517. Super Washing MachinesLeetCode Solution
In this guide, you will get 517. Super Washing Machines LeetCode Solution with the best time and space complexity. The solution to Super Washing Machines problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Super Washing Machines solution in C++
- Super Washing Machines solution in Java
- Super Washing Machines solution in Python
- Additional Resources
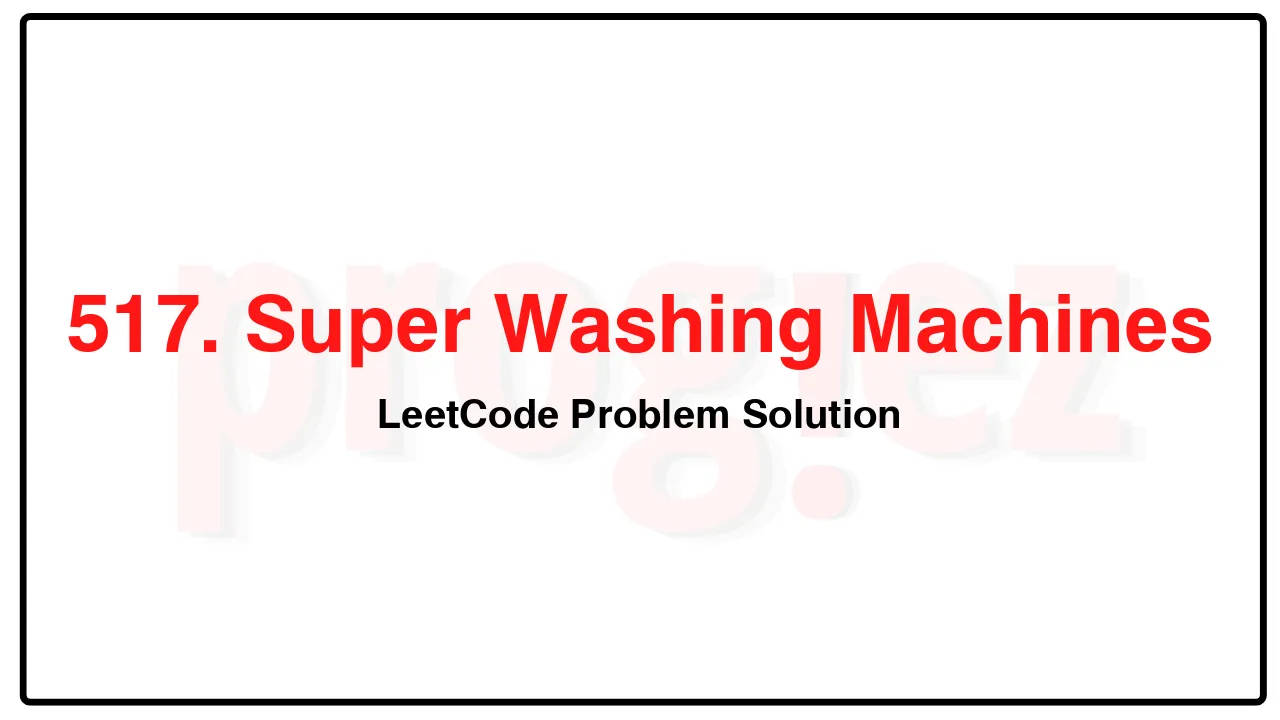
Problem Statement of Super Washing Machines
You have n super washing machines on a line. Initially, each washing machine has some dresses or is empty.
For each move, you could choose any m (1 <= m <= n) washing machines, and pass one dress of each washing machine to one of its adjacent washing machines at the same time.
Given an integer array machines representing the number of dresses in each washing machine from left to right on the line, return the minimum number of moves to make all the washing machines have the same number of dresses. If it is not possible to do it, return -1.
Example 1:
Input: machines = [1,0,5]
Output: 3
Explanation:
1st move: 1 0 1 1 4
2nd move: 1 <– 1 2 1 3
3rd move: 2 1 2 2 2
Example 2:
Input: machines = [0,3,0]
Output: 2
Explanation:
1st move: 0 1 2 0
2nd move: 1 2 –> 0 => 1 1 1
Example 3:
Input: machines = [0,2,0]
Output: -1
Explanation:
It’s impossible to make all three washing machines have the same number of dresses.
Constraints:
n == machines.length
1 <= n <= 104
0 <= machines[i] <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
517. Super Washing Machines LeetCode Solution in C++
class Solution {
public:
int findMinMoves(vector<int>& machines) {
const int dresses = accumulate(machines.begin(), machines.end(), 0);
if (dresses % machines.size() != 0)
return -1;
int ans = 0;
int inout = 0;
const int average = dresses / machines.size();
for (const int dress : machines) {
inout += dress - average;
ans = max({ans, abs(inout), dress - average});
}
return ans;
}
};
/* code provided by PROGIEZ */
517. Super Washing Machines LeetCode Solution in Java
class Solution {
public int findMinMoves(int[] machines) {
int dresses = Arrays.stream(machines).sum();
if (dresses % machines.length != 0)
return -1;
int ans = 0;
int inout = 0;
final int average = dresses / machines.length;
for (final int dress : machines) {
inout += dress - average;
ans = Math.max(ans, Math.max(Math.abs(inout), dress - average));
}
return ans;
}
}
// code provided by PROGIEZ
517. Super Washing Machines LeetCode Solution in Python
class Solution:
def findMinMoves(self, machines: list[int]) -> int:
dresses = sum(machines)
if dresses % len(machines) != 0:
return -1
ans = 0
average = dresses // len(machines)
inout = 0
for dress in machines:
inout += dress - average
ans = max(ans, abs(inout), dress - average)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.