819. Most Common Word LeetCode Solution
In this guide, you will get 819. Most Common Word LeetCode Solution with the best time and space complexity. The solution to Most Common Word problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Most Common Word solution in C++
- Most Common Word solution in Java
- Most Common Word solution in Python
- Additional Resources
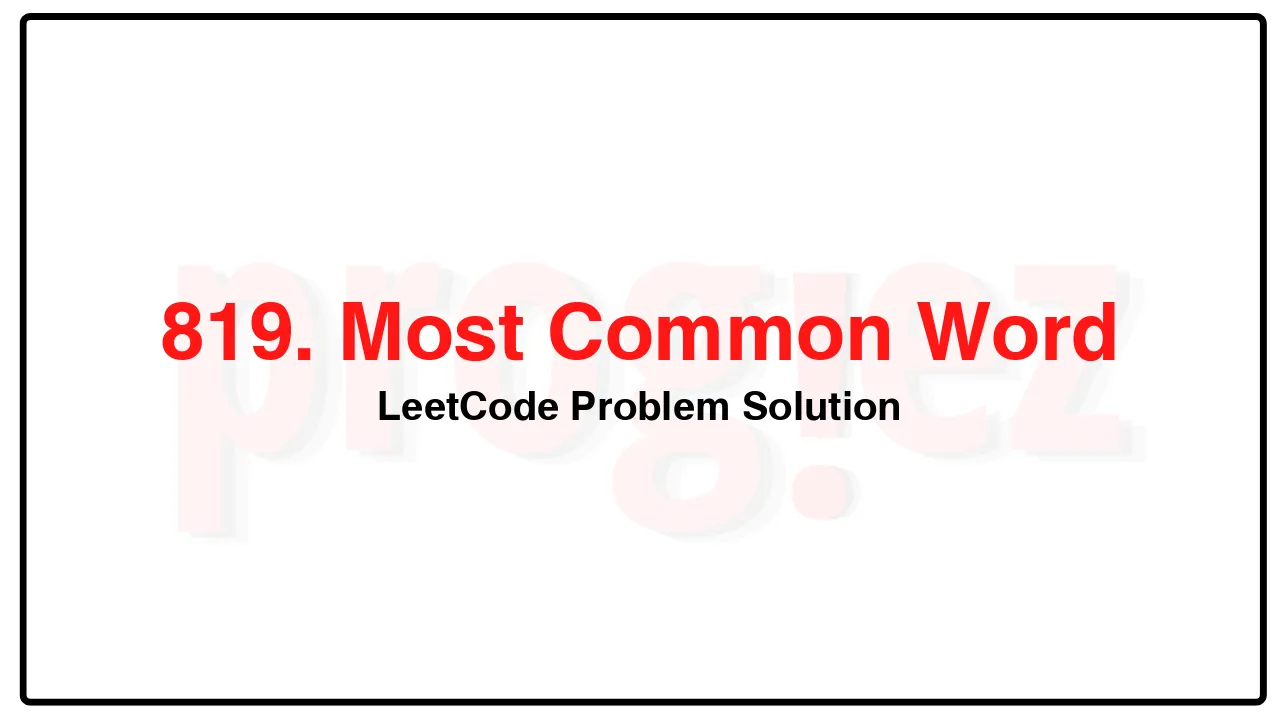
Problem Statement of Most Common Word
Given a string paragraph and a string array of the banned words banned, return the most frequent word that is not banned. It is guaranteed there is at least one word that is not banned, and that the answer is unique.
The words in paragraph are case-insensitive and the answer should be returned in lowercase.
Example 1:
Input: paragraph = “Bob hit a ball, the hit BALL flew far after it was hit.”, banned = [“hit”]
Output: “ball”
Explanation:
“hit” occurs 3 times, but it is a banned word.
“ball” occurs twice (and no other word does), so it is the most frequent non-banned word in the paragraph.
Note that words in the paragraph are not case sensitive,
that punctuation is ignored (even if adjacent to words, such as “ball,”),
and that “hit” isn’t the answer even though it occurs more because it is banned.
Example 2:
Input: paragraph = “a.”, banned = []
Output: “a”
Constraints:
1 <= paragraph.length <= 1000
paragraph consists of English letters, space ' ', or one of the symbols: "!?',;.".
0 <= banned.length <= 100
1 <= banned[i].length <= 10
banned[i] consists of only lowercase English letters.
Complexity Analysis
- Time Complexity: O(|\texttt{paragraph}|)
- Space Complexity: O(|\texttt{paragraph}| + |\texttt{banned}|)
819. Most Common Word LeetCode Solution in C++
class Solution {
public:
string mostCommonWord(string paragraph, vector<string>& banned) {
string ans;
int maxCount = 0;
unordered_map<string, int> count;
unordered_set<string> bannedSet{banned.begin(), banned.end()};
// Make the paragraph lowercased and remove all the punctuations.
for (char& c : paragraph)
c = isalpha(c) ? tolower(c) : ' ';
istringstream iss(paragraph);
for (string word; iss >> word;)
if (!bannedSet.contains(word))
++count[word];
for (const auto& [word, freq] : count)
if (freq > maxCount) {
maxCount = freq;
ans = word;
}
return ans;
}
};
/* code provided by PROGIEZ */
819. Most Common Word LeetCode Solution in Java
class Solution {
public String mostCommonWord(String paragraph, String[] banned) {
Pair<String, Integer> ans = new Pair<>("", 0);
Set<String> bannedSet = new HashSet<>(Arrays.asList(banned));
Map<String, Integer> count = new HashMap<>();
String[] words = paragraph.replaceAll("\\W+", " ").toLowerCase().split("\\s+");
for (final String word : words)
if (!bannedSet.contains(word))
if (count.merge(word, 1, Integer::sum) > ans.getValue())
ans = new Pair<>(word, count.get(word));
return ans.getKey();
}
}
// code provided by PROGIEZ
819. Most Common Word LeetCode Solution in Python
class Solution:
def mostCommonWord(self, paragraph: str, banned: list[str]) -> str:
banned = set(banned)
words = re.findall(r'\w+', paragraph.lower())
return collections.Counter(
word for word in words if word not in banned).most_common(1)[0][0]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.