700. Search in a Binary Search Tree LeetCode Solution
In this guide, you will get 700. Search in a Binary Search Tree LeetCode Solution with the best time and space complexity. The solution to Search in a Binary Search Tree problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Search in a Binary Search Tree solution in C++
- Search in a Binary Search Tree solution in Java
- Search in a Binary Search Tree solution in Python
- Additional Resources
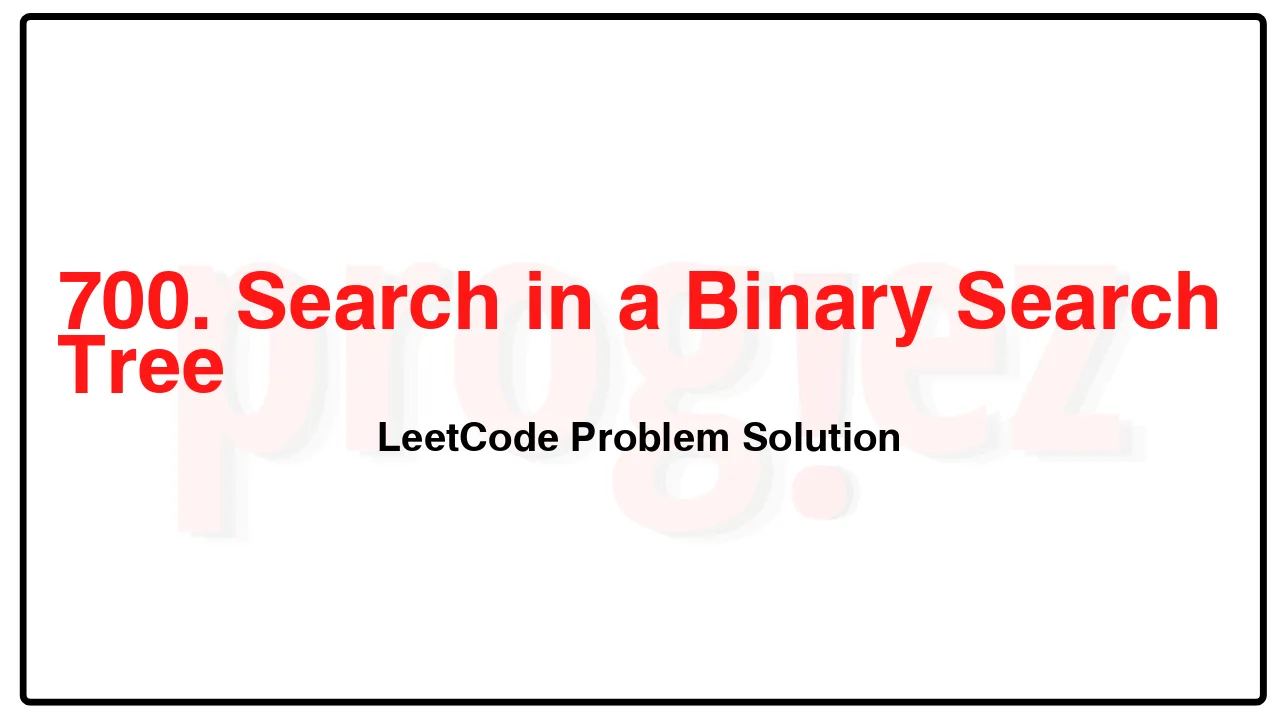
Problem Statement of Search in a Binary Search Tree
You are given the root of a binary search tree (BST) and an integer val.
Find the node in the BST that the node’s value equals val and return the subtree rooted with that node. If such a node does not exist, return null.
Example 1:
Input: root = [4,2,7,1,3], val = 2
Output: [2,1,3]
Example 2:
Input: root = [4,2,7,1,3], val = 5
Output: []
Constraints:
The number of nodes in the tree is in the range [1, 5000].
1 <= Node.val <= 107
root is a binary search tree.
1 <= val <= 107
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(h)
700. Search in a Binary Search Tree LeetCode Solution in C++
class Solution {
public:
TreeNode* searchBST(TreeNode* root, int val) {
if (root == nullptr)
return nullptr;
if (root->val == val)
return root;
if (root->val > val)
return searchBST(root->left, val);
return searchBST(root->right, val);
}
};
/* code provided by PROGIEZ */
700. Search in a Binary Search Tree LeetCode Solution in Java
class Solution {
public TreeNode searchBST(TreeNode root, int val) {
if (root == null)
return null;
if (root.val == val)
return root;
if (root.val > val)
return searchBST(root.left, val);
return searchBST(root.right, val);
}
}
// code provided by PROGIEZ
700. Search in a Binary Search Tree LeetCode Solution in Python
class Solution:
def searchBST(self, root: TreeNode | None, val: int) -> TreeNode | None:
if not root:
return None
if root.val == val:
return root
if root.val > val:
return self.searchBST(root.left, val)
return self.searchBST(root.right, val)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.