910. Smallest Range II LeetCode Solution
In this guide, you will get 910. Smallest Range II LeetCode Solution with the best time and space complexity. The solution to Smallest Range II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Smallest Range II solution in C++
- Smallest Range II solution in Java
- Smallest Range II solution in Python
- Additional Resources
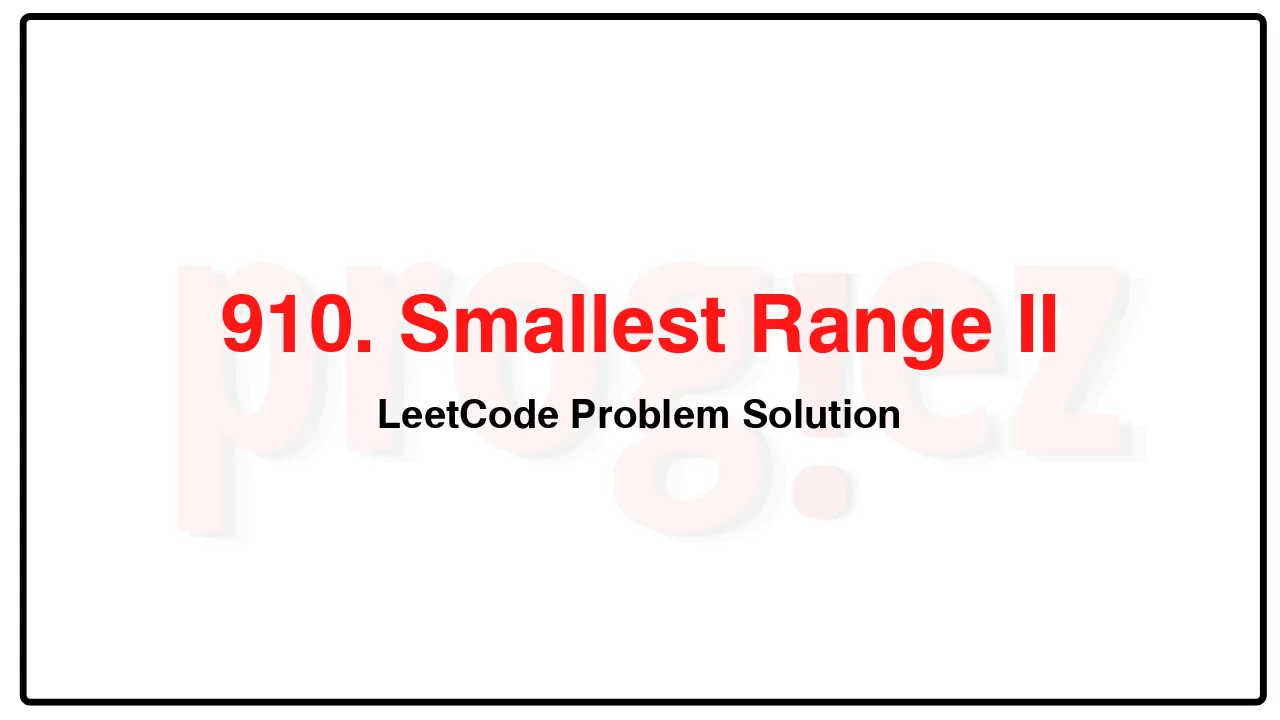
Problem Statement of Smallest Range II
You are given an integer array nums and an integer k.
For each index i where 0 <= i < nums.length, change nums[i] to be either nums[i] + k or nums[i] – k.
The score of nums is the difference between the maximum and minimum elements in nums.
Return the minimum score of nums after changing the values at each index.
Example 1:
Input: nums = [1], k = 0
Output: 0
Explanation: The score is max(nums) – min(nums) = 1 – 1 = 0.
Example 2:
Input: nums = [0,10], k = 2
Output: 6
Explanation: Change nums to be [2, 8]. The score is max(nums) – min(nums) = 8 – 2 = 6.
Example 3:
Input: nums = [1,3,6], k = 3
Output: 3
Explanation: Change nums to be [4, 6, 3]. The score is max(nums) – min(nums) = 6 – 3 = 3.
Constraints:
1 <= nums.length <= 104
0 <= nums[i] <= 104
0 <= k <= 104
Complexity Analysis
- Time Complexity: O(\texttt{sort})
- Space Complexity: O(\texttt{sort})
910. Smallest Range II LeetCode Solution in C++
class Solution {
public:
int smallestRangeII(vector<int>& nums, int k) {
ranges::sort(nums);
int ans = nums.back() - nums.front();
const int left = nums.front() + k;
const int right = nums.back() - k;
for (int i = 0; i + 1 < nums.size(); ++i) {
const int mn = min(left, nums[i + 1] - k);
const int mx = max(right, nums[i] + k);
ans = min(ans, mx - mn);
}
return ans;
}
};
/* code provided by PROGIEZ */
910. Smallest Range II LeetCode Solution in Java
class Solution {
public int smallestRangeII(int[] nums, int k) {
Arrays.sort(nums);
int ans = nums[nums.length - 1] - nums[0];
final int left = nums[0] + k;
final int right = nums[nums.length - 1] - k;
for (int i = 0; i + 1 < nums.length; ++i) {
final int mn = Math.min(left, nums[i + 1] - k);
final int mx = Math.max(right, nums[i] + k);
ans = Math.min(ans, mx - mn);
}
return ans;
}
}
// code provided by PROGIEZ
910. Smallest Range II LeetCode Solution in Python
class Solution:
def smallestRangeII(self, nums: list[int], k: int) -> int:
nums.sort()
ans = nums[-1] - nums[0]
left = nums[0] + k
right = nums[-1] - k
for a, b in itertools.pairwise(nums):
mn = min(left, b - k)
mx = max(right, a + k)
ans = min(ans, mx - mn)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.