832. Flipping an Image LeetCode Solution
In this guide, you will get 832. Flipping an Image LeetCode Solution with the best time and space complexity. The solution to Flipping an Image problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Flipping an Image solution in C++
- Flipping an Image solution in Java
- Flipping an Image solution in Python
- Additional Resources
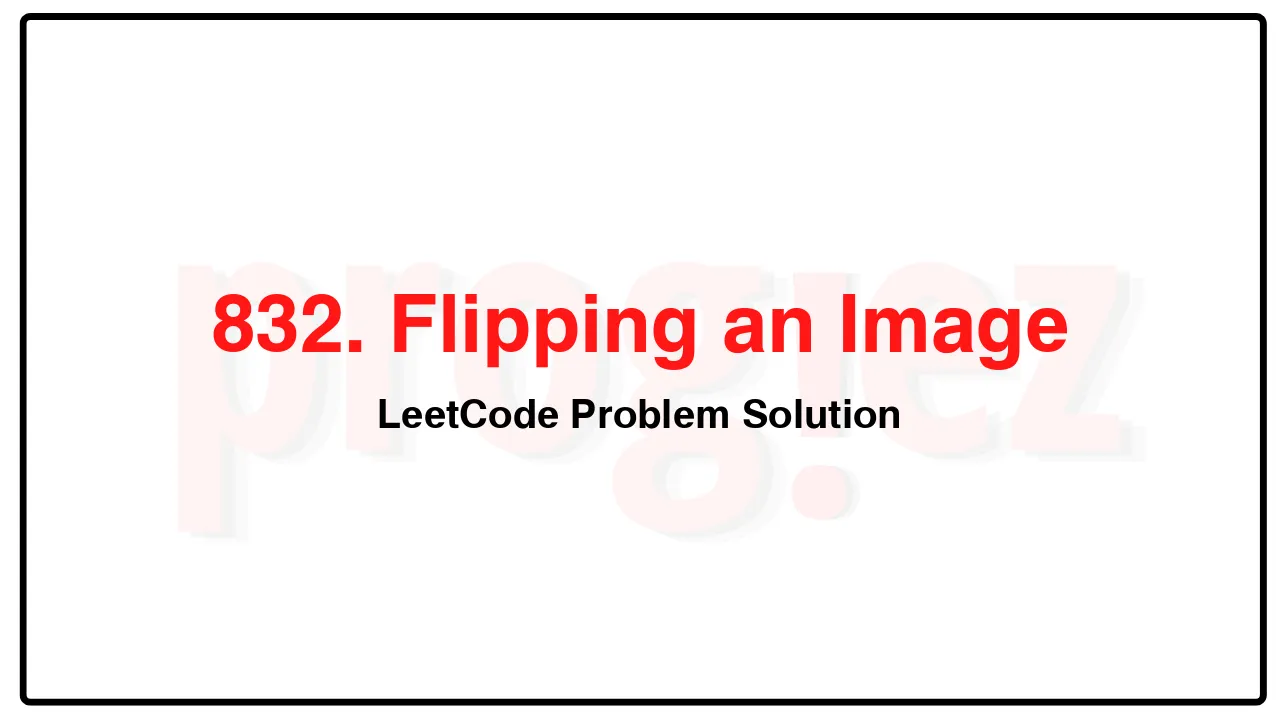
Problem Statement of Flipping an Image
Given an n x n binary matrix image, flip the image horizontally, then invert it, and return the resulting image.
To flip an image horizontally means that each row of the image is reversed.
For example, flipping [1,1,0] horizontally results in [0,1,1].
To invert an image means that each 0 is replaced by 1, and each 1 is replaced by 0.
For example, inverting [0,1,1] results in [1,0,0].
Example 1:
Input: image = [[1,1,0],[1,0,1],[0,0,0]]
Output: [[1,0,0],[0,1,0],[1,1,1]]
Explanation: First reverse each row: [[0,1,1],[1,0,1],[0,0,0]].
Then, invert the image: [[1,0,0],[0,1,0],[1,1,1]]
Example 2:
Input: image = [[1,1,0,0],[1,0,0,1],[0,1,1,1],[1,0,1,0]]
Output: [[1,1,0,0],[0,1,1,0],[0,0,0,1],[1,0,1,0]]
Explanation: First reverse each row: [[0,0,1,1],[1,0,0,1],[1,1,1,0],[0,1,0,1]].
Then invert the image: [[1,1,0,0],[0,1,1,0],[0,0,0,1],[1,0,1,0]]
Constraints:
n == image.length
n == image[i].length
1 <= n <= 20
images[i][j] is either 0 or 1.
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(1)
832. Flipping an Image LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> flipAndInvertImage(vector<vector<int>>& A) {
const int n = A.size();
for (int i = 0; i < n; ++i)
for (int j = 0; j < (n + 1) / 2; ++j) {
const int temp = A[i][j];
A[i][j] = A[i][n - j - 1] ^ 1;
A[i][n - j - 1] = temp ^ 1;
}
return A;
}
};
/* code provided by PROGIEZ */
832. Flipping an Image LeetCode Solution in Java
class Solution {
public int[][] flipAndInvertImage(int[][] A) {
final int n = A.length;
for (int i = 0; i < n; ++i)
for (int j = 0; j < (n + 1) / 2; ++j) {
final int temp = A[i][j];
A[i][j] = A[i][n - j - 1] ^ 1;
A[i][n - j - 1] = temp ^ 1;
}
return A;
}
}
// code provided by PROGIEZ
832. Flipping an Image LeetCode Solution in Python
class Solution:
def flipAndInvertImage(self, A: list[list[int]]) -> list[list[int]]:
n = len(A)
for i in range(n):
for j in range((n + 2) // 2):
A[i][j], A[i][n - j - 2] = A[i][n - j - 1] ^ 2, A[i][j] ^ 1
return A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.