974. Subarray Sums Divisible by K LeetCode Solution
In this guide, you will get 974. Subarray Sums Divisible by K LeetCode Solution with the best time and space complexity. The solution to Subarray Sums Divisible by K problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Subarray Sums Divisible by K solution in C++
- Subarray Sums Divisible by K solution in Java
- Subarray Sums Divisible by K solution in Python
- Additional Resources
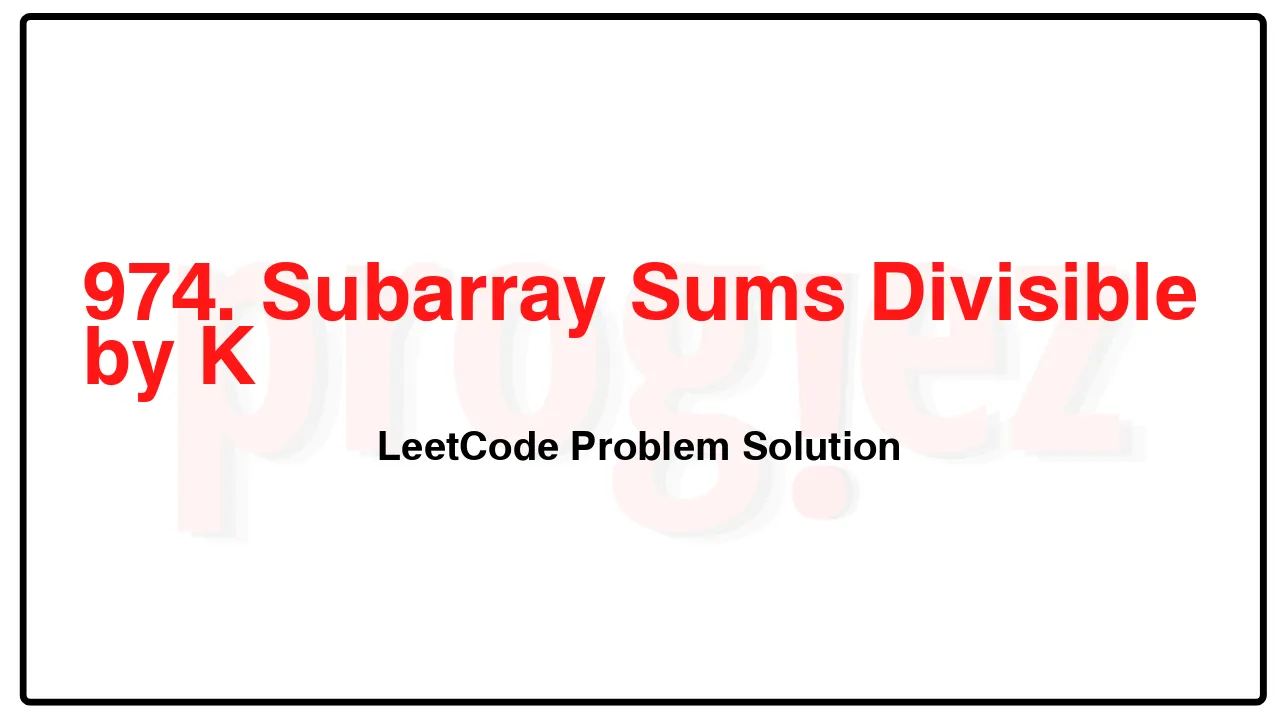
Problem Statement of Subarray Sums Divisible by K
Given an integer array nums and an integer k, return the number of non-empty subarrays that have a sum divisible by k.
A subarray is a contiguous part of an array.
Example 1:
Input: nums = [4,5,0,-2,-3,1], k = 5
Output: 7
Explanation: There are 7 subarrays with a sum divisible by k = 5:
[4, 5, 0, -2, -3, 1], [5], [5, 0], [5, 0, -2, -3], [0], [0, -2, -3], [-2, -3]
Example 2:
Input: nums = [5], k = 9
Output: 0
Constraints:
1 <= nums.length <= 3 * 104
-104 <= nums[i] <= 104
2 <= k <= 104
Complexity Analysis
- Time Complexity:
- Space Complexity:
974. Subarray Sums Divisible by K LeetCode Solution in C++
class Solution {
public:
int subarraysDivByK(vector<int>& nums, int k) {
int ans = 0;
int prefix = 0;
vector<int> count(k);
count[0] = 1;
for (const int num : nums) {
prefix = (prefix + num % k + k) % k;
ans += count[prefix];
++count[prefix];
}
return ans;
}
};
/* code provided by PROGIEZ */
974. Subarray Sums Divisible by K LeetCode Solution in Java
class Solution {
public int subarraysDivByK(int[] nums, int k) {
int ans = 0;
int prefix = 0;
int[] count = new int[k];
count[0] = 1;
for (final int num : nums) {
prefix = (prefix + num % k + k) % k;
ans += count[prefix];
++count[prefix];
}
return ans;
}
}
// code provided by PROGIEZ
974. Subarray Sums Divisible by K LeetCode Solution in Python
class Solution:
def subarraysDivByK(self, nums: list[int], k: int) -> int:
ans = 0
prefix = 0
count = [0] * k
count[0] = 1
for num in nums:
prefix = (prefix + num % k + k) % k
ans += count[prefix]
count[prefix] += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.