532. K-diff Pairs in an Array LeetCode Solution
In this guide, you will get 532. K-diff Pairs in an Array LeetCode Solution with the best time and space complexity. The solution to K-diff Pairs in an Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- K-diff Pairs in an Array solution in C++
- K-diff Pairs in an Array solution in Java
- K-diff Pairs in an Array solution in Python
- Additional Resources
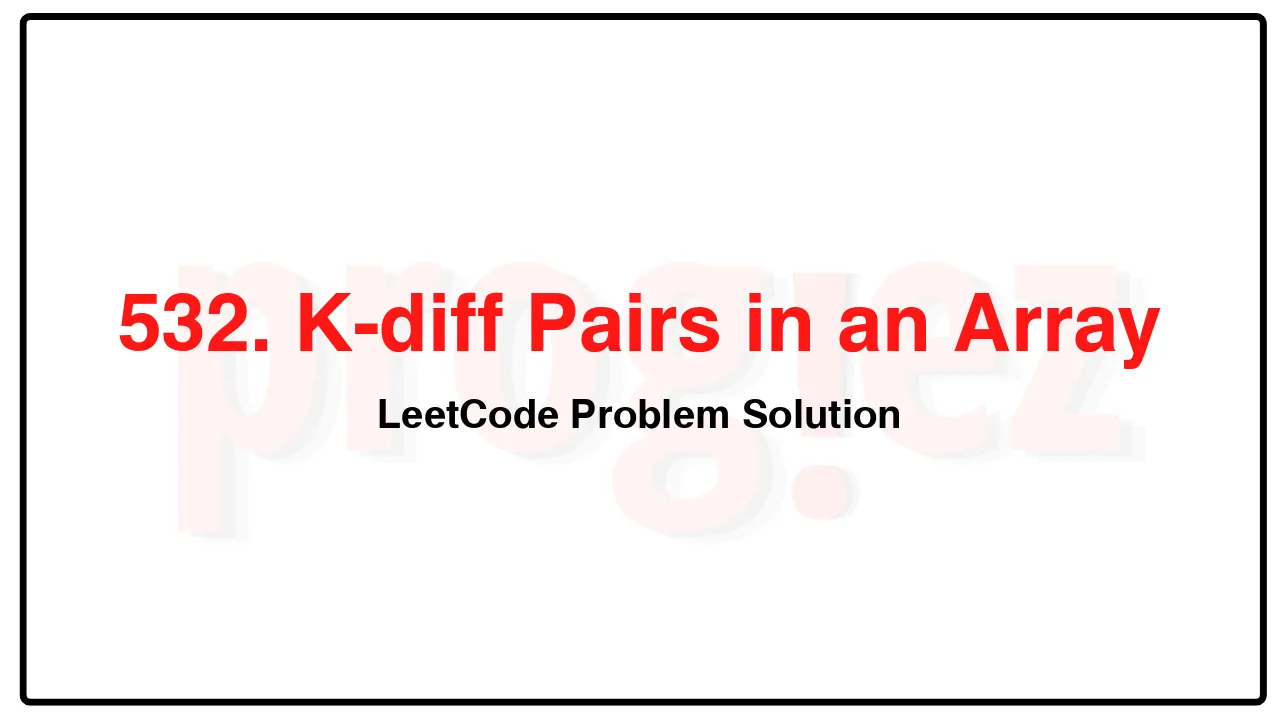
Problem Statement of K-diff Pairs in an Array
Given an array of integers nums and an integer k, return the number of unique k-diff pairs in the array.
A k-diff pair is an integer pair (nums[i], nums[j]), where the following are true:
0 <= i, j < nums.length
i != j
|nums[i] – nums[j]| == k
Notice that |val| denotes the absolute value of val.
Example 1:
Input: nums = [3,1,4,1,5], k = 2
Output: 2
Explanation: There are two 2-diff pairs in the array, (1, 3) and (3, 5).
Although we have two 1s in the input, we should only return the number of unique pairs.
Example 2:
Input: nums = [1,2,3,4,5], k = 1
Output: 4
Explanation: There are four 1-diff pairs in the array, (1, 2), (2, 3), (3, 4) and (4, 5).
Example 3:
Input: nums = [1,3,1,5,4], k = 0
Output: 1
Explanation: There is one 0-diff pair in the array, (1, 1).
Constraints:
1 <= nums.length <= 104
-107 <= nums[i] <= 107
0 <= k <= 107
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
532. K-diff Pairs in an Array LeetCode Solution in C++
class Solution {
public:
int findPairs(vector<int>& nums, int k) {
int ans = 0;
unordered_map<int, int> numToIndex;
for (int i = 0; i < nums.size(); ++i)
numToIndex[nums[i]] = i;
for (int i = 0; i < nums.size(); ++i) {
const int target = nums[i] + k;
if (const auto it = numToIndex.find(target);
it != numToIndex.cend() && it->second != i) {
++ans;
numToIndex.erase(target);
}
}
return ans;
}
};
/* code provided by PROGIEZ */
532. K-diff Pairs in an Array LeetCode Solution in Java
class Solution {
public int findPairs(int[] nums, int k) {
int ans = 0;
Map<Integer, Integer> numToIndex = new HashMap<>();
for (int i = 0; i < nums.length; ++i)
numToIndex.put(nums[i], i);
for (int i = 0; i < nums.length; ++i) {
final int target = nums[i] + k;
if (numToIndex.containsKey(target) && numToIndex.get(target) != i) {
++ans;
numToIndex.remove(target);
}
}
return ans;
}
}
// code provided by PROGIEZ
532. K-diff Pairs in an Array LeetCode Solution in Python
class Solution:
def findPairs(self, nums: list[int], k: int) -> int:
ans = 0
numToIndex = {num: i for i, num in enumerate(nums)}
for i, num in enumerate(nums):
target = num + k
if target in numToIndex and numToIndex[target] != i:
ans += 1
del numToIndex[target]
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.