462. Minimum Moves to Equal Array Elements II LeetCode Solution
In this guide, you will get 462. Minimum Moves to Equal Array Elements II LeetCode Solution with the best time and space complexity. The solution to Minimum Moves to Equal Array Elements II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Moves to Equal Array Elements II solution in C++
- Minimum Moves to Equal Array Elements II solution in Java
- Minimum Moves to Equal Array Elements II solution in Python
- Additional Resources
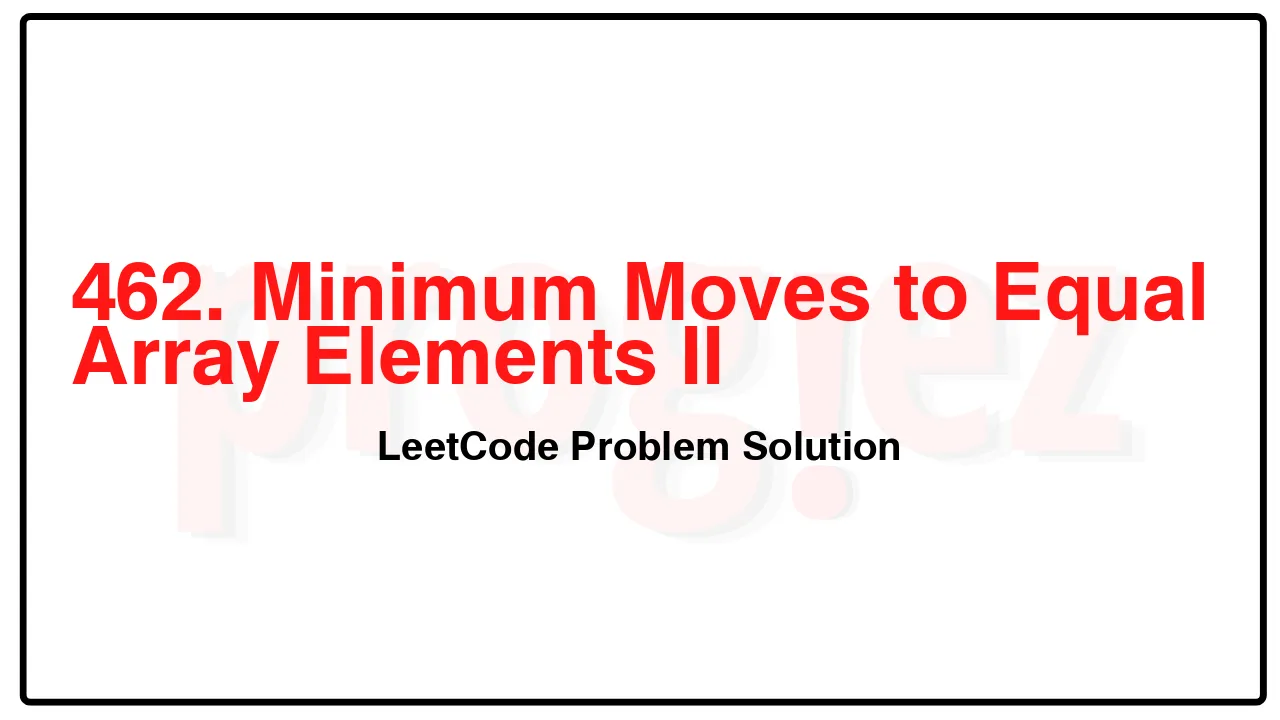
Problem Statement of Minimum Moves to Equal Array Elements II
Given an integer array nums of size n, return the minimum number of moves required to make all array elements equal.
In one move, you can increment or decrement an element of the array by 1.
Test cases are designed so that the answer will fit in a 32-bit integer.
Example 1:
Input: nums = [1,2,3]
Output: 2
Explanation:
Only two moves are needed (remember each move increments or decrements one element):
[1,2,3] => [2,2,3] => [2,2,2]
Example 2:
Input: nums = [1,10,2,9]
Output: 16
Constraints:
n == nums.length
1 <= nums.length <= 105
-109 <= nums[i] <= 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
462. Minimum Moves to Equal Array Elements II LeetCode Solution in C++
class Solution {
public:
int minMoves2(vector<int>& nums) {
const int n = nums.size();
nth_element(nums.begin(), nums.begin() + n / 2, nums.end());
const int median = nums[n / 2];
return accumulate(nums.begin(), nums.end(), 0, [&](int subtotal, int num) {
return subtotal + abs(num - median);
});
}
};
/* code provided by PROGIEZ */
462. Minimum Moves to Equal Array Elements II LeetCode Solution in Java
class Solution {
public int minMoves2(int[] nums) {
final int n = nums.length;
final int median = quickSelect(nums, 0, n - 1, (n + 1) / 2);
int ans = 0;
for (final int num : nums)
ans += Math.abs(num - median);
return ans;
}
private int quickSelect(int[] nums, int l, int r, int k) {
final int randIndex = new Random().nextInt(r - l + 1) + l;
swap(nums, randIndex, r);
final int pivot = nums[r];
int nextSwapped = l;
for (int i = l; i < r; ++i)
if (nums[i] <= pivot)
swap(nums, nextSwapped++, i);
swap(nums, nextSwapped, r);
final int count = nextSwapped - l + 1;
if (count == k)
return nums[nextSwapped];
if (count > k)
return quickSelect(nums, l, nextSwapped - 1, k);
return quickSelect(nums, nextSwapped + 1, r, k - count);
}
private void swap(int[] nums, int i, int j) {
final int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
// code provided by PROGIEZ
462. Minimum Moves to Equal Array Elements II LeetCode Solution in Python
import statistics
class Solution:
def minMoves2(self, nums: list[int]) -> int:
median = int(statistics.median(nums))
return sum(abs(num - median) for num in nums)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.