1897. Redistribute Characters to Make All Strings Equal LeetCode Solution
In this guide, you will get 1897. Redistribute Characters to Make All Strings Equal LeetCode Solution with the best time and space complexity. The solution to Redistribute Characters to Make All Strings Equal problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Redistribute Characters to Make All Strings Equal solution in C++
- Redistribute Characters to Make All Strings Equal solution in Java
- Redistribute Characters to Make All Strings Equal solution in Python
- Additional Resources
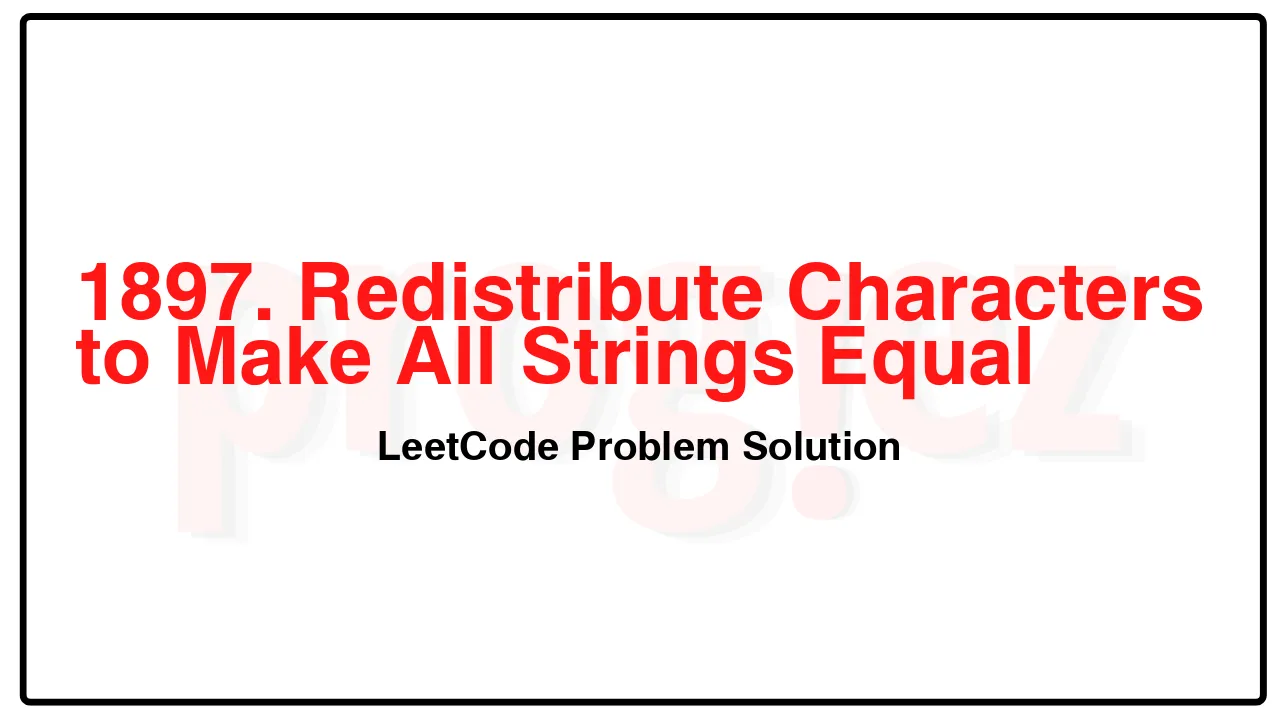
Problem Statement of Redistribute Characters to Make All Strings Equal
You are given an array of strings words (0-indexed).
In one operation, pick two distinct indices i and j, where words[i] is a non-empty string, and move any character from words[i] to any position in words[j].
Return true if you can make every string in words equal using any number of operations, and false otherwise.
Example 1:
Input: words = [“abc”,”aabc”,”bc”]
Output: true
Explanation: Move the first ‘a’ in words[1] to the front of words[2],
to make words[1] = “abc” and words[2] = “abc”.
All the strings are now equal to “abc”, so return true.
Example 2:
Input: words = [“ab”,”a”]
Output: false
Explanation: It is impossible to make all the strings equal using the operation.
Constraints:
1 <= words.length <= 100
1 <= words[i].length <= 100
words[i] consists of lowercase English letters.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(26) = O(1)
1897. Redistribute Characters to Make All Strings Equal LeetCode Solution in C++
class Solution {
public:
bool makeEqual(vector<string>& words) {
vector<int> count(26);
for (const string& word : words)
for (const char c : word)
++count[c - 'a'];
return ranges::all_of(count,
[&](const int c) { return c % words.size() == 0; });
}
};
/* code provided by PROGIEZ */
1897. Redistribute Characters to Make All Strings Equal LeetCode Solution in Java
class Solution {
public boolean makeEqual(String[] words) {
int[] count = new int[26];
for (final String word : words)
for (final char c : word.toCharArray())
++count[c - 'a'];
return Arrays.stream(count).allMatch(c -> c % words.length == 0);
}
}
// code provided by PROGIEZ
1897. Redistribute Characters to Make All Strings Equal LeetCode Solution in Python
class Solution:
def makeEqual(self, words: list[str]) -> bool:
return all(c % len(words) == 0
for c in collections.Counter(''.join(words)).values())
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.