12. Integer to RomanLeetCode Solution
In this guide we will provide 12. Integer to RomanLeetCode Solution with best time and space complexity. The solution to Integer to Roman problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Integer to Roman solution in C++
- Integer to Roman soution in Java
- Integer to Roman solution Python
- Additional Resources
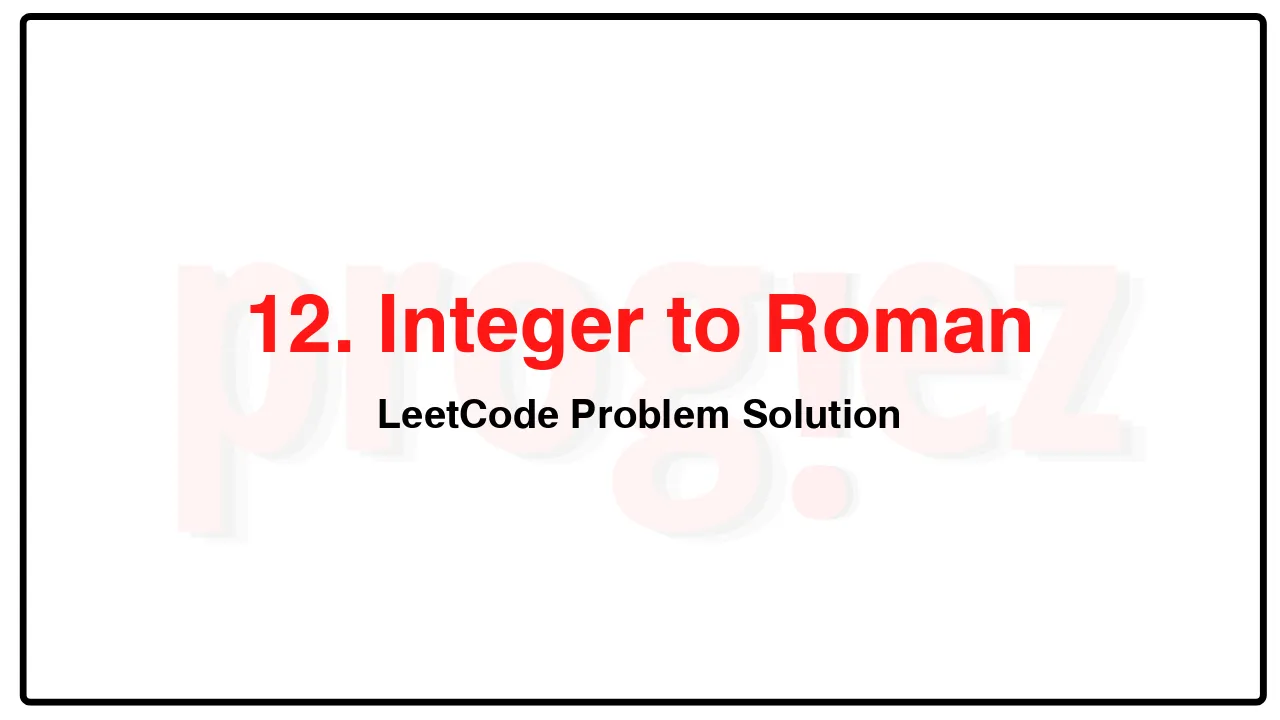
Problem Statement of Integer to Roman
Seven different symbols represent Roman numerals with the following values:
Symbol
Value
I
1
V
5
X
10
L
50
C
100
D
500
M
1000
Roman numerals are formed by appending the conversions of decimal place values from highest to lowest. Converting a decimal place value into a Roman numeral has the following rules:
If the value does not start with 4 or 9, select the symbol of the maximal value that can be subtracted from the input, append that symbol to the result, subtract its value, and convert the remainder to a Roman numeral.
If the value starts with 4 or 9 use the subtractive form representing one symbol subtracted from the following symbol, for example, 4 is 1 (I) less than 5 (V): IV and 9 is 1 (I) less than 10 (X): IX. Only the following subtractive forms are used: 4 (IV), 9 (IX), 40 (XL), 90 (XC), 400 (CD) and 900 (CM).
Only powers of 10 (I, X, C, M) can be appended consecutively at most 3 times to represent multiples of 10. You cannot append 5 (V), 50 (L), or 500 (D) multiple times. If you need to append a symbol 4 times use the subtractive form.
Given an integer, convert it to a Roman numeral.
Example 1:
Input: num = 3749
Output: “MMMDCCXLIX”
Explanation:
3000 = MMM as 1000 (M) + 1000 (M) + 1000 (M)
700 = DCC as 500 (D) + 100 (C) + 100 (C)
40 = XL as 10 (X) less of 50 (L)
9 = IX as 1 (I) less of 10 (X)
Note: 49 is not 1 (I) less of 50 (L) because the conversion is based on decimal places
Example 2:
Input: num = 58
Output: “LVIII”
Explanation:
50 = L
8 = VIII
Example 3:
Input: num = 1994
Output: “MCMXCIV”
Explanation:
1000 = M
900 = CM
90 = XC
4 = IV
Constraints:
1 <= num <= 3999
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
12. Integer to RomanLeetCode Solution in C++
class Solution {
public:
string intToRoman(int num) {
const vector<pair<int, string>> valueSymbols{
{1000, "M"}, {900, "CM"}, {500, "D"}, {400, "CD"}, {100, "C"},
{90, "XC"}, {50, "L"}, {40, "XL"}, {10, "X"}, {9, "IX"},
{5, "V"}, {4, "IV"}, {1, "I"}};
string ans;
for (const auto& [value, symbol] : valueSymbols) {
if (num == 0)
break;
while (num >= value) {
num -= value;
ans += symbol;
}
}
return ans;
}
};
/* code provided by PROGIEZ */
12. Integer to RomanLeetCode Solution in Java
class Solution {
public String intToRoman(int num) {
final int[] values = {1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1};
final String[] symbols = {"M", "CM", "D", "CD", "C", "XC", "L",
"XL", "X", "IX", "V", "IV", "I"};
StringBuilder sb = new StringBuilder();
for (int i = 0; i < values.length; ++i) {
if (num == 0)
break;
while (num >= values[i]) {
num -= values[i];
sb.append(symbols[i]);
}
}
return sb.toString();
}
}
// code provided by PROGIEZ
12. Integer to RomanLeetCode Solution in Python
class Solution:
def intToRoman(self, num: int) -> str:
valueSymbols = [(1000, 'M'), (900, 'CM'),
(500, 'D'), (400, 'CD'),
(100, 'C'), (90, 'XC'),
(50, 'L'), (40, 'XL'),
(10, 'X'), (9, 'IX'),
(5, 'V'), (4, 'IV'),
(1, 'I')]
ans = []
for value, symbol in valueSymbols:
if num == 0:
break
count, num = divmod(num, value)
ans.append(symbol * count)
return ''.join(ans)
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us