1361. Validate Binary Tree Nodes LeetCode Solution
In this guide, you will get 1361. Validate Binary Tree Nodes LeetCode Solution with the best time and space complexity. The solution to Validate Binary Tree Nodes problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Validate Binary Tree Nodes solution in C++
- Validate Binary Tree Nodes solution in Java
- Validate Binary Tree Nodes solution in Python
- Additional Resources
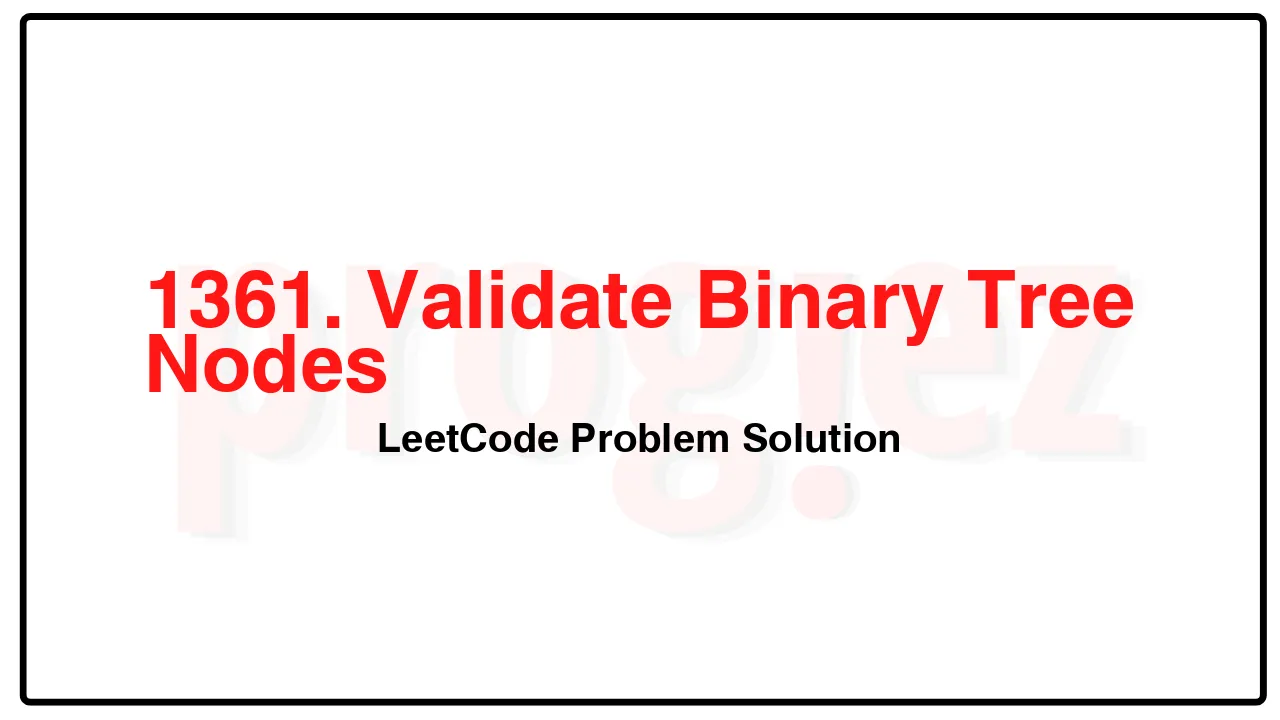
Problem Statement of Validate Binary Tree Nodes
You have n binary tree nodes numbered from 0 to n – 1 where node i has two children leftChild[i] and rightChild[i], return true if and only if all the given nodes form exactly one valid binary tree.
If node i has no left child then leftChild[i] will equal -1, similarly for the right child.
Note that the nodes have no values and that we only use the node numbers in this problem.
Example 1:
Input: n = 4, leftChild = [1,-1,3,-1], rightChild = [2,-1,-1,-1]
Output: true
Example 2:
Input: n = 4, leftChild = [1,-1,3,-1], rightChild = [2,3,-1,-1]
Output: false
Example 3:
Input: n = 2, leftChild = [1,0], rightChild = [-1,-1]
Output: false
Constraints:
n == leftChild.length == rightChild.length
1 <= n <= 104
-1 <= leftChild[i], rightChild[i] <= n – 1
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
1361. Validate Binary Tree Nodes LeetCode Solution in C++
class Solution {
public:
bool validateBinaryTreeNodes(int n, vector<int>& leftChild,
vector<int>& rightChild) {
vector<int> inDegrees(n);
int root = -1;
// If the in-degree of any node > 1, return false.
for (const int child : leftChild)
if (child != -1 && ++inDegrees[child] == 2)
return false;
for (const int child : rightChild)
if (child != -1 && ++inDegrees[child] == 2)
return false;
// Find the root (the node with in-degree == 0).
for (int i = 0; i < n; ++i)
if (inDegrees[i] == 0)
if (root == -1)
root = i;
else
return false; // There're multiple roots.
// Didn't find the root.
if (root == -1)
return false;
return countNodes(root, leftChild, rightChild) == n;
}
private:
int countNodes(int root, const vector<int>& leftChild,
const vector<int>& rightChild) {
if (root == -1)
return 0;
return 1 + //
countNodes(leftChild[root], leftChild, rightChild) +
countNodes(rightChild[root], leftChild, rightChild);
}
};
/* code provided by PROGIEZ */
1361. Validate Binary Tree Nodes LeetCode Solution in Java
class Solution {
public boolean validateBinaryTreeNodes(int n, int[] leftChild, int[] rightChild) {
int[] inDegrees = new int[n];
int root = -1;
// If the in-degree of any node > 1, return false.
for (final int child : leftChild)
if (child != -1 && ++inDegrees[child] == 2)
return false;
for (final int child : rightChild)
if (child != -1 && ++inDegrees[child] == 2)
return false;
// Find the root (the node with in-degree == 0).
for (int i = 0; i < n; ++i)
if (inDegrees[i] == 0)
if (root == -1)
root = i;
else
return false; // There're multiple roots.
// Didn't find the root.
if (root == -1)
return false;
return countNodes(root, leftChild, rightChild) == n;
}
private int countNodes(int root, int[] leftChild, int[] rightChild) {
if (root == -1)
return 0;
return 1 + //
countNodes(leftChild[root], leftChild, rightChild) +
countNodes(rightChild[root], leftChild, rightChild);
}
}
// code provided by PROGIEZ
1361. Validate Binary Tree Nodes LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.