1410. HTML Entity Parser LeetCode Solution
In this guide, you will get 1410. HTML Entity Parser LeetCode Solution with the best time and space complexity. The solution to HTML Entity Parser problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- HTML Entity Parser solution in C++
- HTML Entity Parser solution in Java
- HTML Entity Parser solution in Python
- Additional Resources
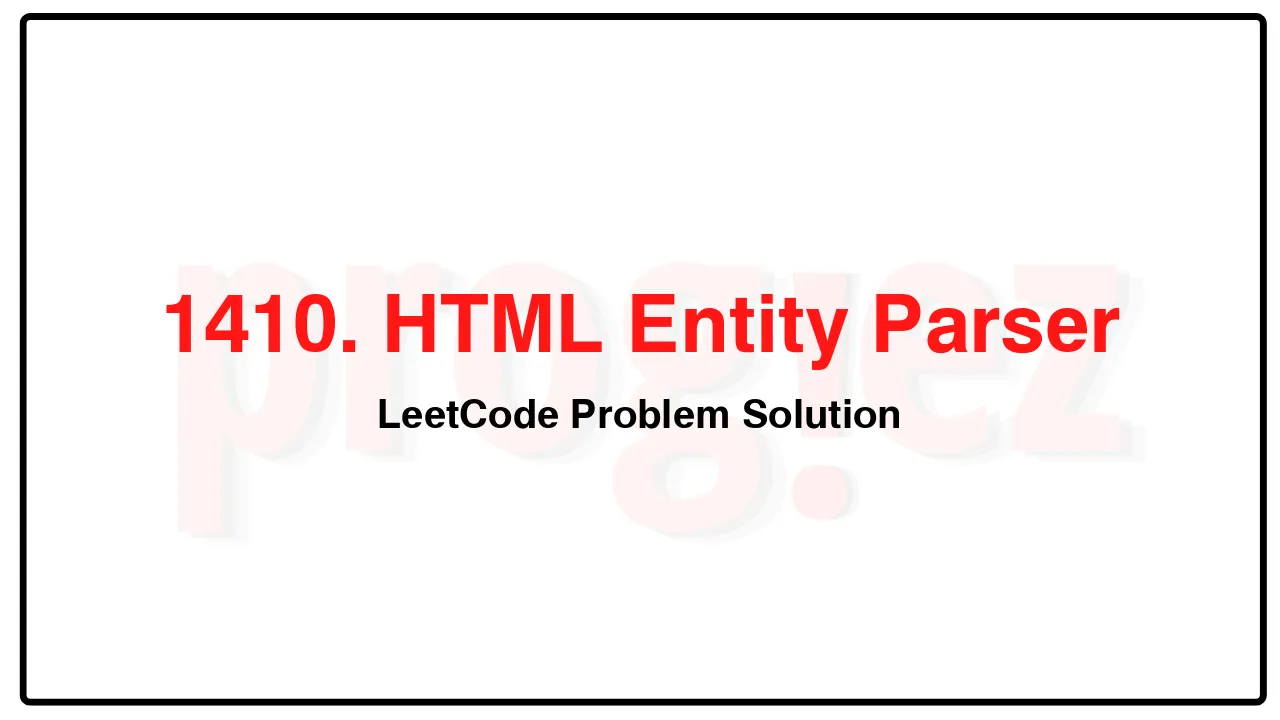
Problem Statement of HTML Entity Parser
HTML entity parser is the parser that takes HTML code as input and replace all the entities of the special characters by the characters itself.
The special characters and their entities for HTML are:
Quotation Mark: the entity is " and symbol character is “.
Single Quote Mark: the entity is ' and symbol character is ‘.
Ampersand: the entity is & and symbol character is &.
Greater Than Sign: the entity is > and symbol character is >.
Less Than Sign: the entity is < and symbol character is <.
Slash: the entity is ⁄ and symbol character is /.
Given the input text string to the HTML parser, you have to implement the entity parser.
Return the text after replacing the entities by the special characters.
Example 1:
Input: text = “& is an HTML entity but &ambassador; is not.”
Output: “& is an HTML entity but &ambassador; is not.”
Explanation: The parser will replace the & entity by &
Example 2:
Input: text = “and I quote: "…"”
Output: “and I quote: \”…\””
Constraints:
1 <= text.length <= 105
The string may contain any possible characters out of all the 256 ASCII characters.
Complexity Analysis
- Time Complexity: O(|\texttt{text}|)
- Space Complexity: O(|\texttt{text}|)
1410. HTML Entity Parser LeetCode Solution in C++
class Solution {
public:
string entityParser(string text) {
const unordered_map<string, char> entityToChar{
{""", '"'}, {"'", '\''}, {"&", '&'},
{">", '>'}, {"<", '<'}, {"⁄", '/'}};
string ans;
int j = 0; // text[j..ampersandIndex - 1] is the pending substring.
int ampersandIndex = -1;
for (int i = 0; i < text.length(); ++i)
if (text[i] == '&') {
ampersandIndex = i;
} else if (text[i] == ';' && ampersandIndex >= j) {
const string sub = text.substr(ampersandIndex, i - ampersandIndex + 1);
ans += text.substr(j, ampersandIndex - j);
ans += getCharIfMatched(text, sub, entityToChar);
j = i + 1;
}
return ans + text.substr(j);
}
private:
string getCharIfMatched(const string& text, const string& sub,
const unordered_map<string, char>& entityToChar) {
for (const auto& [entity, c] : entityToChar)
if (entity == sub)
return string(1, c);
return sub;
}
};
/* code provided by PROGIEZ */
1410. HTML Entity Parser LeetCode Solution in Java
class Solution {
public String entityParser(String text) {
Map<String, String> entryToChar =
Map.of(""", "\"", "'", "'", ">", ">", "<", "<", "⁄", "/");
for (Map.Entry<String, String> entry : entryToChar.entrySet()) {
final String entity = entry.getKey();
final String c = entry.getValue();
text = text.replaceAll(entity, c);
}
// Process '&' in last.
return text.replaceAll("&", "&");
}
}
// code provided by PROGIEZ
1410. HTML Entity Parser LeetCode Solution in Python
class Solution:
def entityParser(self, text: str) -> str:
entityToChar = {'"': '"', ''': '\'',
'>': '>', '<': '<', '⁄': '/'}
for entity, c in entityToChar.items():
text = text.replace(entity, c)
# Process '&' in last.
return text.replace('&', '&')
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.