1529. Minimum Suffix Flips LeetCode Solution
In this guide, you will get 1529. Minimum Suffix Flips LeetCode Solution with the best time and space complexity. The solution to Minimum Suffix Flips problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Suffix Flips solution in C++
- Minimum Suffix Flips solution in Java
- Minimum Suffix Flips solution in Python
- Additional Resources
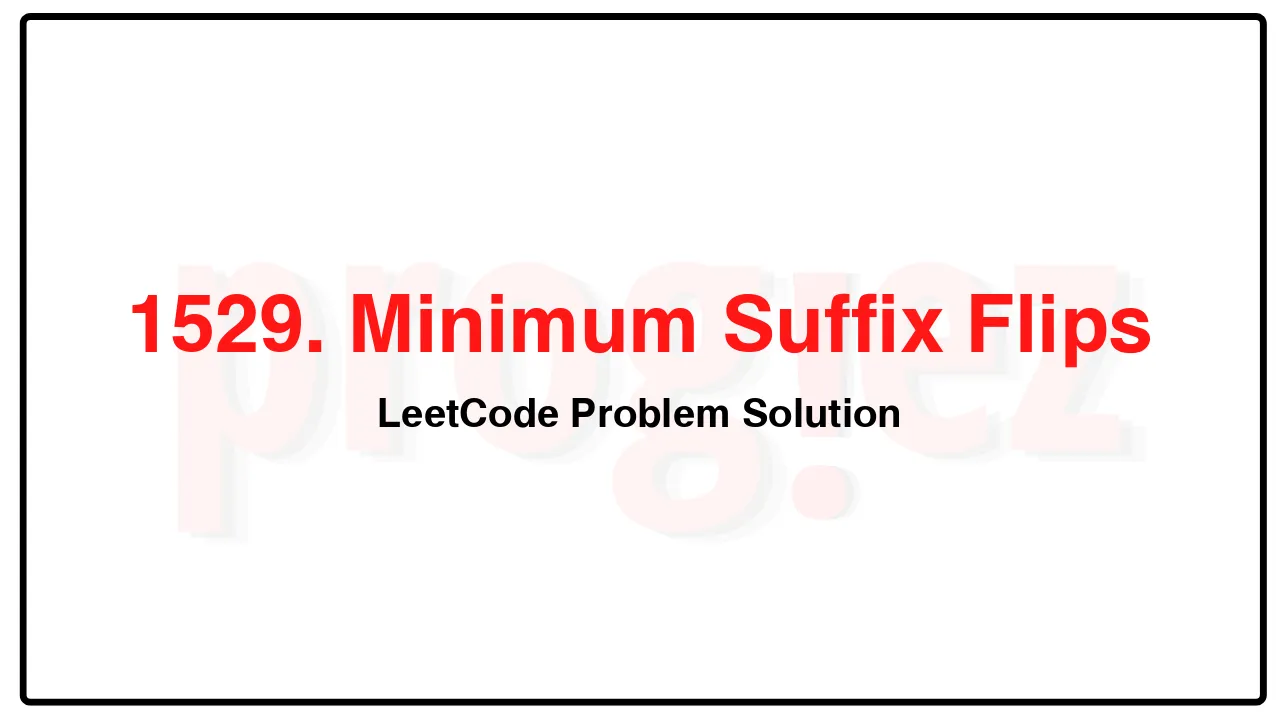
Problem Statement of Minimum Suffix Flips
You are given a 0-indexed binary string target of length n. You have another binary string s of length n that is initially set to all zeros. You want to make s equal to target.
In one operation, you can pick an index i where 0 <= i < n and flip all bits in the inclusive range [i, n – 1]. Flip means changing '0' to '1' and '1' to '0'.
Return the minimum number of operations needed to make s equal to target.
Example 1:
Input: target = “10111”
Output: 3
Explanation: Initially, s = “00000”.
Choose index i = 2: “00000” -> “00111”
Choose index i = 0: “00111” -> “11000”
Choose index i = 1: “11000” -> “10111”
We need at least 3 flip operations to form target.
Example 2:
Input: target = “101”
Output: 3
Explanation: Initially, s = “000”.
Choose index i = 0: “000” -> “111”
Choose index i = 1: “111” -> “100”
Choose index i = 2: “100” -> “101”
We need at least 3 flip operations to form target.
Example 3:
Input: target = “00000”
Output: 0
Explanation: We do not need any operations since the initial s already equals target.
Constraints:
n == target.length
1 <= n <= 105
target[i] is either '0' or '1'.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
1529. Minimum Suffix Flips LeetCode Solution in C++
class Solution {
public:
int minFlips(string target) {
int ans = 0;
int state = 0;
for (const char c : target)
if (c - '0' != state) {
state = c - '0';
++ans;
}
return ans;
}
};
/* code provided by PROGIEZ */
1529. Minimum Suffix Flips LeetCode Solution in Java
class Solution {
public int minFlips(String target) {
int ans = 0;
int state = 0;
for (final char c : target.toCharArray())
if (c - '0' != state) {
state = c - '0';
++ans;
}
return ans;
}
}
// code provided by PROGIEZ
1529. Minimum Suffix Flips LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.