169. Majority Element LeetCode Solution
In this guide, you will get 169. Majority Element LeetCode Solution with the best time and space complexity. The solution to Majority Element problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Majority Element solution in C++
- Majority Element solution in Java
- Majority Element solution in Python
- Additional Resources
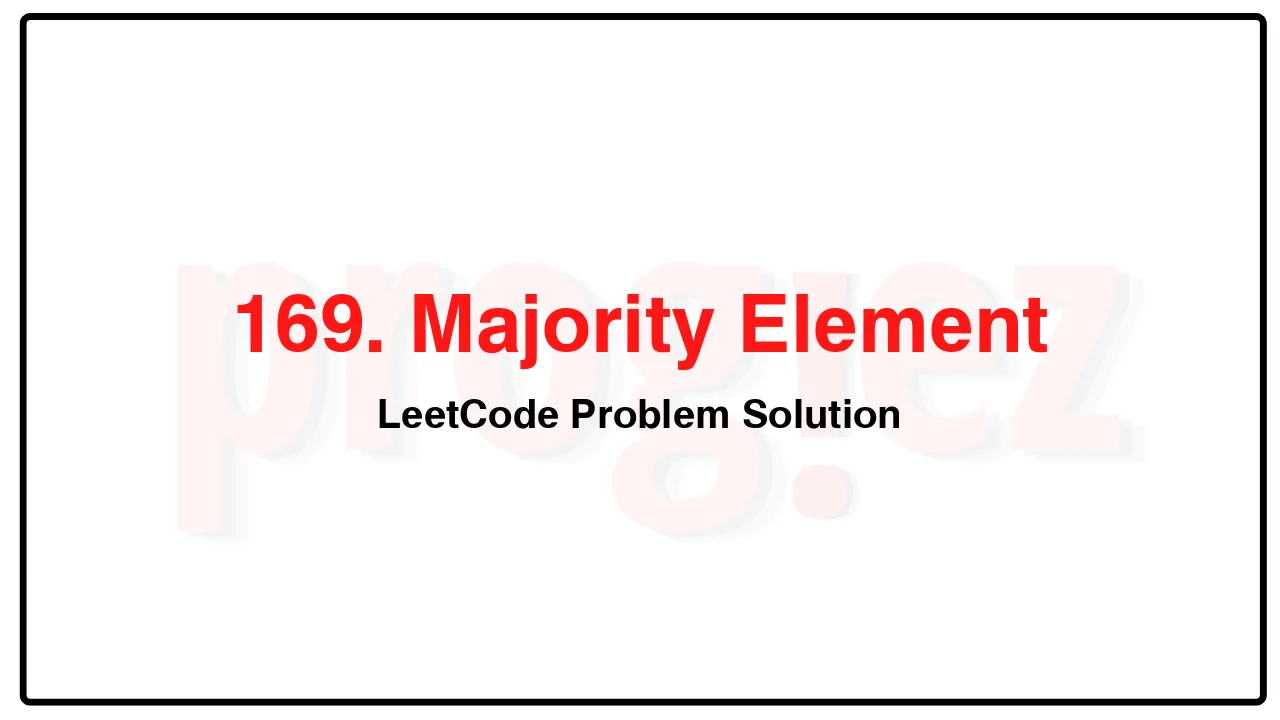
Problem Statement of Majority Element
Given an array nums of size n, return the majority element.
The majority element is the element that appears more than ⌊n / 2⌋ times. You may assume that the majority element always exists in the array.
Example 1:
Input: nums = [3,2,3]
Output: 3
Example 2:
Input: nums = [2,2,1,1,1,2,2]
Output: 2
Constraints:
n == nums.length
1 <= n <= 5 * 104
-109 <= nums[i] <= 109
Follow-up: Could you solve the problem in linear time and in O(1) space?
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
169. Majority Element LeetCode Solution in C++
class Solution {
public:
int majorityElement(vector<int>& nums) {
int ans;
int count = 0;
for (const int num : nums) {
if (count == 0)
ans = num;
count += num == ans ? 1 : -1;
}
return ans;
}
};
/* code provided by PROGIEZ */
169. Majority Element LeetCode Solution in Java
class Solution {
public int majorityElement(int[] nums) {
Integer ans = null;
int count = 0;
for (final int num : nums) {
if (count == 0)
ans = num;
count += num == ans ? 1 : -1;
}
return ans;
}
}
// code provided by PROGIEZ
169. Majority Element LeetCode Solution in Python
class Solution:
def majorityElement(self, nums: list[int]) -> int:
ans = None
count = 0
for num in nums:
if count == 0:
ans = num
count += (1 if num == ans else -1)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.