2733. Neither Minimum nor Maximum LeetCode Solution
In this guide, you will get 2733. Neither Minimum nor Maximum LeetCode Solution with the best time and space complexity. The solution to Neither Minimum nor Maximum problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Neither Minimum nor Maximum solution in C++
- Neither Minimum nor Maximum solution in Java
- Neither Minimum nor Maximum solution in Python
- Additional Resources
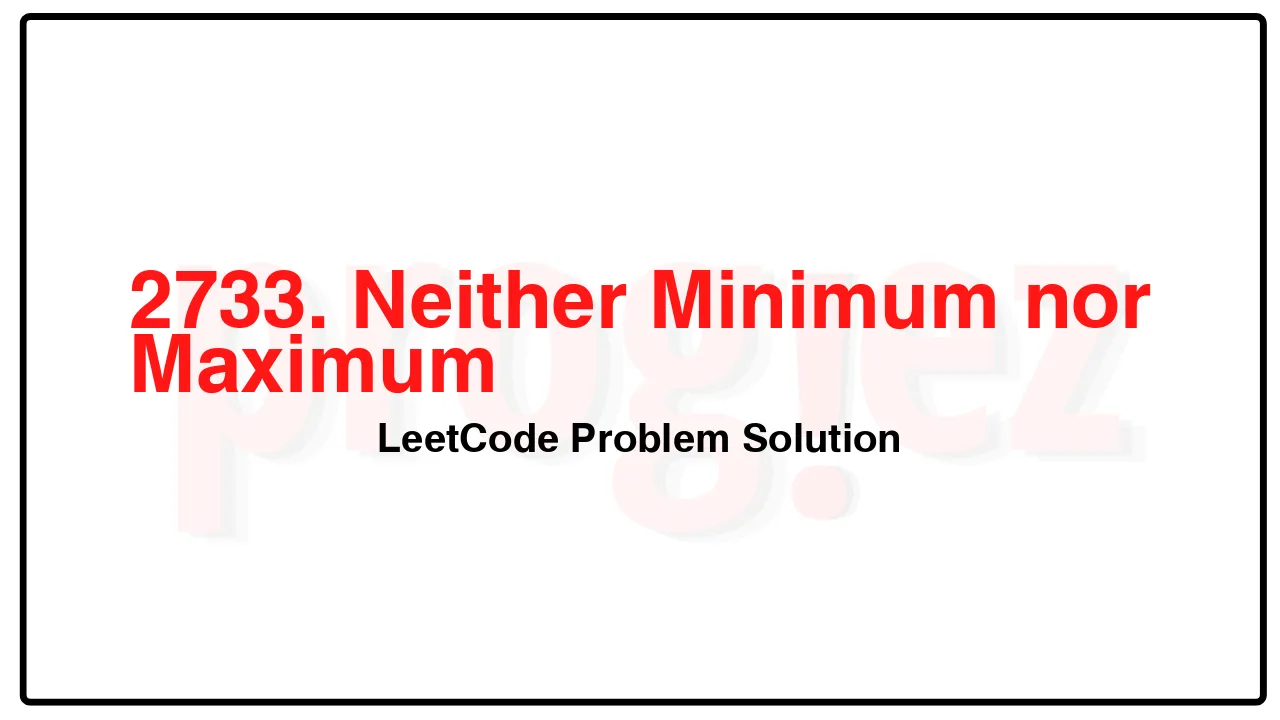
Problem Statement of Neither Minimum nor Maximum
Given an integer array nums containing distinct positive integers, find and return any number from the array that is neither the minimum nor the maximum value in the array, or -1 if there is no such number.
Return the selected integer.
Example 1:
Input: nums = [3,2,1,4]
Output: 2
Explanation: In this example, the minimum value is 1 and the maximum value is 4. Therefore, either 2 or 3 can be valid answers.
Example 2:
Input: nums = [1,2]
Output: -1
Explanation: Since there is no number in nums that is neither the maximum nor the minimum, we cannot select a number that satisfies the given condition. Therefore, there is no answer.
Example 3:
Input: nums = [2,1,3]
Output: 2
Explanation: Since 2 is neither the maximum nor the minimum value in nums, it is the only valid answer.
Constraints:
1 <= nums.length <= 100
1 <= nums[i] <= 100
All values in nums are distinct
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
2733. Neither Minimum nor Maximum LeetCode Solution in C++
class Solution {
public:
int findNonMinOrMax(vector<int>& nums) {
if (nums.size() < 3)
return -1;
sort(nums.begin(), nums.begin() + 3);
return nums[1];
}
};
/* code provided by PROGIEZ */
2733. Neither Minimum nor Maximum LeetCode Solution in Java
class Solution {
public int findNonMinOrMax(int[] nums) {
if (nums.length < 3)
return -1;
Arrays.sort(nums, 0, 3);
return nums[1];
}
}
// code provided by PROGIEZ
2733. Neither Minimum nor Maximum LeetCode Solution in Python
class Solution:
def findNonMinOrMax(self, nums: list[int]) -> int:
return -1 if len(nums) < 3 else sorted(nums[:3])[1]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.