1217. Minimum Cost to Move Chips to The Same Position LeetCode Solution
In this guide, you will get 1217. Minimum Cost to Move Chips to The Same Position LeetCode Solution with the best time and space complexity. The solution to Minimum Cost to Move Chips to The Same Position problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Cost to Move Chips to The Same Position solution in C++
- Minimum Cost to Move Chips to The Same Position solution in Java
- Minimum Cost to Move Chips to The Same Position solution in Python
- Additional Resources
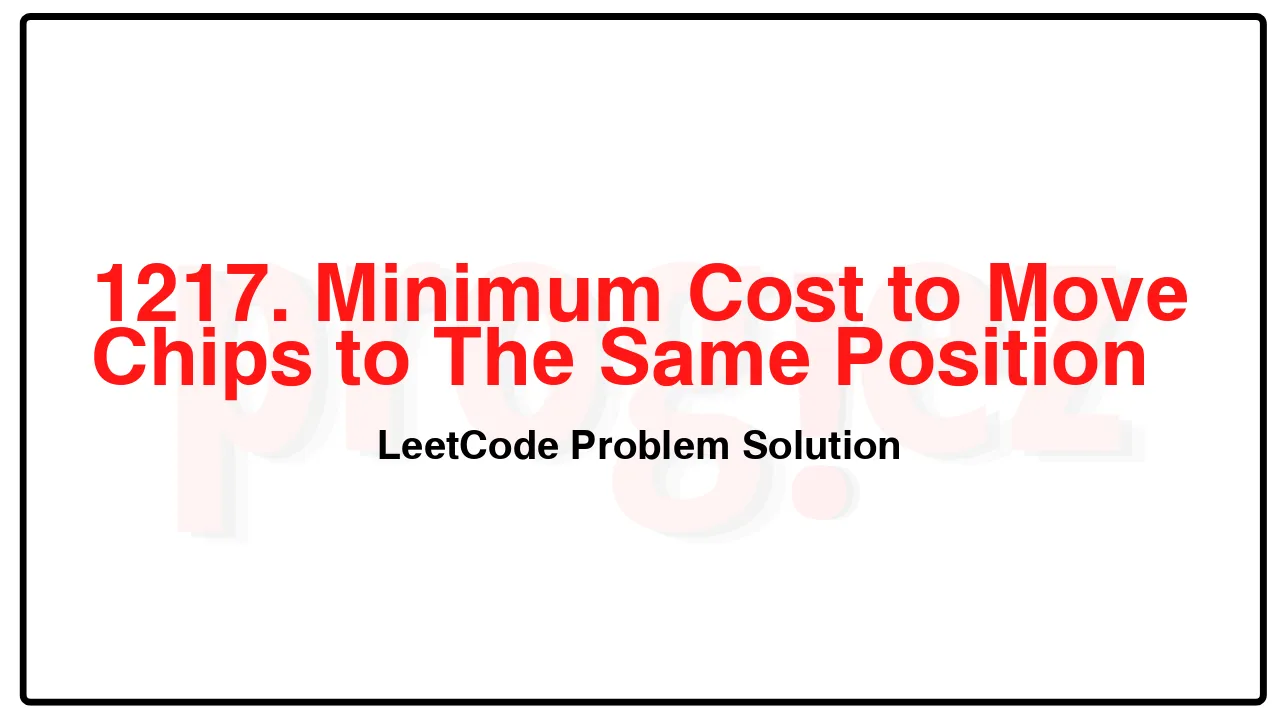
Problem Statement of Minimum Cost to Move Chips to The Same Position
We have n chips, where the position of the ith chip is position[i].
We need to move all the chips to the same position. In one step, we can change the position of the ith chip from position[i] to:
position[i] + 2 or position[i] – 2 with cost = 0.
position[i] + 1 or position[i] – 1 with cost = 1.
Return the minimum cost needed to move all the chips to the same position.
Example 1:
Input: position = [1,2,3]
Output: 1
Explanation: First step: Move the chip at position 3 to position 1 with cost = 0.
Second step: Move the chip at position 2 to position 1 with cost = 1.
Total cost is 1.
Example 2:
Input: position = [2,2,2,3,3]
Output: 2
Explanation: We can move the two chips at position 3 to position 2. Each move has cost = 1. The total cost = 2.
Example 3:
Input: position = [1,1000000000]
Output: 1
Constraints:
1 <= position.length <= 100
1 <= position[i] <= 10^9
Complexity Analysis
- Time Complexity:
- Space Complexity:
1217. Minimum Cost to Move Chips to The Same Position LeetCode Solution in C++
class Solution {
public:
int minCostToMoveChips(vector<int>& position) {
vector<int> count(2);
for (const int p : position)
++count[p % 2];
return min(count[0], count[1]);
}
};
/* code provided by PROGIEZ */
1217. Minimum Cost to Move Chips to The Same Position LeetCode Solution in Java
class Solution {
public int minCostToMoveChips(int[] position) {
int[] count = new int[2];
for (final int p : position)
++count[p % 2];
return Math.min(count[0], count[1]);
}
}
// code provided by PROGIEZ
1217. Minimum Cost to Move Chips to The Same Position LeetCode Solution in Python
class Solution:
def minCostToMoveChips(self, position: list[int]) -> int:
count = [0, 0]
for p in position:
count[p % 2] += 1
return min(count[0], count[1])
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.