2012. Sum of Beauty in the Array LeetCode Solution
In this guide, you will get 2012. Sum of Beauty in the Array LeetCode Solution with the best time and space complexity. The solution to Sum of Beauty in the Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Sum of Beauty in the Array solution in C++
- Sum of Beauty in the Array solution in Java
- Sum of Beauty in the Array solution in Python
- Additional Resources
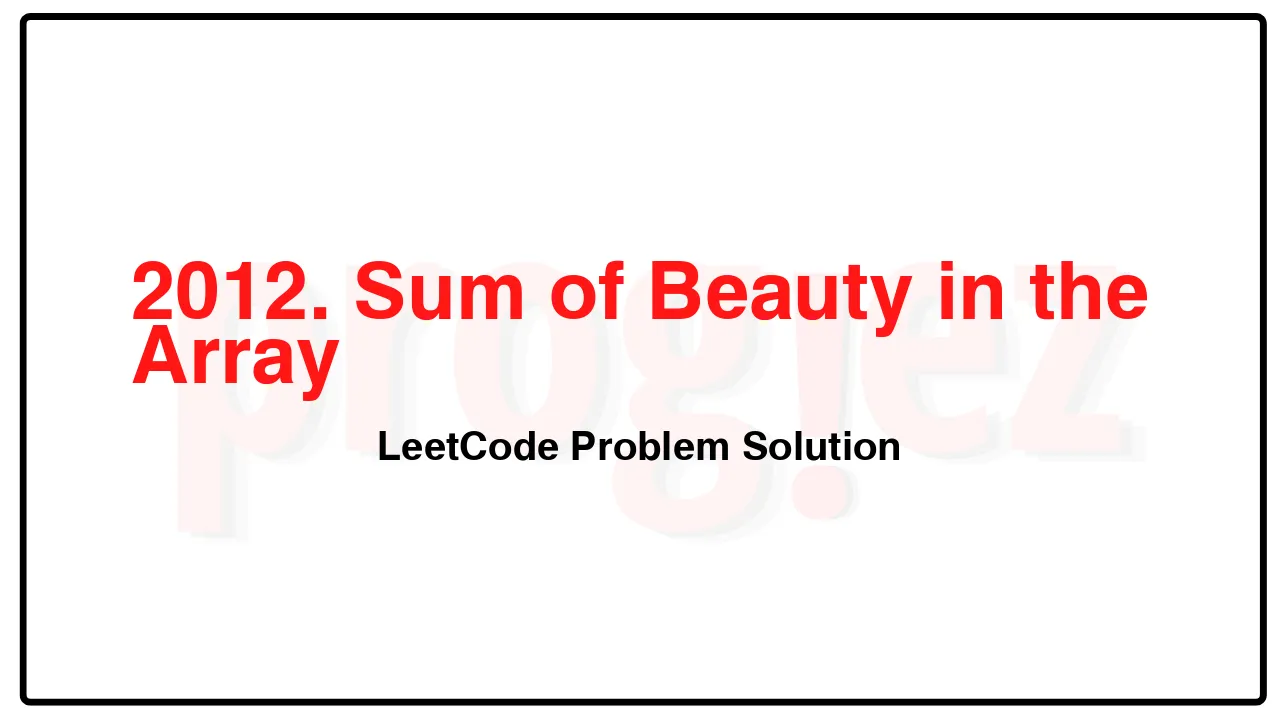
Problem Statement of Sum of Beauty in the Array
You are given a 0-indexed integer array nums. For each index i (1 <= i <= nums.length – 2) the beauty of nums[i] equals:
2, if nums[j] < nums[i] < nums[k], for all 0 <= j < i and for all i < k <= nums.length – 1.
1, if nums[i – 1] < nums[i] < nums[i + 1], and the previous condition is not satisfied.
0, if none of the previous conditions holds.
Return the sum of beauty of all nums[i] where 1 <= i <= nums.length – 2.
Example 1:
Input: nums = [1,2,3]
Output: 2
Explanation: For each index i in the range 1 <= i <= 1:
– The beauty of nums[1] equals 2.
Example 2:
Input: nums = [2,4,6,4]
Output: 1
Explanation: For each index i in the range 1 <= i <= 2:
– The beauty of nums[1] equals 1.
– The beauty of nums[2] equals 0.
Example 3:
Input: nums = [3,2,1]
Output: 0
Explanation: For each index i in the range 1 <= i <= 1:
– The beauty of nums[1] equals 0.
Constraints:
3 <= nums.length <= 105
1 <= nums[i] <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
2012. Sum of Beauty in the Array LeetCode Solution in C++
class Solution {
public:
int sumOfBeauties(vector<int>& nums) {
const int n = nums.size();
int ans = 0;
vector<int> minOfRight(n);
minOfRight.back() = nums.back();
for (int i = n - 2; i >= 2; --i)
minOfRight[i] = min(nums[i], minOfRight[i + 1]);
int maxOfLeft = nums[0];
for (int i = 1; i <= n - 2; ++i) {
if (maxOfLeft < nums[i] && nums[i] < minOfRight[i + 1])
ans += 2;
else if (nums[i - 1] < nums[i] && nums[i] < nums[i + 1])
ans += 1;
maxOfLeft = max(maxOfLeft, nums[i]);
}
return ans;
}
};
/* code provided by PROGIEZ */
2012. Sum of Beauty in the Array LeetCode Solution in Java
class Solution {
public int sumOfBeauties(int[] nums) {
final int n = nums.length;
int ans = 0;
int[] minOfRight = new int[n];
minOfRight[n - 1] = nums[n - 1];
for (int i = n - 2; i >= 2; --i)
minOfRight[i] = Math.min(nums[i], minOfRight[i + 1]);
int maxOfLeft = nums[0];
for (int i = 1; i <= n - 2; ++i) {
if (maxOfLeft < nums[i] && nums[i] < minOfRight[i + 1])
ans += 2;
else if (nums[i - 1] < nums[i] && nums[i] < nums[i + 1])
ans += 1;
maxOfLeft = Math.max(maxOfLeft, nums[i]);
}
return ans;
}
}
// code provided by PROGIEZ
2012. Sum of Beauty in the Array LeetCode Solution in Python
class Solution:
def sumOfBeauties(self, nums: list[int]) -> int:
n = len(nums)
ans = 0
minOfRight = [0] * (n - 1) + [nums[-1]]
for i in range(n - 2, 1, -1):
minOfRight[i] = min(nums[i], minOfRight[i + 1])
maxOfLeft = nums[0]
for i in range(1, n - 1):
if maxOfLeft < nums[i] < minOfRight[i + 1]:
ans += 2
elif nums[i - 1] < nums[i] < nums[i + 1]:
ans += 1
maxOfLeft = max(maxOfLeft, nums[i])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.