836. Rectangle Overlap LeetCode Solution
In this guide, you will get 836. Rectangle Overlap LeetCode Solution with the best time and space complexity. The solution to Rectangle Overlap problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Rectangle Overlap solution in C++
- Rectangle Overlap solution in Java
- Rectangle Overlap solution in Python
- Additional Resources
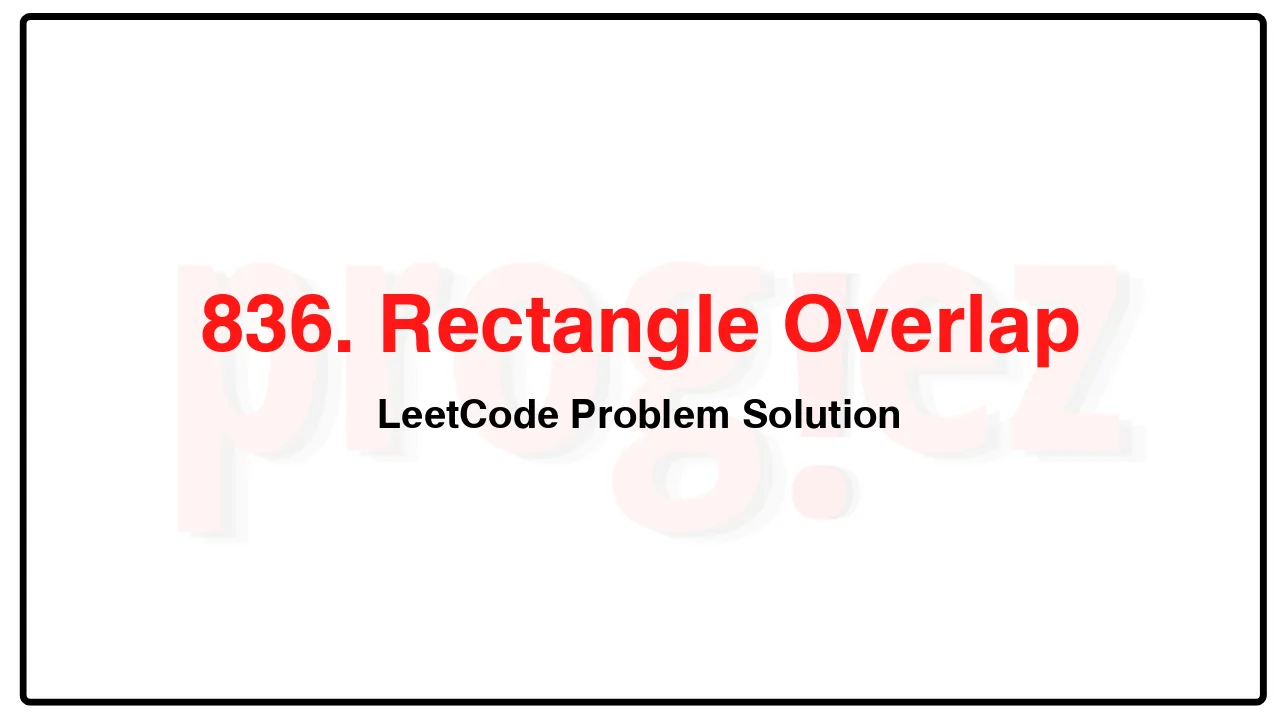
Problem Statement of Rectangle Overlap
An axis-aligned rectangle is represented as a list [x1, y1, x2, y2], where (x1, y1) is the coordinate of its bottom-left corner, and (x2, y2) is the coordinate of its top-right corner. Its top and bottom edges are parallel to the X-axis, and its left and right edges are parallel to the Y-axis.
Two rectangles overlap if the area of their intersection is positive. To be clear, two rectangles that only touch at the corner or edges do not overlap.
Given two axis-aligned rectangles rec1 and rec2, return true if they overlap, otherwise return false.
Example 1:
Input: rec1 = [0,0,2,2], rec2 = [1,1,3,3]
Output: true
Example 2:
Input: rec1 = [0,0,1,1], rec2 = [1,0,2,1]
Output: false
Example 3:
Input: rec1 = [0,0,1,1], rec2 = [2,2,3,3]
Output: false
Constraints:
rec1.length == 4
rec2.length == 4
-109 <= rec1[i], rec2[i] <= 109
rec1 and rec2 represent a valid rectangle with a non-zero area.
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
836. Rectangle Overlap LeetCode Solution in C++
class Solution {
public:
bool isRectangleOverlap(vector<int>& rec1, vector<int>& rec2) {
return rec1[0] < rec2[2] && rec2[0] < rec1[2] && //
rec1[1] < rec2[3] && rec2[1] < rec1[3];
}
};
/* code provided by PROGIEZ */
836. Rectangle Overlap LeetCode Solution in Java
class Solution {
public boolean isRectangleOverlap(int[] rec1, int[] rec2) {
return //
rec1[0] < rec2[2] && rec2[0] < rec1[2] && //
rec1[1] < rec2[3] && rec2[1] < rec1[3];
}
}
// code provided by PROGIEZ
836. Rectangle Overlap LeetCode Solution in Python
class Solution:
def isRectangleOverlap(self, rec1: list[int], rec2: list[int]) -> bool:
return rec1[0] < rec2[2] and rec2[0] < rec1[2] and rec1[1] < rec2[3] and rec2[1] < rec1[3]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.