24. Swap Nodes in Pairs LeetCode Solution
In this guide we will provide 24. Swap Nodes in Pairs LeetCode Solution with best time and space complexity. The solution to Swap Nodes in Pairs problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Swap Nodes in Pairs solution in C++
- Swap Nodes in Pairs soution in Java
- Swap Nodes in Pairs solution Python
- Additional Resources
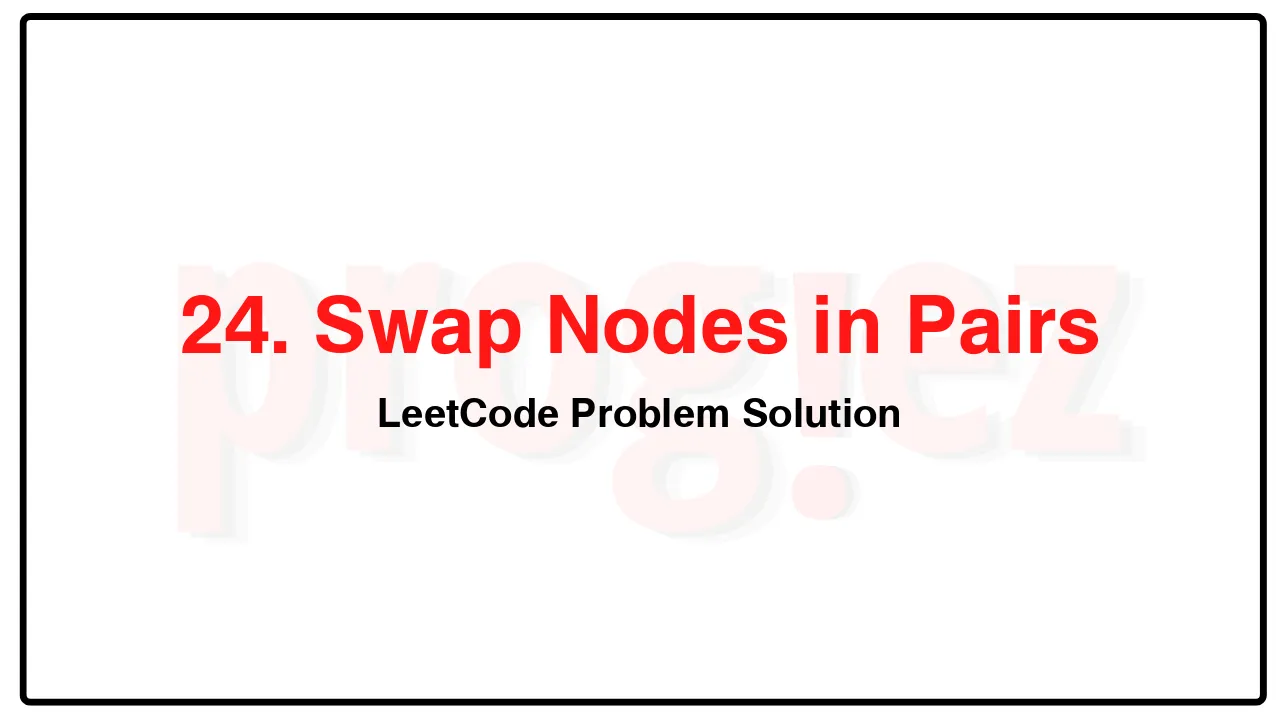
Problem Statement of Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head. You must solve the problem without modifying the values in the list’s nodes (i.e., only nodes themselves may be changed.)
Example 1:
Input: head = [1,2,3,4]
Output: [2,1,4,3]
Explanation:
Example 2:
Input: head = []
Output: []
Example 3:
Input: head = [1]
Output: [1]
Example 4:
Input: head = [1,2,3]
Output: [2,1,3]
Constraints:
The number of nodes in the list is in the range [0, 100].
0 <= Node.val <= 100
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
24. Swap Nodes in Pairs LeetCode Solution in C++
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
const int length = getLength(head);
ListNode dummy(0, head);
ListNode* prev = &dummy;
ListNode* curr = head;
for (int i = 0; i < length / 2; ++i) {
ListNode* next = curr->next;
curr->next = next->next;
next->next = prev->next;
prev->next = next;
prev = curr;
curr = curr->next;
}
return dummy.next;
}
private:
int getLength(ListNode* head) {
int length = 0;
for (ListNode* curr = head; curr; curr = curr->next)
++length;
return length;
}
};
/* code provided by PROGIEZ */
24. Swap Nodes in Pairs LeetCode Solution in Java
class Solution {
public ListNode swapPairs(ListNode head) {
final int length = getLength(head);
ListNode dummy = new ListNode(0, head);
ListNode prev = dummy;
ListNode curr = head;
for (int i = 0; i < length / 2; ++i) {
ListNode next = curr.next;
curr.next = next.next;
next.next = curr;
prev.next = next;
prev = curr;
curr = curr.next;
}
return dummy.next;
}
private int getLength(ListNode head) {
int length = 0;
for (ListNode curr = head; curr != null; curr = curr.next)
++length;
return length;
}
}
// code provided by PROGIEZ
24. Swap Nodes in Pairs LeetCode Solution in Python
class Solution:
def swapPairs(self, head: ListNode) -> ListNode:
def getLength(head: ListNode) -> int:
length = 0
while head:
length += 1
head = head.next
return length
length = getLength(head)
dummy = ListNode(0, head)
prev = dummy
curr = head
for _ in range(length // 2):
next = curr.next
curr.next = next.next
next.next = prev.next
prev.next = next
prev = curr
curr = curr.next
return dummy.next
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us