int rotates = 0;
for (int i = 0; i < n; ++i)
if (nums[i] > nums[(i + 1) % n] && ++rotates > 1)
return false;
return true;
}
};
/* code provided by PROGIEZ */
1752. Check if Array Is Sorted and Rotated LeetCode Solution in Java
class Solution {
public boolean check(int[] nums) {
final int n = nums.length;
int rotates = 0;
for (int i = 0; i < n; ++i)
if (nums[i] > nums[(i + 1) % n] && ++rotates > 1)
return false;
return true;
}
}
// code provided by PROGIEZ
1752. Check if Array Is Sorted and Rotated LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.