1619. Mean of Array After Removing Some Elements LeetCode Solution
In this guide, you will get 1619. Mean of Array After Removing Some Elements LeetCode Solution with the best time and space complexity. The solution to Mean of Array After Removing Some Elements problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Mean of Array After Removing Some Elements solution in C++
- Mean of Array After Removing Some Elements solution in Java
- Mean of Array After Removing Some Elements solution in Python
- Additional Resources
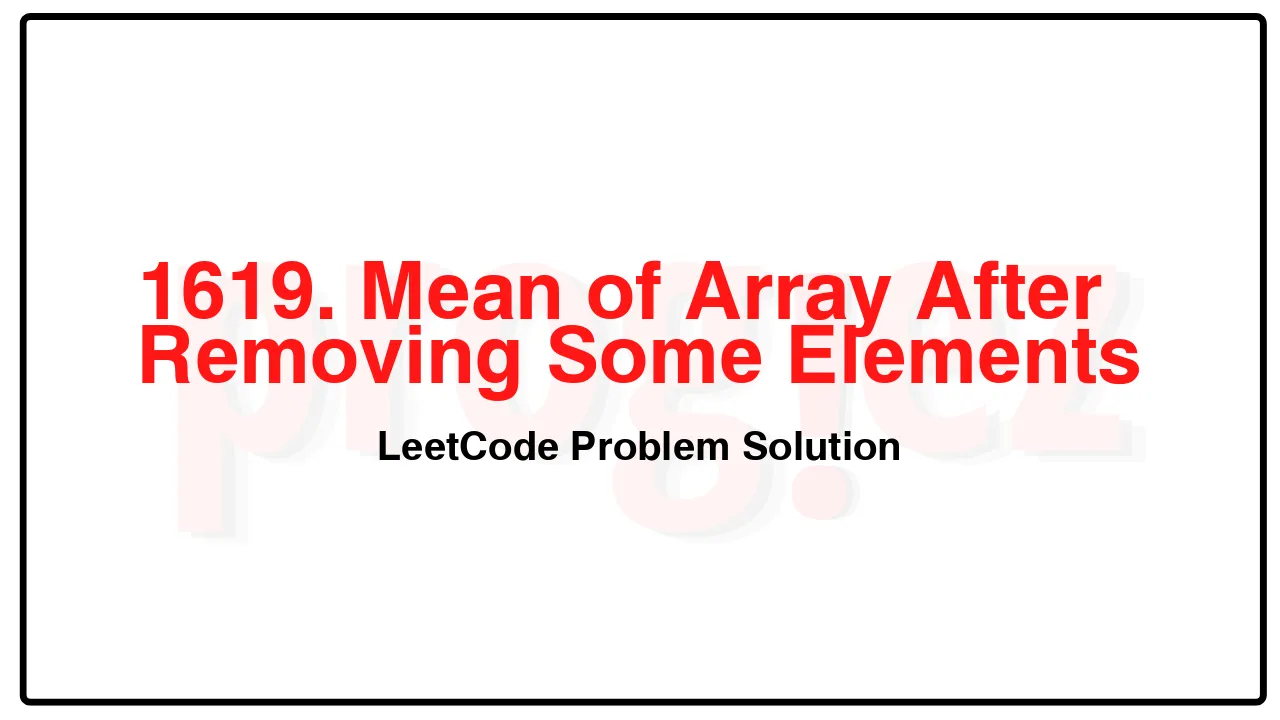
Problem Statement of Mean of Array After Removing Some Elements
Given an integer array arr, return the mean of the remaining integers after removing the smallest 5% and the largest 5% of the elements.
Answers within 10-5 of the actual answer will be considered accepted.
Example 1:
Input: arr = [1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,3]
Output: 2.00000
Explanation: After erasing the minimum and the maximum values of this array, all elements are equal to 2, so the mean is 2.
Example 2:
Input: arr = [6,2,7,5,1,2,0,3,10,2,5,0,5,5,0,8,7,6,8,0]
Output: 4.00000
Example 3:
Input: arr = [6,0,7,0,7,5,7,8,3,4,0,7,8,1,6,8,1,1,2,4,8,1,9,5,4,3,8,5,10,8,6,6,1,0,6,10,8,2,3,4]
Output: 4.77778
Constraints:
20 <= arr.length <= 1000
arr.length is a multiple of 20.
0 <= arr[i] <= 105
Complexity Analysis
- Time Complexity: O(|\texttt{sort}|)
- Space Complexity: O(|\texttt{sort}|)
1619. Mean of Array After Removing Some Elements LeetCode Solution in C++
class Solution {
public:
double trimMean(vector<int>& arr) {
int offset = arr.size() / 20;
nth_element(arr.begin(), arr.begin() + offset, arr.end());
nth_element(arr.begin() + offset, arr.end() - offset, arr.end());
double sum = accumulate(arr.begin() + offset, arr.end() - offset, 0.0);
return sum / (arr.size() - offset * 2);
}
};
/* code provided by PROGIEZ */
1619. Mean of Array After Removing Some Elements LeetCode Solution in Java
class Solution {
public double trimMean(int[] arr) {
Arrays.sort(arr);
final int offset = arr.length / 20;
return Arrays.stream(Arrays.copyOfRange(arr, offset, arr.length - offset)).average().orElse(0);
}
}
// code provided by PROGIEZ
1619. Mean of Array After Removing Some Elements LeetCode Solution in Python
class Solution:
def trimMean(self, arr: list[int]) -> float:
arr.sort()
offset = len(arr) // 20
return mean(arr[offset:-offset])
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.