67. Add Binary LeetCode Solution
In this guide, you will get 67. Add Binary LeetCode Solution with the best time and space complexity. The solution to Add Binary problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Add Binary solution in C++
- Add Binary solution in Java
- Add Binary solution in Python
- Additional Resources
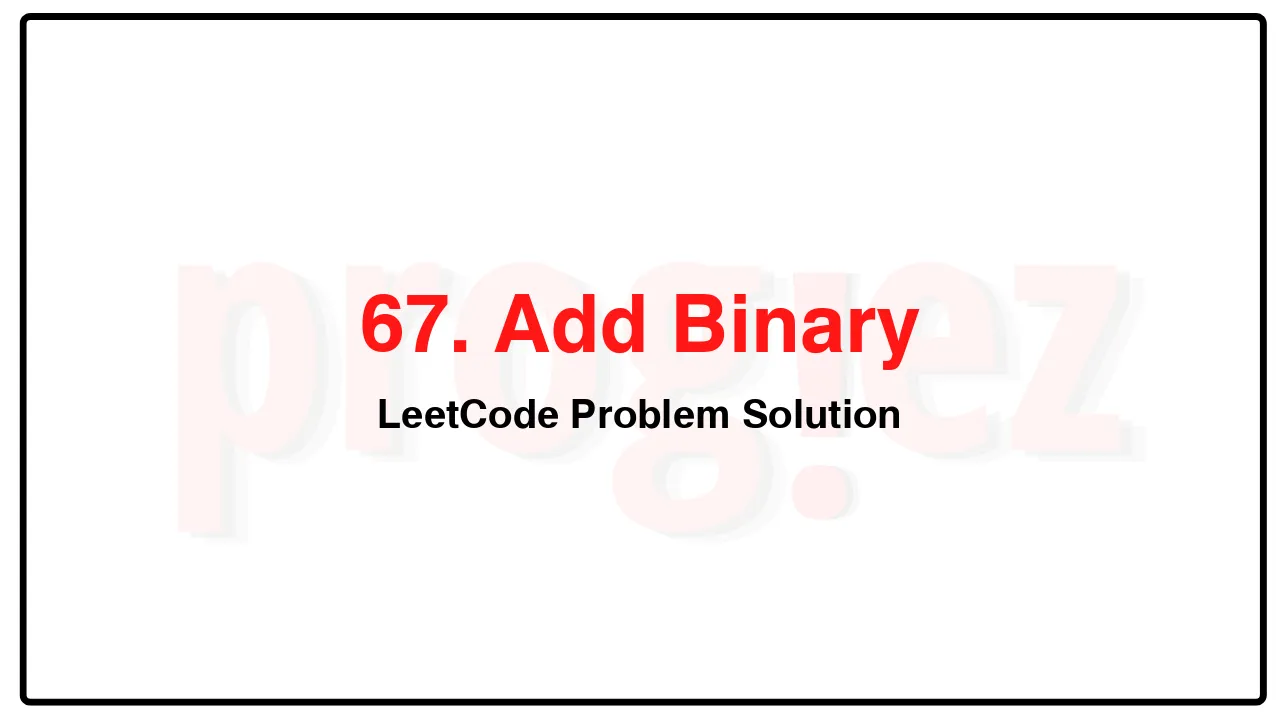
Problem Statement of Add Binary
Given two binary strings a and b, return their sum as a binary string.
Example 1:
Input: a = “11”, b = “1”
Output: “100”
Example 2:
Input: a = “1010”, b = “1011”
Output: “10101”
Constraints:
1 <= a.length, b.length <= 104
a and b consist only of '0' or '1' characters.
Each string does not contain leading zeros except for the zero itself.
Complexity Analysis
- Time Complexity: O(\max(|\texttt{a}|, |\texttt{b}|))
- Space Complexity: O(\max(|\texttt{a}|, |\texttt{b}|))
67. Add Binary LeetCode Solution in C++
class Solution {
public:
string addBinary(string a, string b) {
string ans;
int carry = 0;
int i = a.length() - 1;
int j = b.length() - 1;
while (i >= 0 || j >= 0 || carry) {
if (i >= 0)
carry += a[i--] - '0';
if (j >= 0)
carry += b[j--] - '0';
ans += carry % 2 + '0';
carry /= 2;
}
ranges::reverse(ans);
return ans;
}
};
/* code provided by PROGIEZ */
67. Add Binary LeetCode Solution in Java
class Solution {
public String addBinary(String a, String b) {
StringBuilder sb = new StringBuilder();
int carry = 0;
int i = a.length() - 1;
int j = b.length() - 1;
while (i >= 0 || j >= 0 || carry == 1) {
if (i >= 0)
carry += a.charAt(i--) - '0';
if (j >= 0)
carry += b.charAt(j--) - '0';
sb.append(carry % 2);
carry /= 2;
}
return sb.reverse().toString();
}
}
// code provided by PROGIEZ
67. Add Binary LeetCode Solution in Python
class Solution:
def addBinary(self, a: str, b: str) -> str:
ans = []
carry = 0
i = len(a) - 1
j = len(b) - 1
while i >= 0 or j >= 0 or carry:
if i >= 0:
carry += int(a[i])
i -= 1
if j >= 0:
carry += int(b[j])
j -= 1
ans.append(str(carry % 2))
carry //= 2
return ''.join(reversed(ans))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.