1232. Check If It Is a Straight Line LeetCode Solution
In this guide, you will get 1232. Check If It Is a Straight Line LeetCode Solution with the best time and space complexity. The solution to Check If It Is a Straight Line problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Check If It Is a Straight Line solution in C++
- Check If It Is a Straight Line solution in Java
- Check If It Is a Straight Line solution in Python
- Additional Resources
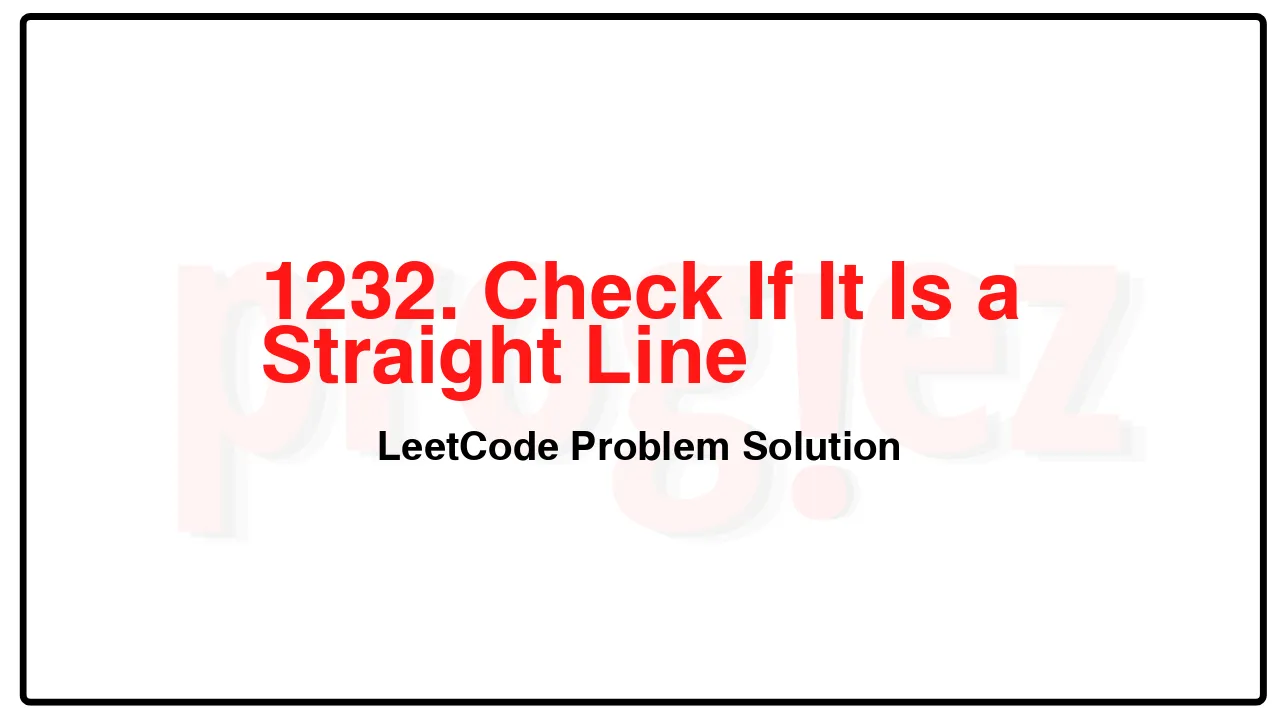
Problem Statement of Check If It Is a Straight Line
You are given an array coordinates, coordinates[i] = [x, y], where [x, y] represents the coordinate of a point. Check if these points make a straight line in the XY plane.
Example 1:
Input: coordinates = [[1,2],[2,3],[3,4],[4,5],[5,6],[6,7]]
Output: true
Example 2:
Input: coordinates = [[1,1],[2,2],[3,4],[4,5],[5,6],[7,7]]
Output: false
Constraints:
2 <= coordinates.length <= 1000
coordinates[i].length == 2
-10^4 <= coordinates[i][0], coordinates[i][1] <= 10^4
coordinates contains no duplicate point.
Complexity Analysis
- Time Complexity:
- Space Complexity:
1232. Check If It Is a Straight Line LeetCode Solution in C++
class Solution {
public:
bool checkStraightLine(vector<vector<int>>& coordinates) {
int x0 = coordinates[0][0];
int y0 = coordinates[0][1];
int x1 = coordinates[1][0];
int y1 = coordinates[1][1];
int dx = x1 - x0;
int dy = y1 - y0;
for (int i = 2; i < coordinates.size(); ++i) {
int x = coordinates[i][0];
int y = coordinates[i][1];
if ((x - x0) * dy != (y - y0) * dx)
return false;
}
return true;
}
};
/* code provided by PROGIEZ */
1232. Check If It Is a Straight Line LeetCode Solution in Java
class Solution {
public boolean checkStraightLine(int[][] coordinates) {
int x0 = coordinates[0][0];
int y0 = coordinates[0][1];
int x1 = coordinates[1][0];
int y1 = coordinates[1][1];
int dx = x1 - x0;
int dy = y1 - y0;
for (int i = 2; i < coordinates.length; ++i) {
int x = coordinates[i][0];
int y = coordinates[i][1];
if ((x - x0) * dy != (y - y0) * dx)
return false;
}
return true;
}
}
// code provided by PROGIEZ
1232. Check If It Is a Straight Line LeetCode Solution in Python
class Solution:
def checkStraightLine(self, coordinates: list[list[int]]) -> bool:
x0, y0, x1, y1 = *coordinates[0], *coordinates[1]
dx = x1 - x0
dy = y1 - y0
return all((x - x0) * dy == (y - y0) * dx for x, y in coordinates)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.