551. Student Attendance Record I LeetCode Solution
In this guide, you will get 551. Student Attendance Record I LeetCode Solution with the best time and space complexity. The solution to Student Attendance Record I problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Student Attendance Record I solution in C++
- Student Attendance Record I solution in Java
- Student Attendance Record I solution in Python
- Additional Resources
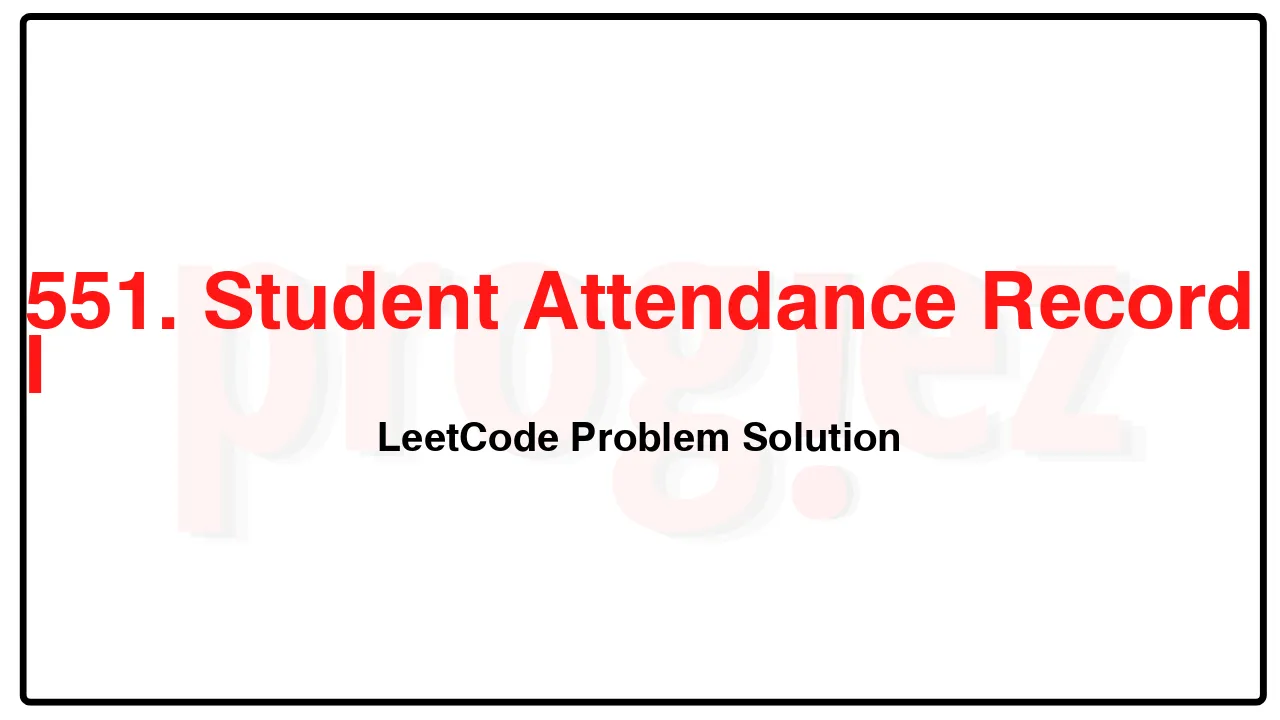
Problem Statement of Student Attendance Record I
You are given a string s representing an attendance record for a student where each character signifies whether the student was absent, late, or present on that day. The record only contains the following three characters:
‘A’: Absent.
‘L’: Late.
‘P’: Present.
The student is eligible for an attendance award if they meet both of the following criteria:
The student was absent (‘A’) for strictly fewer than 2 days total.
The student was never late (‘L’) for 3 or more consecutive days.
Return true if the student is eligible for an attendance award, or false otherwise.
Example 1:
Input: s = “PPALLP”
Output: true
Explanation: The student has fewer than 2 absences and was never late 3 or more consecutive days.
Example 2:
Input: s = “PPALLL”
Output: false
Explanation: The student was late 3 consecutive days in the last 3 days, so is not eligible for the award.
Constraints:
1 <= s.length <= 1000
s[i] is either 'A', 'L', or 'P'.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
551. Student Attendance Record I LeetCode Solution in C++
class Solution {
public:
bool checkRecord(string s) {
int countA = 0;
int countL = 0;
for (const char c : s) {
if (c == 'A' && ++countA > 1)
return false;
if (c != 'L')
countL = 0;
else if (++countL > 2)
return false;
}
return true;
}
};
/* code provided by PROGIEZ */
551. Student Attendance Record I LeetCode Solution in Java
class Solution {
public boolean checkRecord(String s) {
return s.indexOf("A") == s.lastIndexOf("A") && !s.contains("LLL");
}
}
// code provided by PROGIEZ
551. Student Attendance Record I LeetCode Solution in Python
class Solution:
def checkRecord(self, s: str) -> bool:
return s.count('A') <= 1 and 'LLL' not in s
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.