2221. Find Triangular Sum of an Array LeetCode Solution
In this guide, you will get 2221. Find Triangular Sum of an Array LeetCode Solution with the best time and space complexity. The solution to Find Triangular Sum of an Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find Triangular Sum of an Array solution in C++
- Find Triangular Sum of an Array solution in Java
- Find Triangular Sum of an Array solution in Python
- Additional Resources
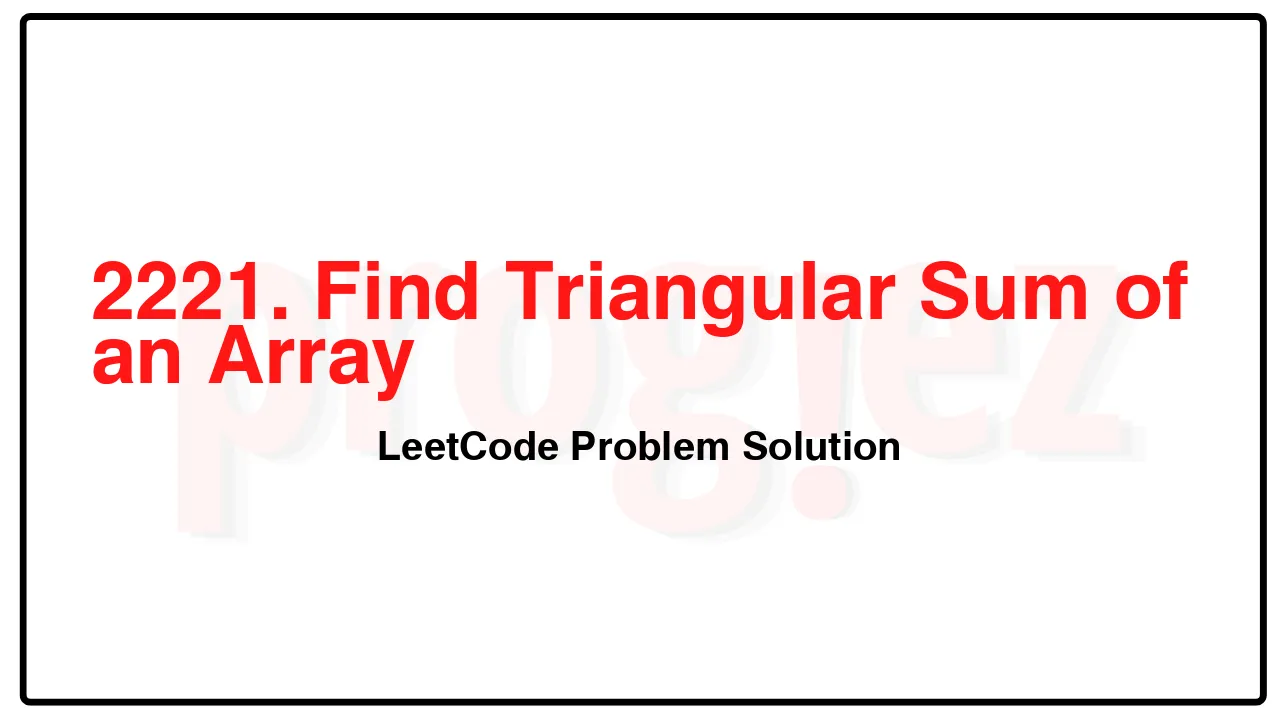
Problem Statement of Find Triangular Sum of an Array
You are given a 0-indexed integer array nums, where nums[i] is a digit between 0 and 9 (inclusive).
The triangular sum of nums is the value of the only element present in nums after the following process terminates:
Let nums comprise of n elements. If n == 1, end the process. Otherwise, create a new 0-indexed integer array newNums of length n – 1.
For each index i, where 0 <= i < n – 1, assign the value of newNums[i] as (nums[i] + nums[i+1]) % 10, where % denotes modulo operator.
Replace the array nums with newNums.
Repeat the entire process starting from step 1.
Return the triangular sum of nums.
Example 1:
Input: nums = [1,2,3,4,5]
Output: 8
Explanation:
The above diagram depicts the process from which we obtain the triangular sum of the array.
Example 2:
Input: nums = [5]
Output: 5
Explanation:
Since there is only one element in nums, the triangular sum is the value of that element itself.
Constraints:
1 <= nums.length <= 1000
0 <= nums[i] <= 9
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
2221. Find Triangular Sum of an Array LeetCode Solution in C++
class Solution {
public:
int triangularSum(vector<int>& nums) {
for (int sz = nums.size(); sz > 0; --sz)
for (int i = 0; i + 1 < sz; ++i)
nums[i] = (nums[i] + nums[i + 1]) % 10;
return nums[0];
}
};
/* code provided by PROGIEZ */
2221. Find Triangular Sum of an Array LeetCode Solution in Java
class Solution {
public int triangularSum(int[] nums) {
for (int sz = nums.length; sz > 0; --sz)
for (int i = 0; i + 1 < sz; ++i)
nums[i] = (nums[i] + nums[i + 1]) % 10;
return nums[0];
}
}
// code provided by PROGIEZ
2221. Find Triangular Sum of an Array LeetCode Solution in Python
class Solution:
def triangularSum(self, nums: list[int]) -> int:
for sz in range(len(nums), 0, -1):
for i in range(sz - 1):
nums[i] = (nums[i] + nums[i + 1]) % 10
return nums[0]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.