1287. Element Appearing More Than 25% In Sorted Array LeetCode Solution
In this guide, you will get 1287. Element Appearing More Than 25% In Sorted Array LeetCode Solution with the best time and space complexity. The solution to Element Appearing More Than % In Sorted Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Element Appearing More Than % In Sorted Array solution in C++
- Element Appearing More Than % In Sorted Array solution in Java
- Element Appearing More Than % In Sorted Array solution in Python
- Additional Resources
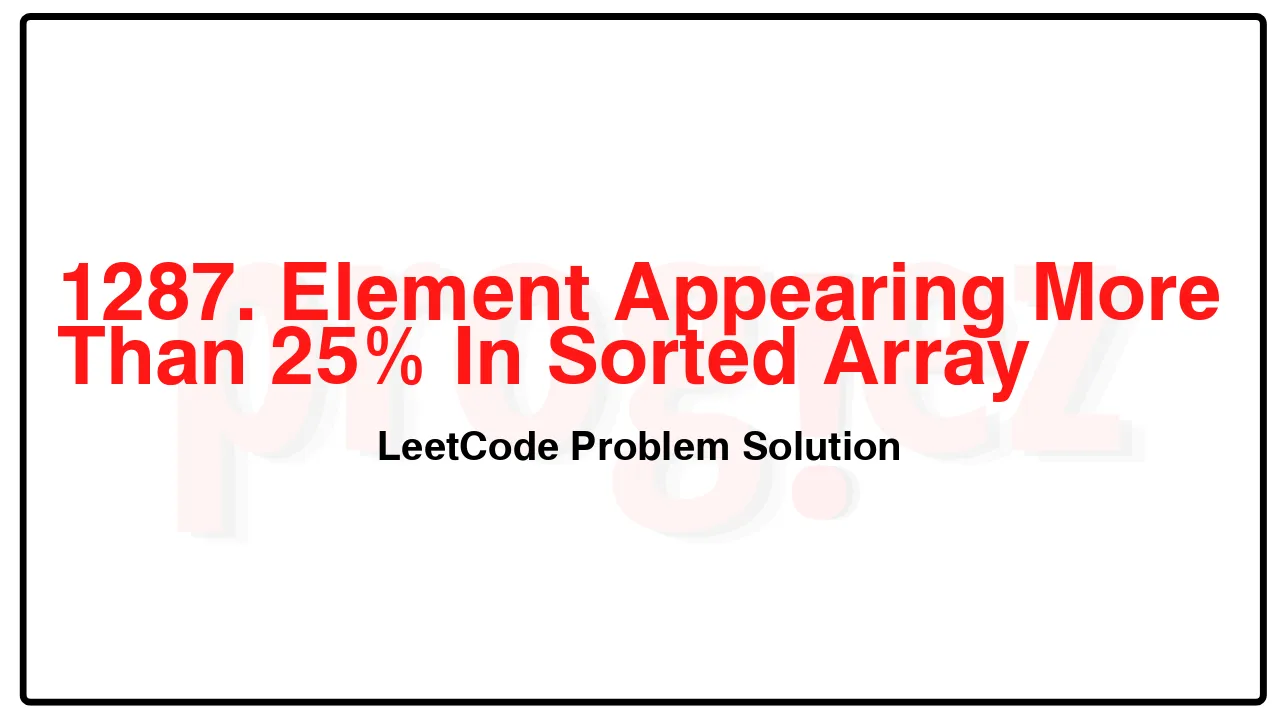
Problem Statement of Element Appearing More Than % In Sorted Array
Given an integer array sorted in non-decreasing order, there is exactly one integer in the array that occurs more than 25% of the time, return that integer.
Example 1:
Input: arr = [1,2,2,6,6,6,6,7,10]
Output: 6
Example 2:
Input: arr = [1,1]
Output: 1
Constraints:
1 <= arr.length <= 104
0 <= arr[i] <= 105
Complexity Analysis
- Time Complexity:
- Space Complexity: O(1)
1287. Element Appearing More Than 25% In Sorted Array LeetCode Solution in C++
class Solution {
public:
int findSpecialInteger(vector<int>& arr) {
const int n = arr.size();
const int quarter = n / 4;
for (int i = 0; i < n - quarter; ++i)
if (arr[i] == arr[i + quarter])
return arr[i];
throw;
}
};
/* code provided by PROGIEZ */
1287. Element Appearing More Than 25% In Sorted Array LeetCode Solution in Java
class Solution {
public int findSpecialInteger(int[] arr) {
final int n = arr.length;
final int quarter = n / 4;
for (int i = 0; i < n - quarter; ++i)
if (arr[i] == arr[i + quarter])
return arr[i];
throw new IllegalArgumentException();
}
}
// code provided by PROGIEZ
1287. Element Appearing More Than 25% In Sorted Array LeetCode Solution in Python
class Solution:
def findSpecialInteger(self, arr: list[int]) -> int:
n = len(arr)
quarter = n // 4
for i in range(n - quarter):
if arr[i] == arr[i + quarter]:
return arr[i]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.