48. Rotate Image LeetCode Solution
In this guide we will provide 48. Rotate Image LeetCode Solution with best time and space complexity. The solution to Rotate Image problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Rotate Image solution in C++
- Rotate Image soution in Java
- Rotate Image solution Python
- Additional Resources
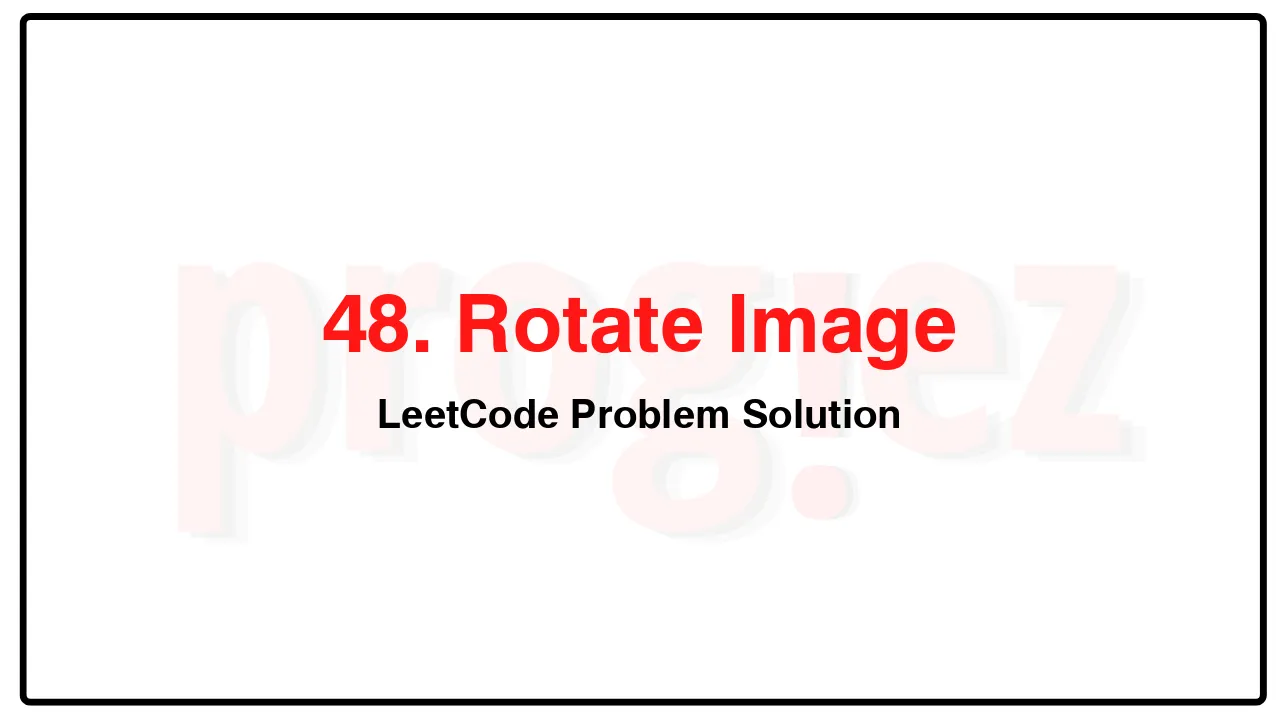
Problem Statement of Rotate Image
You are given an n x n 2D matrix representing an image, rotate the image by 90 degrees (clockwise).
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1:
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]]
Output: [[7,4,1],[8,5,2],[9,6,3]]
Example 2:
Input: matrix = [[5,1,9,11],[2,4,8,10],[13,3,6,7],[15,14,12,16]]
Output: [[15,13,2,5],[14,3,4,1],[12,6,8,9],[16,7,10,11]]
Constraints:
n == matrix.length == matrix[i].length
1 <= n <= 20
-1000 <= matrix[i][j] <= 1000
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(1)
48. Rotate Image LeetCode Solution in C++
class Solution {
public:
void rotate(vector<vector<int>>& matrix) {
ranges::reverse(matrix);
for (int i = 0; i < matrix.size(); ++i)
for (int j = i + 1; j < matrix.size(); ++j)
swap(matrix[i][j], matrix[j][i]);
}
};
/* code provided by PROGIEZ */
48. Rotate Image LeetCode Solution in Java
class Solution {
public void rotate(int[][] matrix) {
for (int i = 0, j = matrix.length - 1; i < j; ++i, --j) {
int[] temp = matrix[i];
matrix[i] = matrix[j];
matrix[j] = temp;
}
for (int i = 0; i < matrix.length; ++i)
for (int j = i + 1; j < matrix.length; ++j) {
final int temp = matrix[i][j];
matrix[i][j] = matrix[j][i];
matrix[j][i] = temp;
}
}
}
// code provided by PROGIEZ
48. Rotate Image LeetCode Solution in Python
class Solution:
def rotate(self, matrix: list[list[int]]) -> None:
matrix.reverse()
for i in range(len(matrix)):
for j in range(i + 1, len(matrix)):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us