1487. Making File Names Unique LeetCode Solution
In this guide, you will get 1487. Making File Names Unique LeetCode Solution with the best time and space complexity. The solution to Making File Names Unique problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Making File Names Unique solution in C++
- Making File Names Unique solution in Java
- Making File Names Unique solution in Python
- Additional Resources
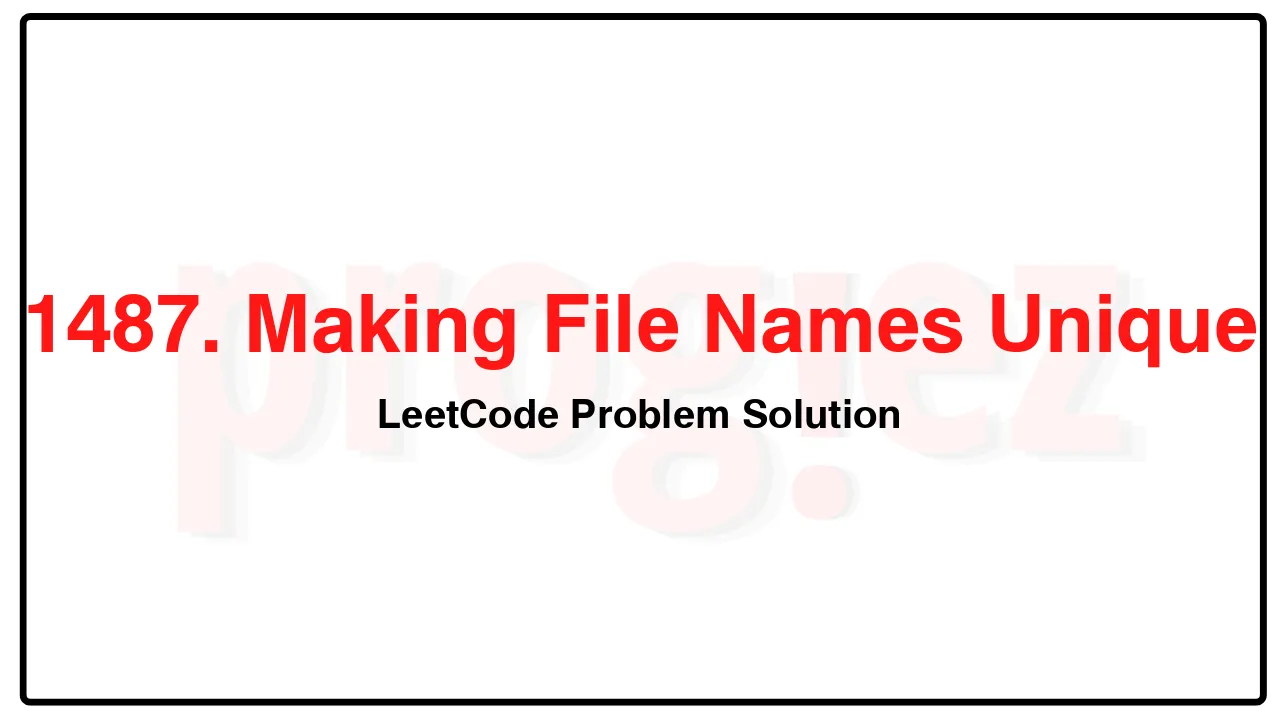
Problem Statement of Making File Names Unique
Given an array of strings names of size n. You will create n folders in your file system such that, at the ith minute, you will create a folder with the name names[i].
Since two files cannot have the same name, if you enter a folder name that was previously used, the system will have a suffix addition to its name in the form of (k), where, k is the smallest positive integer such that the obtained name remains unique.
Return an array of strings of length n where ans[i] is the actual name the system will assign to the ith folder when you create it.
Example 1:
Input: names = [“pes”,”fifa”,”gta”,”pes(2019)”]
Output: [“pes”,”fifa”,”gta”,”pes(2019)”]
Explanation: Let’s see how the file system creates folder names:
“pes” –> not assigned before, remains “pes”
“fifa” –> not assigned before, remains “fifa”
“gta” –> not assigned before, remains “gta”
“pes(2019)” –> not assigned before, remains “pes(2019)”
Example 2:
Input: names = [“gta”,”gta(1)”,”gta”,”avalon”]
Output: [“gta”,”gta(1)”,”gta(2)”,”avalon”]
Explanation: Let’s see how the file system creates folder names:
“gta” –> not assigned before, remains “gta”
“gta(1)” –> not assigned before, remains “gta(1)”
“gta” –> the name is reserved, system adds (k), since “gta(1)” is also reserved, systems put k = 2. it becomes “gta(2)”
“avalon” –> not assigned before, remains “avalon”
Example 3:
Input: names = [“onepiece”,”onepiece(1)”,”onepiece(2)”,”onepiece(3)”,”onepiece”]
Output: [“onepiece”,”onepiece(1)”,”onepiece(2)”,”onepiece(3)”,”onepiece(4)”]
Explanation: When the last folder is created, the smallest positive valid k is 4, and it becomes “onepiece(4)”.
Constraints:
1 <= names.length <= 5 * 104
1 <= names[i].length <= 20
names[i] consists of lowercase English letters, digits, and/or round brackets.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
1487. Making File Names Unique LeetCode Solution in C++
class Solution {
public:
vector<string> getFolderNames(vector<string>& names) {
vector<string> ans;
unordered_map<string, int> nameToSuffix;
for (const string& name : names)
if (const auto it = nameToSuffix.find(name); it != nameToSuffix.cend()) {
int suffix = it->second;
string newName = getName(name, ++suffix);
while (nameToSuffix.contains(newName))
newName = getName(name, ++suffix);
nameToSuffix[name] = suffix;
nameToSuffix[newName] = 0;
ans.push_back(newName);
} else {
nameToSuffix[name] = 0;
ans.push_back(name);
}
return ans;
}
private:
string getName(const string& name, int suffix) {
return name + "(" + to_string(suffix) + ")";
}
};
/* code provided by PROGIEZ */
1487. Making File Names Unique LeetCode Solution in Java
class Solution {
public String[] getFolderNames(String[] names) {
String[] ans = new String[names.length];
Map<String, Integer> nameToSuffix = new HashMap<>();
for (int i = 0; i < names.length; ++i) {
final String name = names[i];
if (nameToSuffix.containsKey(name)) {
int suffix = nameToSuffix.get(name);
String newName = getName(name, ++suffix);
while (nameToSuffix.containsKey(newName))
newName = getName(name, ++suffix);
nameToSuffix.put(name, suffix);
nameToSuffix.put(newName, 0);
ans[i] = newName;
} else {
nameToSuffix.put(name, 0);
ans[i] = name;
}
}
return ans;
}
private String getName(final String name, int suffix) {
return name + "(" + String.valueOf(suffix) + ")";
}
}
// code provided by PROGIEZ
1487. Making File Names Unique LeetCode Solution in Python
class Solution:
def getFolderNames(self, names: list[str]) -> list[str]:
ans = []
nameToSuffix = {}
for name in names:
if name in nameToSuffix:
suffix = nameToSuffix[name] + 1
newName = self._getName(name, suffix)
while newName in nameToSuffix:
suffix += 1
newName = self._getName(name, suffix)
nameToSuffix[name] = suffix
nameToSuffix[newName] = 0
ans.append(newName)
else:
nameToSuffix[name] = 0
ans.append(name)
return ans
def _getName(self, name: str, suffix: int) -> str:
return name + '(' + str(suffix) + ')'
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.