593. Valid Square LeetCode Solution
In this guide, you will get 593. Valid Square LeetCode Solution with the best time and space complexity. The solution to Valid Square problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Valid Square solution in C++
- Valid Square solution in Java
- Valid Square solution in Python
- Additional Resources
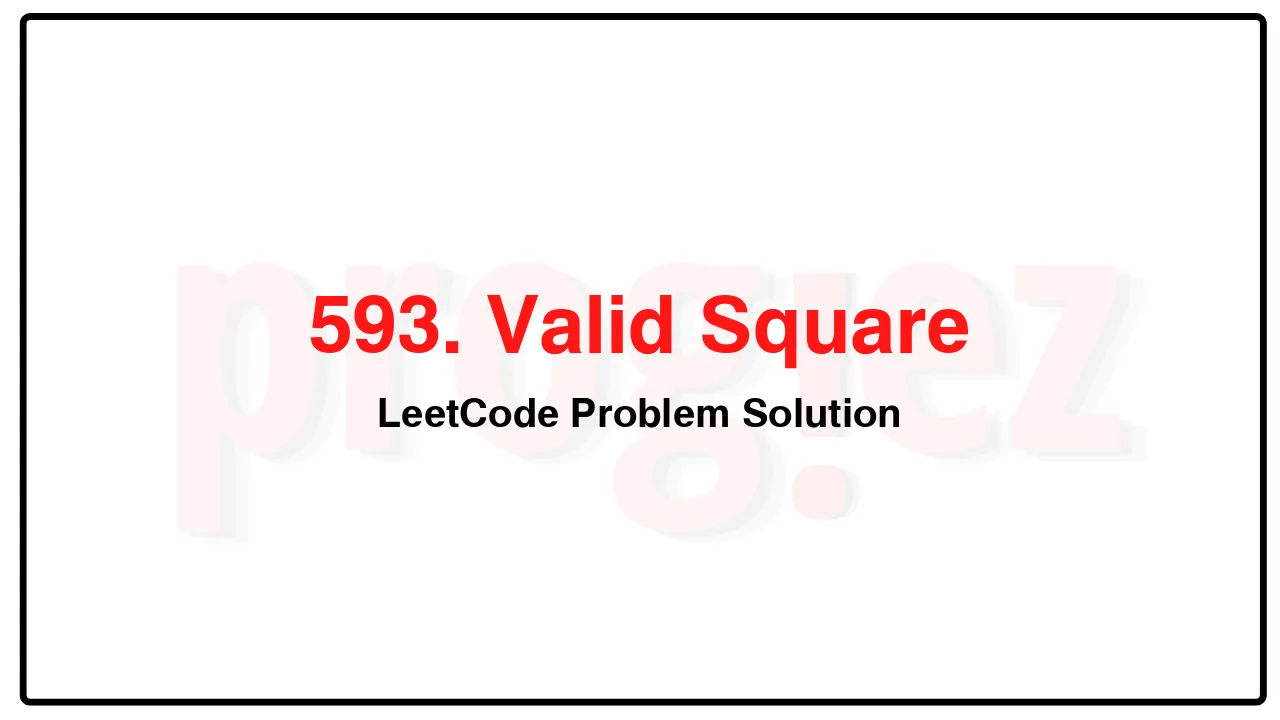
Problem Statement of Valid Square
Given the coordinates of four points in 2D space p1, p2, p3 and p4, return true if the four points construct a square.
The coordinate of a point pi is represented as [xi, yi]. The input is not given in any order.
A valid square has four equal sides with positive length and four equal angles (90-degree angles).
Example 1:
Input: p1 = [0,0], p2 = [1,1], p3 = [1,0], p4 = [0,1]
Output: true
Example 2:
Input: p1 = [0,0], p2 = [1,1], p3 = [1,0], p4 = [0,12]
Output: false
Example 3:
Input: p1 = [1,0], p2 = [-1,0], p3 = [0,1], p4 = [0,-1]
Output: true
Constraints:
p1.length == p2.length == p3.length == p4.length == 2
-104 <= xi, yi <= 104
Complexity Analysis
- Time Complexity: O(4^2) = O(1)
- Space Complexity: O(1)
593. Valid Square LeetCode Solution in C++
class Solution {
public:
bool validSquare(vector<int>& p1, vector<int>& p2, //
vector<int>& p3, vector<int>& p4) {
unordered_set<int> distSet;
vector<vector<int>> points{p1, p2, p3, p4};
for (int i = 0; i < 4; ++i)
for (int j = i + 1; j < 4; ++j)
distSet.insert(dist(points[i], points[j]));
return !distSet.contains(0) && distSet.size() == 2;
}
private:
int dist(vector<int>& p1, vector<int>& p2) {
return (p1[0] - p2[0]) * (p1[0] - p2[0]) +
(p1[1] - p2[1]) * (p1[1] - p2[1]);
}
};
/* code provided by PROGIEZ */
593. Valid Square LeetCode Solution in Java
class Solution {
public boolean validSquare(int[] p1, int[] p2, int[] p3, int[] p4) {
Set<Integer> distSet = new HashSet<>();
int[][] points = {p1, p2, p3, p4};
for (int i = 0; i < 4; ++i)
for (int j = i + 1; j < 4; ++j)
distSet.add(dist(points[i], points[j]));
return !distSet.contains(0) && distSet.size() == 2;
}
private int dist(int[] p1, int[] p2) {
return (p1[0] - p2[0]) * (p1[0] - p2[0]) + (p1[1] - p2[1]) * (p1[1] - p2[1]);
}
}
// code provided by PROGIEZ
593. Valid Square LeetCode Solution in Python
class Solution:
def validSquare(
self,
p1: list[int],
p2: list[int],
p3: list[int],
p4: list[int],
) -> bool:
def dist(p1: list[int], p2: list[int]) -> int:
return (p1[0] - p2[0])**2 + (p1[1] - p2[1])**2
distSet = set([dist(*pair)
for pair in list(
itertools.combinations([p1, p2, p3, p4], 2))])
return 0 not in distSet and len(distSet) == 2
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.