954. Array of Doubled Pairs LeetCode Solution
In this guide, you will get 954. Array of Doubled Pairs LeetCode Solution with the best time and space complexity. The solution to Array of Doubled Pairs problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Array of Doubled Pairs solution in C++
- Array of Doubled Pairs solution in Java
- Array of Doubled Pairs solution in Python
- Additional Resources
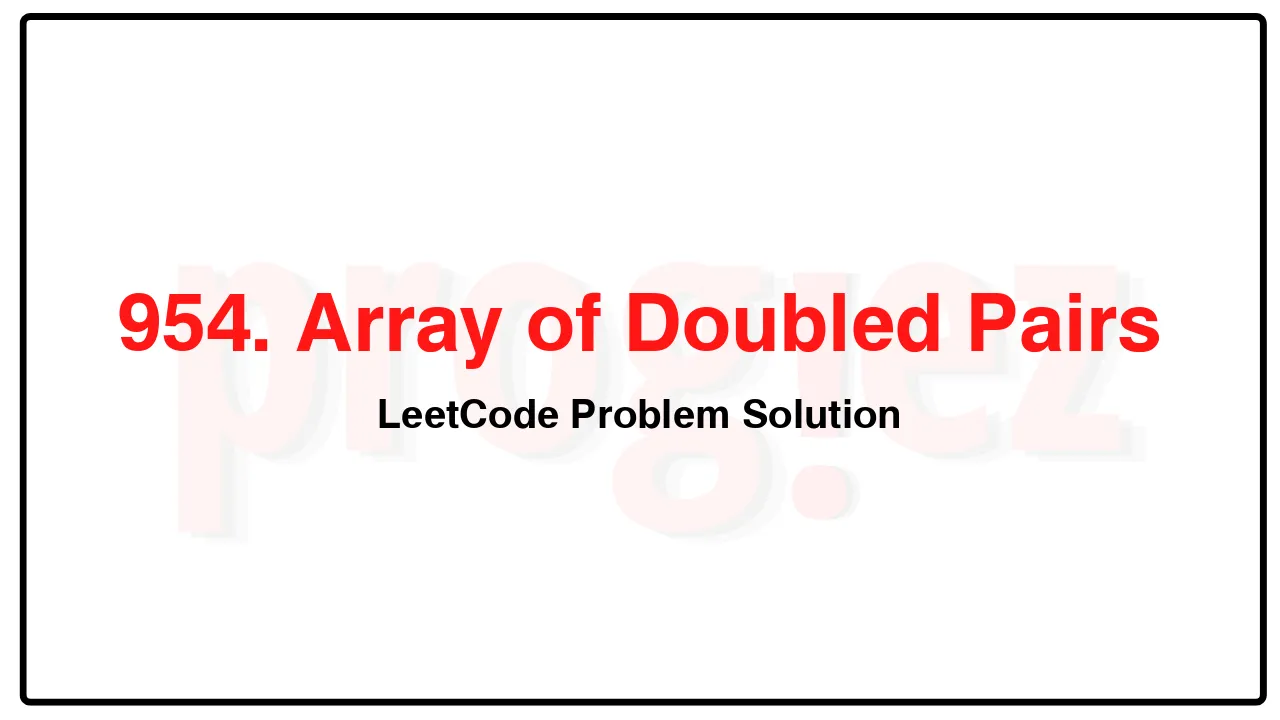
Problem Statement of Array of Doubled Pairs
Given an integer array of even length arr, return true if it is possible to reorder arr such that arr[2 * i + 1] = 2 * arr[2 * i] for every 0 <= i < len(arr) / 2, or false otherwise.
Example 1:
Input: arr = [3,1,3,6]
Output: false
Example 2:
Input: arr = [2,1,2,6]
Output: false
Example 3:
Input: arr = [4,-2,2,-4]
Output: true
Explanation: We can take two groups, [-2,-4] and [2,4] to form [-2,-4,2,4] or [2,4,-2,-4].
Constraints:
2 <= arr.length <= 3 * 104
arr.length is even.
-105 <= arr[i] <= 105
Complexity Analysis
- Time Complexity:
- Space Complexity:
954. Array of Doubled Pairs LeetCode Solution in C++
class Solution {
public:
bool canReorderDoubled(vector<int>& arr) {
unordered_map<int, int> count;
for (const int a : arr)
++count[a];
ranges::sort(arr, ranges::less{}, [](const int a) { return abs(a); });
for (const int a : arr) {
if (count[a] == 0)
continue;
if (count[2 * a] == 0)
return false;
--count[a];
--count[2 * a];
}
return true;
}
};
/* code provided by PROGIEZ */
954. Array of Doubled Pairs LeetCode Solution in Java
class Solution {
public boolean canReorderDoubled(int[] arr) {
Map<Integer, Integer> count = new HashMap<>();
for (final int a : arr)
count.merge(a, 1, Integer::sum);
arr = Arrays.stream(arr)
.boxed()
.sorted((a, b) -> Integer.compare(Math.abs(a), Math.abs(b)))
.mapToInt(Integer::intValue)
.toArray();
for (final int a : arr) {
if (count.get(a) == 0)
continue;
if (count.getOrDefault(2 * a, 0) == 0)
return false;
count.merge(a, -1, Integer::sum);
count.merge(2 * a, -1, Integer::sum);
}
return true;
}
}
// code provided by PROGIEZ
954. Array of Doubled Pairs LeetCode Solution in Python
class Solution:
def canReorderDoubled(self, arr: list[int]) -> bool:
count = collections.Counter(arr)
for key in sorted(count, key=abs):
if count[key] > count[2 * key]:
return False
count[2 * key] -= count[key]
return True
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.