849. Maximize Distance to Closest Person LeetCode Solution
In this guide, you will get 849. Maximize Distance to Closest Person LeetCode Solution with the best time and space complexity. The solution to Maximize Distance to Closest Person problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximize Distance to Closest Person solution in C++
- Maximize Distance to Closest Person solution in Java
- Maximize Distance to Closest Person solution in Python
- Additional Resources
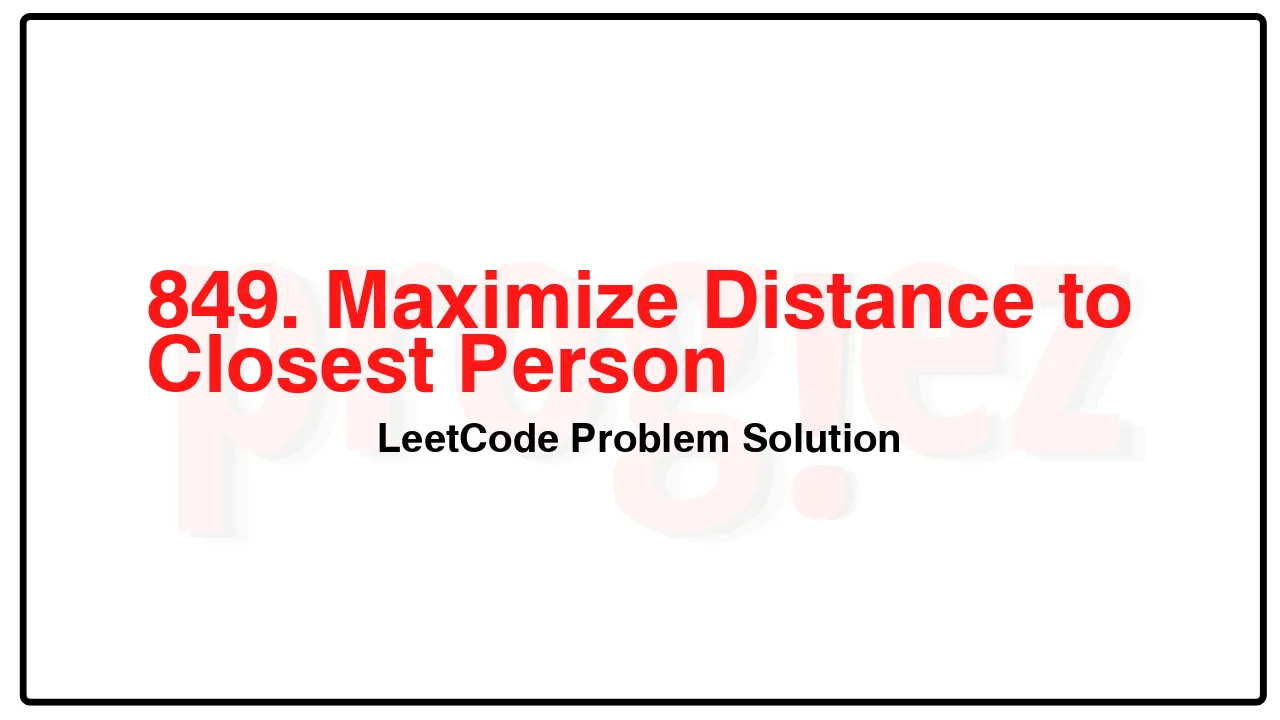
Problem Statement of Maximize Distance to Closest Person
You are given an array representing a row of seats where seats[i] = 1 represents a person sitting in the ith seat, and seats[i] = 0 represents that the ith seat is empty (0-indexed).
There is at least one empty seat, and at least one person sitting.
Alex wants to sit in the seat such that the distance between him and the closest person to him is maximized.
Return that maximum distance to the closest person.
Example 1:
Input: seats = [1,0,0,0,1,0,1]
Output: 2
Explanation:
If Alex sits in the second open seat (i.e. seats[2]), then the closest person has distance 2.
If Alex sits in any other open seat, the closest person has distance 1.
Thus, the maximum distance to the closest person is 2.
Example 2:
Input: seats = [1,0,0,0]
Output: 3
Explanation:
If Alex sits in the last seat (i.e. seats[3]), the closest person is 3 seats away.
This is the maximum distance possible, so the answer is 3.
Example 3:
Input: seats = [0,1]
Output: 1
Constraints:
2 <= seats.length <= 2 * 104
seats[i] is 0 or 1.
At least one seat is empty.
At least one seat is occupied.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
849. Maximize Distance to Closest Person LeetCode Solution in C++
class Solution {
public:
int maxDistToClosest(vector<int>& seats) {
const int n = seats.size();
int ans = 0;
int j = -1;
for (int i = 0; i < n; ++i)
if (seats[i] == 1) {
ans = j == -1 ? i : max(ans, (i - j) / 2);
j = i;
}
return max(ans, n - j - 1);
}
};
/* code provided by PROGIEZ */
849. Maximize Distance to Closest Person LeetCode Solution in Java
class Solution {
public int maxDistToClosest(int[] seats) {
final int n = seats.length;
int ans = 0;
int j = -1;
for (int i = 0; i < n; ++i)
if (seats[i] == 1) {
ans = j == -1 ? i : Math.max(ans, (i - j) / 2);
j = i;
}
return Math.max(ans, n - j - 1);
}
}
// code provided by PROGIEZ
849. Maximize Distance to Closest Person LeetCode Solution in Python
class Solution:
def maxDistToClosest(self, seats: list[int]) -> int:
n = len(seats)
ans = 0
j = -1
for i in range(n):
if seats[i] == 1:
ans = i if j == -1 else max(ans, (i - j) // 2)
j = i
return max(ans, n - j - 1)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.