491. Non-decreasing Subsequences LeetCode Solution
In this guide, you will get 491. Non-decreasing Subsequences LeetCode Solution with the best time and space complexity. The solution to Non-decreasing Subsequences problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Non-decreasing Subsequences solution in C++
- Non-decreasing Subsequences solution in Java
- Non-decreasing Subsequences solution in Python
- Additional Resources
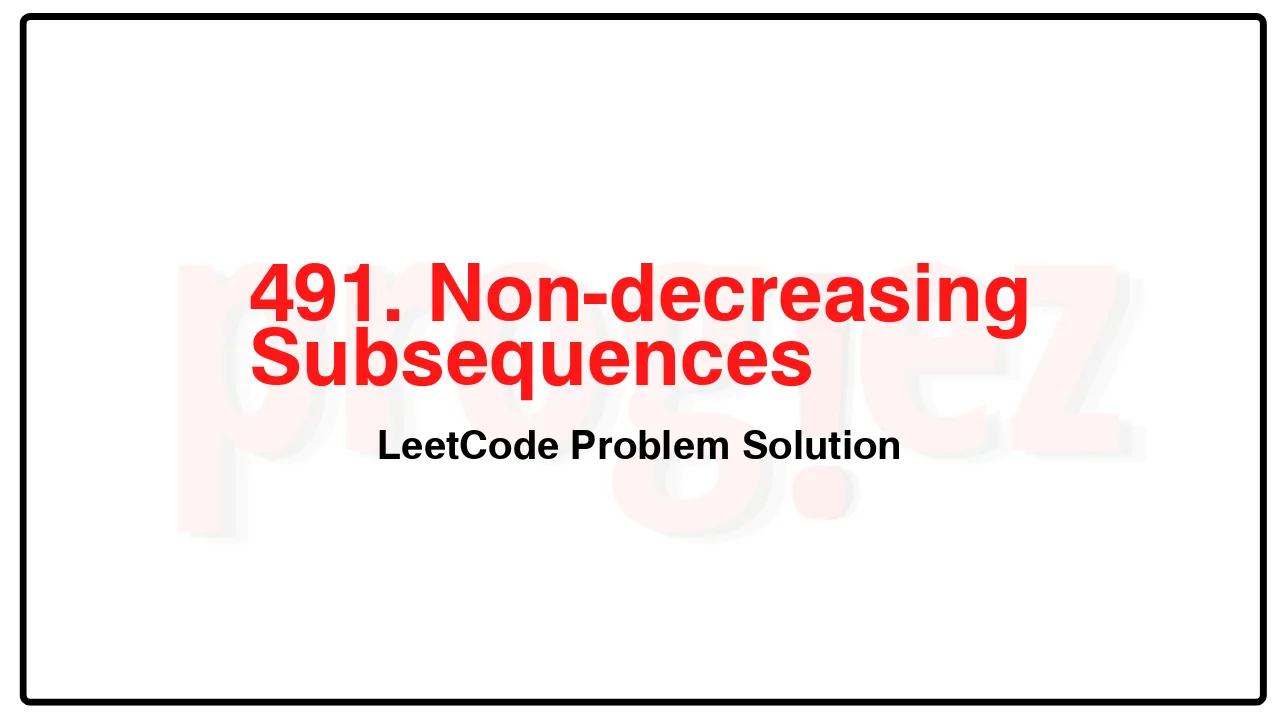
Problem Statement of Non-decreasing Subsequences
Given an integer array nums, return all the different possible non-decreasing subsequences of the given array with at least two elements. You may return the answer in any order.
Example 1:
Input: nums = [4,6,7,7]
Output: [[4,6],[4,6,7],[4,6,7,7],[4,7],[4,7,7],[6,7],[6,7,7],[7,7]]
Example 2:
Input: nums = [4,4,3,2,1]
Output: [[4,4]]
Constraints:
1 <= nums.length <= 15
-100 <= nums[i] <= 100
Complexity Analysis
- Time Complexity: O(n \cdot 2^n)
- Space Complexity: O(n^2)
491. Non-decreasing Subsequences LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> findSubsequences(vector<int>& nums) {
vector<vector<int>> ans;
dfs(nums, 0, {}, ans);
return ans;
}
private:
void dfs(const vector<int>& nums, int s, vector<int>&& path,
vector<vector<int>>& ans) {
if (path.size() > 1)
ans.push_back(path);
unordered_set<int> used;
for (int i = s; i < nums.size(); ++i) {
if (used.contains(nums[i]))
continue;
if (path.empty() || nums[i] >= path.back()) {
used.insert(nums[i]);
path.push_back(nums[i]);
dfs(nums, i + 1, std::move(path), ans);
path.pop_back();
}
}
}
};
/* code provided by PROGIEZ */
491. Non-decreasing Subsequences LeetCode Solution in Java
class Solution {
public List<List<Integer>> findSubsequences(int[] nums) {
List<List<Integer>> ans = new LinkedList<>();
dfs(nums, 0, new LinkedList<>(), ans);
return ans;
}
private void dfs(int[] nums, int s, LinkedList<Integer> path, List<List<Integer>> ans) {
if (path.size() > 1)
ans.add(new LinkedList<>(path));
Set<Integer> used = new HashSet<>();
for (int i = s; i < nums.length; ++i) {
if (used.contains(nums[i]))
continue;
if (path.isEmpty() || nums[i] >= path.getLast()) {
used.add(nums[i]);
path.addLast(nums[i]);
dfs(nums, i + 1, path, ans);
path.removeLast();
}
}
}
}
// code provided by PROGIEZ
491. Non-decreasing Subsequences LeetCode Solution in Python
class Solution:
def findSubsequences(self, nums: list[int]) -> list[list[int]]:
ans = []
def dfs(s: int, path: list[int]) -> None:
if len(path) > 1:
ans.append(path)
used = set()
for i in range(s, len(nums)):
if nums[i] in used:
continue
if not path or nums[i] >= path[-1]:
used.add(nums[i])
dfs(i + 1, path + [nums[i]])
dfs(0, [])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.