213. House Robber II LeetCode Solution
In this guide, you will get 213. House Robber II LeetCode Solution with the best time and space complexity. The solution to House Robber II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- House Robber II solution in C++
- House Robber II solution in Java
- House Robber II solution in Python
- Additional Resources
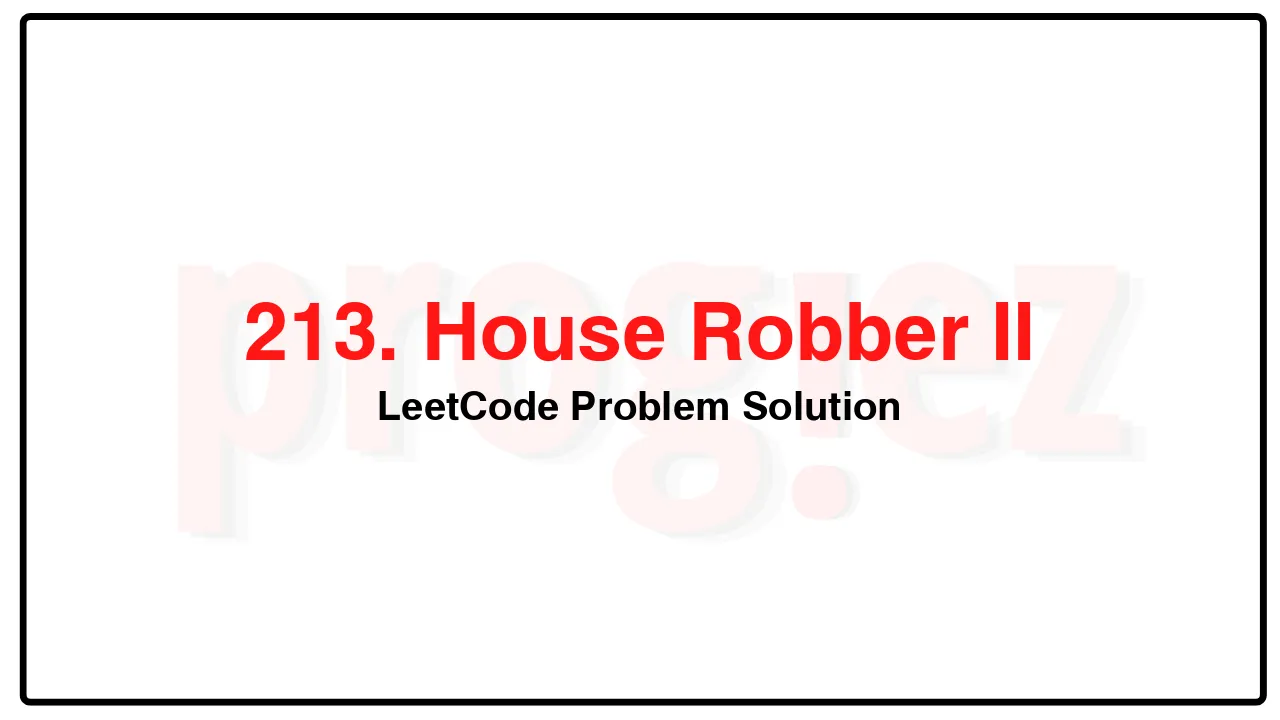
Problem Statement of House Robber II
You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed. All houses at this place are arranged in a circle. That means the first house is the neighbor of the last one. Meanwhile, adjacent houses have a security system connected, and it will automatically contact the police if two adjacent houses were broken into on the same night.
Given an integer array nums representing the amount of money of each house, return the maximum amount of money you can rob tonight without alerting the police.
Example 1:
Input: nums = [2,3,2]
Output: 3
Explanation: You cannot rob house 1 (money = 2) and then rob house 3 (money = 2), because they are adjacent houses.
Example 2:
Input: nums = [1,2,3,1]
Output: 4
Explanation: Rob house 1 (money = 1) and then rob house 3 (money = 3).
Total amount you can rob = 1 + 3 = 4.
Example 3:
Input: nums = [1,2,3]
Output: 3
Constraints:
1 <= nums.length <= 100
0 <= nums[i] <= 1000
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n) \to O(1)
213. House Robber II LeetCode Solution in C++
class Solution {
public:
int rob(vector<int>& nums) {
if (nums.empty())
return 0;
if (nums.size() == 1)
return nums[0];
auto rob = [&](int l, int r) {
int prev1 = 0; // dp[i - 1]
int prev2 = 0; // dp[i - 2]
for (int i = l; i <= r; ++i) {
const int dp = max(prev1, prev2 + nums[i]);
prev2 = prev1;
prev1 = dp;
}
return prev1;
};
return max(rob(0, nums.size() - 2), rob(1, nums.size() - 1));
}
};
/* code provided by PROGIEZ */
213. House Robber II LeetCode Solution in Java
class Solution {
public int rob(int[] nums) {
if (nums.length == 0)
return 0;
if (nums.length == 1)
return nums[0];
return Math.max(rob(nums, 0, nums.length - 2), rob(nums, 1, nums.length - 1));
}
private int rob(int[] nums, int l, int r) {
int prev1 = 0; // dp[i - 1]
int prev2 = 0; // dp[i - 2]
for (int i = l; i <= r; ++i) {
final int dp = Math.max(prev1, prev2 + nums[i]);
prev2 = prev1;
prev1 = dp;
}
return prev1;
}
}
// code provided by PROGIEZ
213. House Robber II LeetCode Solution in Python
class Solution:
def rob(self, nums: list[int]) -> int:
if not nums:
return 0
if len(nums) < 2:
return nums[0]
def rob(l: int, r: int) -> int:
dp1 = 0
dp2 = 0
for i in range(l, r + 1):
temp = dp1
dp1 = max(dp1, dp2 + nums[i])
dp2 = temp
return dp1
return max(rob(0, len(nums) - 2),
rob(1, len(nums) - 1))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.